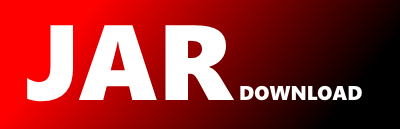
com.amazonaws.services.s3.model.CompleteMultipartUploadResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-java-sdk Show documentation
Show all versions of aws-java-sdk Show documentation
The Amazon Web Services SDK for Java provides Java APIs for building software on AWS' cost-effective, scalable, and reliable infrastructure products. The AWS Java SDK allows developers to code against APIs for all of Amazon's infrastructure web services (Amazon S3, Amazon EC2, Amazon SQS, Amazon Relational Database Service, Amazon AutoScaling, etc).
The newest version!
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.s3.model;
import java.util.Date;
import com.amazonaws.services.s3.internal.ObjectExpirationResult;
import com.amazonaws.services.s3.internal.ServerSideEncryptionResult;
/**
* The CompleteMultipartUploadResult contains all the information about the
* CompleteMultipartUpload method.
*/
public class CompleteMultipartUploadResult implements ServerSideEncryptionResult, ObjectExpirationResult {
/** The name of the bucket containing the completed multipart upload. */
private String bucketName;
/** The key by which the object is stored. */
private String key;
/** The URL identifying the new multipart object. */
private String location;
/**
* The entity tag identifying the new object. An entity tag is an opaque
* string that changes if and only if an object's data changes.
*/
private String eTag;
/**
* The version ID of the new object, only present if versioning has been
* enabled for the bucket.
*/
private String versionId;
/** The server side encryption algorithm of the new object */
private String serverSideEncryption;
/** The time this object expires, or null if it has no expiration */
private Date expirationTime;
/** The expiration rule for this object */
private String expirationTimeRuleId;
/**
* Returns the URL identifying the new multipart object.
*
* @return The URL identifying the new multipart object.
*/
public String getLocation() {
return location;
}
/**
* Sets the URL identifying the new multipart object.
*
* @param location
* The URL identifying the new multipart object.
*/
public void setLocation(String location) {
this.location = location;
}
/**
* Returns the name of the bucket containing the completed multipart object.
*
* @return The name of the bucket containing the completed multipart object.
*/
public String getBucketName() {
return bucketName;
}
/**
* Sets the name of the bucket containing the completed multipart object.
*
* @param bucketName
* The name of the bucket containing the completed multipart
* object.
*/
public void setBucketName(String bucketName) {
this.bucketName = bucketName;
}
/**
* Gets the key by which the newly created object is stored.
*/
public String getKey() {
return key;
}
/**
* Sets the key of the newly created object.
*/
public void setKey(String key) {
this.key = key;
}
/**
* Returns the entity tag identifying the new object. An entity tag is an
* opaque string that changes if and only if an object's data changes.
*
* @return An opaque string that changes if and only if an object's data
* changes.
*/
public String getETag() {
return eTag;
}
/**
* Sets the entity tag identifying the new object. An entity tag is an
* opaque string that changes if and only if an object's data changes.
*
* @param etag
* The entity tag.
*/
public void setETag(String etag) {
this.eTag = etag;
}
/**
* Returns the version ID of the new object, only present if versioning has
* been enabled for the bucket.
*
* @return The version ID of the new object, only present if versioning has
* been enabled for the bucket.
*/
public String getVersionId() {
return versionId;
}
/**
* Sets the version ID of the new object, only present if versioning has
* been enabled for the bucket.
*
* @param versionId
* The version ID of the new object, only present if versioning
* has been enabled for the bucket.
*/
public void setVersionId(String versionId) {
this.versionId = versionId;
}
/**
* Returns the server-side encryption algorithm for the newly created
* object, or null if none was used.
*/
public String getServerSideEncryption() {
return serverSideEncryption;
}
/**
* Sets the server-side encryption algorithm for the newly created object.
*
* @param serverSideEncryption
* The server-side encryption algorithm for the new object.
*/
public void setServerSideEncryption(String serverSideEncryption) {
this.serverSideEncryption = serverSideEncryption;
}
/**
* Returns the expiration time for this object, or null if it doesn't expire.
*/
public Date getExpirationTime() {
return expirationTime;
}
/**
* Sets the expiration time for the object.
*
* @param expirationTime
* The expiration time for the object.
*/
public void setExpirationTime(Date expirationTime) {
this.expirationTime = expirationTime;
}
/**
* Returns the {@link BucketLifecycleConfiguration} rule ID for this
* object's expiration, or null if it doesn't expire.
*/
public String getExpirationTimeRuleId() {
return expirationTimeRuleId;
}
/**
* Sets the {@link BucketLifecycleConfiguration} rule ID for this object's
* expiration
*
* @param expirationTimeRuleId
* The rule ID for this object's expiration
*/
public void setExpirationTimeRuleId(String expirationTimeRuleId) {
this.expirationTimeRuleId = expirationTimeRuleId;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy