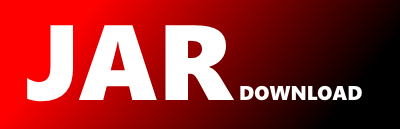
com.amazonaws.services.simpledb.AmazonSimpleDB Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.simpledb;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.simpledb.model.*;
/**
* Interface for accessing AmazonSimpleDB.
* Amazon SimpleDB
* Amazon SimpleDB is a web service providing the core database
* functions of data indexing and querying in the cloud. By offloading
* the time and effort associated with building and operating a web-scale
* database, SimpleDB provides developers the freedom to focus on
* application development.
*
*
* A traditional, clustered relational database requires a sizable
* upfront capital outlay, is complex to design, and often requires
* extensive and repetitive database administration. Amazon SimpleDB is
* dramatically simpler, requiring no schema, automatically indexing your
* data and providing a simple API for storage and access. This approach
* eliminates the administrative burden of data modeling, index
* maintenance, and performance tuning. Developers gain access to this
* functionality within Amazon's proven computing environment, are able
* to scale instantly, and pay only for what they use.
*
*
* Visit
* http://aws.amazon.com/simpledb/
* for more information.
*
*/
public interface AmazonSimpleDB {
/**
* Overrides the default endpoint for this client ("http://sdb.amazonaws.com").
* Callers can use this method to control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex: "sdb.amazonaws.com") or a full
* URL, including the protocol (ex: "http://sdb.amazonaws.com"). If the
* protocol is not specified here, the default protocol from this client's
* {@link ClientConfiguration} will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and
* a complete list of all available endpoints for all AWS services, see:
*
* http://developer.amazonwebservices.com/connect/entry.jspa?externalID=3912
*
* This method is not threadsafe. An endpoint should be configured when the
* client is created and before any service requests are made. Changing it
* afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param endpoint
* The endpoint (ex: "sdb.amazonaws.com") or a full URL,
* including the protocol (ex: "http://sdb.amazonaws.com") of
* the region specific AWS endpoint this client will communicate
* with.
*
* @throws IllegalArgumentException
* If any problems are detected with the specified endpoint.
*/
public void setEndpoint(String endpoint) throws java.lang.IllegalArgumentException;
/**
* An alternative to {@link AmazonSimpleDB#setEndpoint(String)}, sets the
* regional endpoint for this client's service calls. Callers can use this
* method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol.
* To use http instead, specify it in the {@link ClientConfiguration}
* supplied at construction.
*
* This method is not threadsafe. A region should be configured when the
* client is created and before any service requests are made. Changing it
* afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param region
* The region this client will communicate with. See
* {@link Region#getRegion(com.amazonaws.regions.Regions)} for
* accessing a given region.
* @throws java.lang.IllegalArgumentException
* If the given region is null, or if this service isn't
* available in the given region. See
* {@link Region#isServiceSupported(String)}
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class, com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
*/
public void setRegion(Region region) throws java.lang.IllegalArgumentException;
/**
*
* The Select
operation returns a set of attributes for
* ItemNames
that match the select expression.
* Select
is similar to the standard SQL SELECT statement.
*
*
* The total size of the response cannot exceed 1 MB in total size.
* Amazon SimpleDB automatically adjusts the number of items returned per
* page to enforce this limit. For example, if the client asks to
* retrieve 2500 items, but each individual item is 10 kB in size, the
* system returns 100 items and an appropriate NextToken
so
* the client can access the next page of results.
*
*
* For information on how to construct select expressions, see Using
* Select to Create Amazon SimpleDB Queries in the Developer Guide.
*
*
* @param selectRequest Container for the necessary parameters to execute
* the Select service method on AmazonSimpleDB.
*
* @return The response from the Select service method, as returned by
* AmazonSimpleDB.
*
* @throws InvalidParameterValueException
* @throws InvalidQueryExpressionException
* @throws RequestTimeoutException
* @throws InvalidNumberPredicatesException
* @throws NoSuchDomainException
* @throws InvalidNextTokenException
* @throws TooManyRequestedAttributesException
* @throws MissingParameterException
* @throws InvalidNumberValueTestsException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleDB indicating
* either a problem with the data in the request, or a server side issue.
*/
public SelectResult select(SelectRequest selectRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The PutAttributes operation creates or replaces attributes in an
* item. The client may specify new attributes using a combination of the
* Attribute.X.Name
and Attribute.X.Value
* parameters. The client specifies the first attribute by the parameters
* Attribute.0.Name
and Attribute.0.Value
,
* the second attribute by the parameters Attribute.1.Name
* and Attribute.1.Value
, and so on.
*
*
* Attributes are uniquely identified in an item by their name/value
* combination. For example, a single item can have the attributes
* { "first_name", "first_value" }
and { "first_name",
* second_value" }
. However, it cannot have two attribute
* instances where both the Attribute.X.Name
and
* Attribute.X.Value
are the same.
*
*
* Optionally, the requestor can supply the Replace
* parameter for each individual attribute. Setting this value to
* true
causes the new attribute value to replace the
* existing attribute value(s). For example, if an item has the
* attributes { 'a', '1' }
,
*
* { 'b', '2'}
and { 'b', '3'
* }
and the requestor calls PutAttributes
using the
* attributes { 'b', '4' }
with the Replace
* parameter set to true, the final attributes of the item are changed to
* { 'a', '1' }
and { 'b', '4' }
, which
* replaces the previous values of the 'b' attribute with the new value.
*
*
* NOTE: Using PutAttributes to replace attribute values that do
* not exist will not result in an error response.
*
*
* You cannot specify an empty string as an attribute name.
*
*
* Because Amazon SimpleDB makes multiple copies of client data and uses
* an eventual consistency update model, an immediate GetAttributes or
* Select operation (read) immediately after a PutAttributes or
* DeleteAttributes operation (write) might not return the updated data.
*
*
* The following limitations are enforced for this operation:
*
* - 256 total attribute name-value pairs per item
* - One billion attributes per domain
* - 10 GB of total user data storage per domain
*
*
*
*
*
* @param putAttributesRequest Container for the necessary parameters to
* execute the PutAttributes service method on AmazonSimpleDB.
*
*
* @throws InvalidParameterValueException
* @throws NumberDomainBytesExceededException
* @throws NumberDomainAttributesExceededException
* @throws NoSuchDomainException
* @throws NumberItemAttributesExceededException
* @throws AttributeDoesNotExistException
* @throws MissingParameterException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleDB indicating
* either a problem with the data in the request, or a server side issue.
*/
public void putAttributes(PutAttributesRequest putAttributesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Performs multiple DeleteAttributes operations in a single call, which
* reduces round trips and latencies. This enables Amazon SimpleDB to
* optimize requests, which generally yields better throughput.
*
*
* NOTE: If you specify BatchDeleteAttributes without attributes
* or values, all the attributes for the item are deleted.
* BatchDeleteAttributes is an idempotent operation; running it multiple
* times on the same item or attribute doesn't result in an error. The
* BatchDeleteAttributes operation succeeds or fails in its entirety.
* There are no partial deletes. You can execute multiple
* BatchDeleteAttributes operations and other operations in parallel.
* However, large numbers of concurrent BatchDeleteAttributes calls can
* result in Service Unavailable (503) responses. This operation is
* vulnerable to exceeding the maximum URL size when making a REST
* request using the HTTP GET method. This operation does not support
* conditions using Expected.X.Name, Expected.X.Value, or
* Expected.X.Exists.
*
*
* The following limitations are enforced for this operation:
*
* - 1 MB request size
* - 25 item limit per BatchDeleteAttributes operation
*
*
*
*
*
* @param batchDeleteAttributesRequest Container for the necessary
* parameters to execute the BatchDeleteAttributes service method on
* AmazonSimpleDB.
*
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleDB indicating
* either a problem with the data in the request, or a server side issue.
*/
public void batchDeleteAttributes(BatchDeleteAttributesRequest batchDeleteAttributesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The DeleteDomain
operation deletes a domain. Any items
* (and their attributes) in the domain are deleted as well. The
* DeleteDomain
operation might take 10 or more seconds to
* complete.
*
*
* NOTE: Running DeleteDomain on a domain that does not exist or
* running the function multiple times using the same domain name will
* not result in an error response.
*
*
* @param deleteDomainRequest Container for the necessary parameters to
* execute the DeleteDomain service method on AmazonSimpleDB.
*
*
* @throws MissingParameterException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleDB indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteDomain(DeleteDomainRequest deleteDomainRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The CreateDomain
operation creates a new domain. The
* domain name should be unique among the domains associated with the
* Access Key ID provided in the request. The CreateDomain
* operation may take 10 or more seconds to complete.
*
*
* NOTE: CreateDomain is an idempotent operation; running it
* multiple times using the same domain name will not result in an error
* response.
*
*
* The client can create up to 100 domains per account.
*
*
* If the client requires additional domains, go to
* http://aws.amazon.com/contact-us/simpledb-limit-request/
* .
*
*
* @param createDomainRequest Container for the necessary parameters to
* execute the CreateDomain service method on AmazonSimpleDB.
*
*
* @throws InvalidParameterValueException
* @throws NumberDomainsExceededException
* @throws MissingParameterException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleDB indicating
* either a problem with the data in the request, or a server side issue.
*/
public void createDomain(CreateDomainRequest createDomainRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deletes one or more attributes associated with an item. If all
* attributes of the item are deleted, the item is deleted.
*
*
* NOTE: If DeleteAttributes is called without being passed any
* attributes or values specified, all the attributes for the item are
* deleted.
*
*
* DeleteAttributes
is an idempotent operation; running it
* multiple times on the same item or attribute does not result in an
* error response.
*
*
* Because Amazon SimpleDB makes multiple copies of item data and uses
* an eventual consistency update model, performing a GetAttributes or
* Select operation (read) immediately after a
* DeleteAttributes
or PutAttributes operation (write) might
* not return updated item data.
*
*
* @param deleteAttributesRequest Container for the necessary parameters
* to execute the DeleteAttributes service method on AmazonSimpleDB.
*
*
* @throws InvalidParameterValueException
* @throws NoSuchDomainException
* @throws AttributeDoesNotExistException
* @throws MissingParameterException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleDB indicating
* either a problem with the data in the request, or a server side issue.
*/
public void deleteAttributes(DeleteAttributesRequest deleteAttributesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ListDomains
operation lists all domains associated
* with the Access Key ID. It returns domain names up to the limit set by
* MaxNumberOfDomains. A NextToken is returned if there are more than
* MaxNumberOfDomains
domains. Calling
* ListDomains
successive times with the
* NextToken
provided by the operation returns up to
* MaxNumberOfDomains
more domain names with each successive
* operation call.
*
*
* @param listDomainsRequest Container for the necessary parameters to
* execute the ListDomains service method on AmazonSimpleDB.
*
* @return The response from the ListDomains service method, as returned
* by AmazonSimpleDB.
*
* @throws InvalidParameterValueException
* @throws InvalidNextTokenException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleDB indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListDomainsResult listDomains(ListDomainsRequest listDomainsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns all of the attributes associated with the specified item.
* Optionally, the attributes returned can be limited to one or more
* attributes by specifying an attribute name parameter.
*
*
* If the item does not exist on the replica that was accessed for this
* operation, an empty set is returned. The system does not return an
* error as it cannot guarantee the item does not exist on other
* replicas.
*
*
* NOTE: If GetAttributes is called without being passed any
* attribute names, all the attributes for the item are returned.
*
*
* @param getAttributesRequest Container for the necessary parameters to
* execute the GetAttributes service method on AmazonSimpleDB.
*
* @return The response from the GetAttributes service method, as
* returned by AmazonSimpleDB.
*
* @throws InvalidParameterValueException
* @throws NoSuchDomainException
* @throws MissingParameterException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleDB indicating
* either a problem with the data in the request, or a server side issue.
*/
public GetAttributesResult getAttributes(GetAttributesRequest getAttributesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The BatchPutAttributes
operation creates or replaces
* attributes within one or more items. By using this operation, the
* client can perform multiple PutAttribute operation with a single call.
* This helps yield savings in round trips and latencies, enabling
* Amazon SimpleDB to optimize requests and generally produce better
* throughput.
*
*
* The client may specify the item name with the
* Item.X.ItemName
parameter. The client may specify new
* attributes using a combination of the
* Item.X.Attribute.Y.Name
and
* Item.X.Attribute.Y.Value
parameters. The client may
* specify the first attribute for the first item using the parameters
* Item.0.Attribute.0.Name
and
* Item.0.Attribute.0.Value
,
* and for the second attribute for the first item by the parameters
* Item.0.Attribute.1.Name
and
* Item.0.Attribute.1.Value
,
* and so on.
*
*
* Attributes are uniquely identified within an item by their name/value
* combination. For example, a single item can have the attributes
* { "first_name", "first_value" }
and { "first_name",
* "second_value" }
.
* However, it cannot have two attribute instances where both the
* Item.X.Attribute.Y.Name
and
* Item.X.Attribute.Y.Value
are the same.
*
*
* Optionally, the requester can supply the Replace
* parameter for each individual value. Setting this value to
* true
will cause the new attribute values to replace the
* existing attribute values. For example, if an item I
has
* the attributes { 'a', '1' }, { 'b', '2'}
and { 'b',
* '3' }
and the requester does a BatchPutAttributes of
* {'I', 'b', '4' }
with the Replace parameter set to true,
* the final attributes of the item will be { 'a', '1' }
and
* { 'b', '4' }
,
* replacing the previous values of the 'b' attribute with the new
* value.
*
*
* NOTE: You cannot specify an empty string as an item or as an
* attribute name. The BatchPutAttributes operation succeeds or fails in
* its entirety. There are no partial puts.
*
*
* IMPORTANT: This operation is vulnerable to exceeding the
* maximum URL size when making a REST request using the HTTP GET method.
* This operation does not support conditions using Expected.X.Name,
* Expected.X.Value, or Expected.X.Exists.
*
*
* You can execute multiple BatchPutAttributes
operations
* and other operations in parallel. However, large numbers of concurrent
* BatchPutAttributes
calls can result in Service
* Unavailable (503) responses.
*
*
* The following limitations are enforced for this operation:
*
* - 256 attribute name-value pairs per item
* - 1 MB request size
* - 1 billion attributes per domain
* - 10 GB of total user data storage per domain
* - 25 item limit per
BatchPutAttributes
operation
*
*
*
*
*
* @param batchPutAttributesRequest Container for the necessary
* parameters to execute the BatchPutAttributes service method on
* AmazonSimpleDB.
*
*
* @throws DuplicateItemNameException
* @throws InvalidParameterValueException
* @throws NumberDomainBytesExceededException
* @throws NumberSubmittedItemsExceededException
* @throws NumberSubmittedAttributesExceededException
* @throws NumberDomainAttributesExceededException
* @throws NoSuchDomainException
* @throws NumberItemAttributesExceededException
* @throws MissingParameterException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleDB indicating
* either a problem with the data in the request, or a server side issue.
*/
public void batchPutAttributes(BatchPutAttributesRequest batchPutAttributesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about the domain, including when the domain was
* created, the number of items and attributes in the domain, and the
* size of the attribute names and values.
*
*
* @param domainMetadataRequest Container for the necessary parameters to
* execute the DomainMetadata service method on AmazonSimpleDB.
*
* @return The response from the DomainMetadata service method, as
* returned by AmazonSimpleDB.
*
* @throws NoSuchDomainException
* @throws MissingParameterException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleDB indicating
* either a problem with the data in the request, or a server side issue.
*/
public DomainMetadataResult domainMetadata(DomainMetadataRequest domainMetadataRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* The ListDomains
operation lists all domains associated
* with the Access Key ID. It returns domain names up to the limit set by
* MaxNumberOfDomains. A NextToken is returned if there are more than
* MaxNumberOfDomains
domains. Calling
* ListDomains
successive times with the
* NextToken
provided by the operation returns up to
* MaxNumberOfDomains
more domain names with each successive
* operation call.
*
*
* @return The response from the ListDomains service method, as returned
* by AmazonSimpleDB.
*
* @throws InvalidParameterValueException
* @throws InvalidNextTokenException
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleDB indicating
* either a problem with the data in the request, or a server side issue.
*/
public ListDomainsResult listDomains() throws AmazonServiceException, AmazonClientException;
}