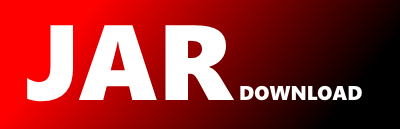
com.amazonaws.services.simpleemail.AmazonSimpleEmailServiceAsyncClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.simpleemail;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
import com.amazonaws.AmazonClientException;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.handlers.AsyncHandler;
import com.amazonaws.ClientConfiguration;
import com.amazonaws.auth.AWSCredentials;
import com.amazonaws.auth.AWSCredentialsProvider;
import com.amazonaws.auth.DefaultAWSCredentialsProviderChain;
import com.amazonaws.services.simpleemail.model.*;
/**
* Asynchronous client for accessing AmazonSimpleEmailService.
* All asynchronous calls made using this client are non-blocking. Callers could either
* process the result and handle the exceptions in the worker thread by providing a callback handler
* when making the call, or use the returned Future object to check the result of the call in the calling thread.
* Amazon Simple Email Service
* This is the API Reference for Amazon Simple Email Service (Amazon
* SES). This documentation is intended to be used in conjunction with
* the
* Amazon SES Developer Guide
* .
*
*
* NOTE:For a list of Amazon SES endpoints to use in service
* requests, see Regions and Amazon SES in the Amazon SES Developer
* Guide.
*
*/
public class AmazonSimpleEmailServiceAsyncClient extends AmazonSimpleEmailServiceClient
implements AmazonSimpleEmailServiceAsync {
/**
* Executor service for executing asynchronous requests.
*/
private ExecutorService executorService;
private static final int DEFAULT_THREAD_POOL_SIZE = 50;
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonSimpleEmailService. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @see DefaultAWSCredentialsProviderChain
*/
public AmazonSimpleEmailServiceAsyncClient() {
this(new DefaultAWSCredentialsProviderChain());
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonSimpleEmailService. A credentials provider chain will be used
* that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not
* return until the service call completes.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to AmazonSimpleEmailService
* (ex: proxy settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
*/
public AmazonSimpleEmailServiceAsyncClient(ClientConfiguration clientConfiguration) {
this(new DefaultAWSCredentialsProviderChain(), clientConfiguration, Executors.newFixedThreadPool(clientConfiguration.getMaxConnections()));
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonSimpleEmailService using the specified AWS account credentials.
* Default client settings will be used, and a fixed size thread pool will be
* created for executing the asynchronous tasks.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentials The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
*/
public AmazonSimpleEmailServiceAsyncClient(AWSCredentials awsCredentials) {
this(awsCredentials, Executors.newFixedThreadPool(DEFAULT_THREAD_POOL_SIZE));
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonSimpleEmailService using the specified AWS account credentials
* and executor service. Default client settings will be used.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
* @param executorService
* The executor service by which all asynchronous requests will
* be executed.
*/
public AmazonSimpleEmailServiceAsyncClient(AWSCredentials awsCredentials, ExecutorService executorService) {
super(awsCredentials);
this.executorService = executorService;
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonSimpleEmailService using the specified AWS account credentials,
* executor service, and client configuration options.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use
* when authenticating with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy
* settings, etc).
* @param executorService
* The executor service by which all asynchronous requests will
* be executed.
*/
public AmazonSimpleEmailServiceAsyncClient(AWSCredentials awsCredentials,
ClientConfiguration clientConfiguration, ExecutorService executorService) {
super(awsCredentials, clientConfiguration);
this.executorService = executorService;
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonSimpleEmailService using the specified AWS account credentials provider.
* Default client settings will be used, and a fixed size thread pool will be
* created for executing the asynchronous tasks.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
*/
public AmazonSimpleEmailServiceAsyncClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, Executors.newFixedThreadPool(DEFAULT_THREAD_POOL_SIZE));
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonSimpleEmailService using the specified AWS account credentials provider
* and executor service. Default client settings will be used.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param executorService
* The executor service by which all asynchronous requests will
* be executed.
*/
public AmazonSimpleEmailServiceAsyncClient(AWSCredentialsProvider awsCredentialsProvider, ExecutorService executorService) {
this(awsCredentialsProvider, new ClientConfiguration(), executorService);
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonSimpleEmailService using the specified AWS account credentials
* provider and client configuration options.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy
* settings, etc).
*/
public AmazonSimpleEmailServiceAsyncClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, Executors.newFixedThreadPool(clientConfiguration.getMaxConnections()));
}
/**
* Constructs a new asynchronous client to invoke service methods on
* AmazonSimpleEmailService using the specified AWS account credentials
* provider, executor service, and client configuration options.
*
*
* All calls made using this new client object are non-blocking, and will immediately
* return a Java Future object that the caller can later check to see if the service
* call has actually completed.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials
* to authenticate requests with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy
* settings, etc).
* @param executorService
* The executor service by which all asynchronous requests will
* be executed.
*/
public AmazonSimpleEmailServiceAsyncClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration, ExecutorService executorService) {
super(awsCredentialsProvider, clientConfiguration);
this.executorService = executorService;
}
/**
* Returns the executor service used by this async client to execute
* requests.
*
* @return The executor service used by this async client to execute
* requests.
*/
public ExecutorService getExecutorService() {
return executorService;
}
/**
* Shuts down the client, releasing all managed resources. This includes
* forcibly terminating all pending asynchronous service calls. Clients who
* wish to give pending asynchronous service calls time to complete should
* call getExecutorService().shutdown() followed by
* getExecutorService().awaitTermination() prior to calling this method.
*/
@Override
public void shutdown() {
super.shutdown();
executorService.shutdownNow();
}
/**
*
* Deletes the specified identity (email address or domain) from the list
* of verified identities.
*
*
* This action is throttled at one request per second.
*
*
* @param deleteIdentityRequest Container for the necessary parameters to
* execute the DeleteIdentity operation on AmazonSimpleEmailService.
*
* @return A Java Future object containing the response from the
* DeleteIdentity service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteIdentityAsync(final DeleteIdentityRequest deleteIdentityRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DeleteIdentityResult call() throws Exception {
return deleteIdentity(deleteIdentityRequest);
}
});
}
/**
*
* Deletes the specified identity (email address or domain) from the list
* of verified identities.
*
*
* This action is throttled at one request per second.
*
*
* @param deleteIdentityRequest Container for the necessary parameters to
* execute the DeleteIdentity operation on AmazonSimpleEmailService.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteIdentity service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteIdentityAsync(
final DeleteIdentityRequest deleteIdentityRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public DeleteIdentityResult call() throws Exception {
DeleteIdentityResult result;
try {
result = deleteIdentity(deleteIdentityRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(deleteIdentityRequest, result);
return result;
}
});
}
/**
*
* Returns a list containing all of the email addresses that have been
* verified.
*
*
* IMPORTANT:The ListVerifiedEmailAddresses action is deprecated
* as of the May 15, 2012 release of Domain Verification. The
* ListIdentities action is now preferred.
*
*
* This action is throttled at one request per second.
*
*
* @param listVerifiedEmailAddressesRequest Container for the necessary
* parameters to execute the ListVerifiedEmailAddresses operation on
* AmazonSimpleEmailService.
*
* @return A Java Future object containing the response from the
* ListVerifiedEmailAddresses service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listVerifiedEmailAddressesAsync(final ListVerifiedEmailAddressesRequest listVerifiedEmailAddressesRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ListVerifiedEmailAddressesResult call() throws Exception {
return listVerifiedEmailAddresses(listVerifiedEmailAddressesRequest);
}
});
}
/**
*
* Returns a list containing all of the email addresses that have been
* verified.
*
*
* IMPORTANT:The ListVerifiedEmailAddresses action is deprecated
* as of the May 15, 2012 release of Domain Verification. The
* ListIdentities action is now preferred.
*
*
* This action is throttled at one request per second.
*
*
* @param listVerifiedEmailAddressesRequest Container for the necessary
* parameters to execute the ListVerifiedEmailAddresses operation on
* AmazonSimpleEmailService.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListVerifiedEmailAddresses service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listVerifiedEmailAddressesAsync(
final ListVerifiedEmailAddressesRequest listVerifiedEmailAddressesRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ListVerifiedEmailAddressesResult call() throws Exception {
ListVerifiedEmailAddressesResult result;
try {
result = listVerifiedEmailAddresses(listVerifiedEmailAddressesRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(listVerifiedEmailAddressesRequest, result);
return result;
}
});
}
/**
*
* Returns the user's sending statistics. The result is a list of data
* points, representing the last two weeks of sending activity.
*
*
* Each data point in the list contains statistics for a 15-minute
* interval.
*
*
* This action is throttled at one request per second.
*
*
* @param getSendStatisticsRequest Container for the necessary parameters
* to execute the GetSendStatistics operation on
* AmazonSimpleEmailService.
*
* @return A Java Future object containing the response from the
* GetSendStatistics service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getSendStatisticsAsync(final GetSendStatisticsRequest getSendStatisticsRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetSendStatisticsResult call() throws Exception {
return getSendStatistics(getSendStatisticsRequest);
}
});
}
/**
*
* Returns the user's sending statistics. The result is a list of data
* points, representing the last two weeks of sending activity.
*
*
* Each data point in the list contains statistics for a 15-minute
* interval.
*
*
* This action is throttled at one request per second.
*
*
* @param getSendStatisticsRequest Container for the necessary parameters
* to execute the GetSendStatistics operation on
* AmazonSimpleEmailService.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* GetSendStatistics service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getSendStatisticsAsync(
final GetSendStatisticsRequest getSendStatisticsRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetSendStatisticsResult call() throws Exception {
GetSendStatisticsResult result;
try {
result = getSendStatistics(getSendStatisticsRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(getSendStatisticsRequest, result);
return result;
}
});
}
/**
*
* Verifies an email address. This action causes a confirmation email
* message to be sent to the specified address.
*
*
* This action is throttled at one request per second.
*
*
* @param verifyEmailIdentityRequest Container for the necessary
* parameters to execute the VerifyEmailIdentity operation on
* AmazonSimpleEmailService.
*
* @return A Java Future object containing the response from the
* VerifyEmailIdentity service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future verifyEmailIdentityAsync(final VerifyEmailIdentityRequest verifyEmailIdentityRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public VerifyEmailIdentityResult call() throws Exception {
return verifyEmailIdentity(verifyEmailIdentityRequest);
}
});
}
/**
*
* Verifies an email address. This action causes a confirmation email
* message to be sent to the specified address.
*
*
* This action is throttled at one request per second.
*
*
* @param verifyEmailIdentityRequest Container for the necessary
* parameters to execute the VerifyEmailIdentity operation on
* AmazonSimpleEmailService.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* VerifyEmailIdentity service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future verifyEmailIdentityAsync(
final VerifyEmailIdentityRequest verifyEmailIdentityRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public VerifyEmailIdentityResult call() throws Exception {
VerifyEmailIdentityResult result;
try {
result = verifyEmailIdentity(verifyEmailIdentityRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(verifyEmailIdentityRequest, result);
return result;
}
});
}
/**
*
* Given a list of verified identities (email addresses and/or domains),
* returns a structure describing identity notification attributes.
*
*
* This action is throttled at one request per second.
*
*
* For more information about feedback notification, see the
* Amazon SES Developer Guide
* .
*
*
* @param getIdentityNotificationAttributesRequest Container for the
* necessary parameters to execute the GetIdentityNotificationAttributes
* operation on AmazonSimpleEmailService.
*
* @return A Java Future object containing the response from the
* GetIdentityNotificationAttributes service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getIdentityNotificationAttributesAsync(final GetIdentityNotificationAttributesRequest getIdentityNotificationAttributesRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetIdentityNotificationAttributesResult call() throws Exception {
return getIdentityNotificationAttributes(getIdentityNotificationAttributesRequest);
}
});
}
/**
*
* Given a list of verified identities (email addresses and/or domains),
* returns a structure describing identity notification attributes.
*
*
* This action is throttled at one request per second.
*
*
* For more information about feedback notification, see the
* Amazon SES Developer Guide
* .
*
*
* @param getIdentityNotificationAttributesRequest Container for the
* necessary parameters to execute the GetIdentityNotificationAttributes
* operation on AmazonSimpleEmailService.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* GetIdentityNotificationAttributes service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getIdentityNotificationAttributesAsync(
final GetIdentityNotificationAttributesRequest getIdentityNotificationAttributesRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetIdentityNotificationAttributesResult call() throws Exception {
GetIdentityNotificationAttributesResult result;
try {
result = getIdentityNotificationAttributes(getIdentityNotificationAttributesRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(getIdentityNotificationAttributesRequest, result);
return result;
}
});
}
/**
*
* Returns a set of DKIM tokens for a domain. DKIM tokens are
* character strings that represent your domain's identity. Using these
* tokens, you will need to create DNS CNAME records that point to DKIM
* public keys hosted by Amazon SES. Amazon Web Services will eventually
* detect that you have updated your DNS records; this detection process
* may take up to 72 hours. Upon successful detection, Amazon SES will be
* able to DKIM-sign email originating from that domain.
*
*
* This action is throttled at one request per second.
*
*
* To enable or disable Easy DKIM signing for a domain, use the
* SetIdentityDkimEnabled
action.
*
*
* For more information about creating DNS records using DKIM tokens, go
* to the
* Amazon SES Developer Guide
* .
*
*
* @param verifyDomainDkimRequest Container for the necessary parameters
* to execute the VerifyDomainDkim operation on AmazonSimpleEmailService.
*
* @return A Java Future object containing the response from the
* VerifyDomainDkim service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future verifyDomainDkimAsync(final VerifyDomainDkimRequest verifyDomainDkimRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public VerifyDomainDkimResult call() throws Exception {
return verifyDomainDkim(verifyDomainDkimRequest);
}
});
}
/**
*
* Returns a set of DKIM tokens for a domain. DKIM tokens are
* character strings that represent your domain's identity. Using these
* tokens, you will need to create DNS CNAME records that point to DKIM
* public keys hosted by Amazon SES. Amazon Web Services will eventually
* detect that you have updated your DNS records; this detection process
* may take up to 72 hours. Upon successful detection, Amazon SES will be
* able to DKIM-sign email originating from that domain.
*
*
* This action is throttled at one request per second.
*
*
* To enable or disable Easy DKIM signing for a domain, use the
* SetIdentityDkimEnabled
action.
*
*
* For more information about creating DNS records using DKIM tokens, go
* to the
* Amazon SES Developer Guide
* .
*
*
* @param verifyDomainDkimRequest Container for the necessary parameters
* to execute the VerifyDomainDkim operation on AmazonSimpleEmailService.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* VerifyDomainDkim service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future verifyDomainDkimAsync(
final VerifyDomainDkimRequest verifyDomainDkimRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public VerifyDomainDkimResult call() throws Exception {
VerifyDomainDkimResult result;
try {
result = verifyDomainDkim(verifyDomainDkimRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(verifyDomainDkimRequest, result);
return result;
}
});
}
/**
*
* Returns the current status of Easy DKIM signing for an entity. For
* domain name identities, this action also returns the DKIM tokens that
* are required for Easy DKIM signing, and whether Amazon SES has
* successfully verified that these tokens have been published.
*
*
* This action takes a list of identities as input and returns the
* following information for each:
*
*
*
* - Whether Easy DKIM signing is enabled or disabled.
* - A set of DKIM tokens that represent the identity. If the identity
* is an email address, the tokens represent the domain of that
* address.
* - Whether Amazon SES has successfully verified the DKIM tokens
* published in the domain's DNS. This information is only returned for
* domain name identities, not for email addresses.
*
*
*
* This action is throttled at one request per second.
*
*
* For more information about creating DNS records using DKIM tokens, go
* to the
* Amazon SES Developer Guide
* .
*
*
* @param getIdentityDkimAttributesRequest Container for the necessary
* parameters to execute the GetIdentityDkimAttributes operation on
* AmazonSimpleEmailService.
*
* @return A Java Future object containing the response from the
* GetIdentityDkimAttributes service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getIdentityDkimAttributesAsync(final GetIdentityDkimAttributesRequest getIdentityDkimAttributesRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetIdentityDkimAttributesResult call() throws Exception {
return getIdentityDkimAttributes(getIdentityDkimAttributesRequest);
}
});
}
/**
*
* Returns the current status of Easy DKIM signing for an entity. For
* domain name identities, this action also returns the DKIM tokens that
* are required for Easy DKIM signing, and whether Amazon SES has
* successfully verified that these tokens have been published.
*
*
* This action takes a list of identities as input and returns the
* following information for each:
*
*
*
* - Whether Easy DKIM signing is enabled or disabled.
* - A set of DKIM tokens that represent the identity. If the identity
* is an email address, the tokens represent the domain of that
* address.
* - Whether Amazon SES has successfully verified the DKIM tokens
* published in the domain's DNS. This information is only returned for
* domain name identities, not for email addresses.
*
*
*
* This action is throttled at one request per second.
*
*
* For more information about creating DNS records using DKIM tokens, go
* to the
* Amazon SES Developer Guide
* .
*
*
* @param getIdentityDkimAttributesRequest Container for the necessary
* parameters to execute the GetIdentityDkimAttributes operation on
* AmazonSimpleEmailService.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* GetIdentityDkimAttributes service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getIdentityDkimAttributesAsync(
final GetIdentityDkimAttributesRequest getIdentityDkimAttributesRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetIdentityDkimAttributesResult call() throws Exception {
GetIdentityDkimAttributesResult result;
try {
result = getIdentityDkimAttributes(getIdentityDkimAttributesRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(getIdentityDkimAttributesRequest, result);
return result;
}
});
}
/**
*
* Verifies an email address. This action causes a confirmation email
* message to be sent to the specified address.
*
*
* IMPORTANT:The VerifyEmailAddress action is deprecated as of the
* May 15, 2012 release of Domain Verification. The VerifyEmailIdentity
* action is now preferred.
*
*
* This action is throttled at one request per second.
*
*
* @param verifyEmailAddressRequest Container for the necessary
* parameters to execute the VerifyEmailAddress operation on
* AmazonSimpleEmailService.
*
* @return A Java Future object containing the response from the
* VerifyEmailAddress service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future verifyEmailAddressAsync(final VerifyEmailAddressRequest verifyEmailAddressRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
verifyEmailAddress(verifyEmailAddressRequest);
return null;
}
});
}
/**
*
* Verifies an email address. This action causes a confirmation email
* message to be sent to the specified address.
*
*
* IMPORTANT:The VerifyEmailAddress action is deprecated as of the
* May 15, 2012 release of Domain Verification. The VerifyEmailIdentity
* action is now preferred.
*
*
* This action is throttled at one request per second.
*
*
* @param verifyEmailAddressRequest Container for the necessary
* parameters to execute the VerifyEmailAddress operation on
* AmazonSimpleEmailService.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* VerifyEmailAddress service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future verifyEmailAddressAsync(
final VerifyEmailAddressRequest verifyEmailAddressRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
verifyEmailAddress(verifyEmailAddressRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(verifyEmailAddressRequest, null);
return null;
}
});
}
/**
*
* Sends an email message, with header and content specified by the
* client. The SendRawEmail
action is useful for sending
* multipart MIME emails. The raw text of the message must comply with
* Internet email standards; otherwise, the message cannot be sent.
*
*
* IMPORTANT: You can only send email from verified email
* addresses and domains. If you have not requested production access to
* Amazon SES, you must also verify every recipient email address except
* for the recipients provided by the Amazon SES mailbox simulator. For
* more information, go to the Amazon SES Developer Guide.
*
*
* The total size of the message cannot exceed 10 MB. This includes any
* attachments that are part of the message.
*
*
* Amazon SES has a limit on the total number of recipients per message:
* The combined number of To:, CC: and BCC: email addresses cannot exceed
* 50. If you need to send an email message to a larger audience, you can
* divide your recipient list into groups of 50 or fewer, and then call
* Amazon SES repeatedly to send the message to each group.
*
*
* For every message that you send, the total number of recipients (To:,
* CC: and BCC:) is counted against your sending quota - the
* maximum number of emails you can send in a 24-hour period. For
* information about your sending quota, go to the
* Amazon SES Developer Guide
* .
*
*
* @param sendRawEmailRequest Container for the necessary parameters to
* execute the SendRawEmail operation on AmazonSimpleEmailService.
*
* @return A Java Future object containing the response from the
* SendRawEmail service method, as returned by AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future sendRawEmailAsync(final SendRawEmailRequest sendRawEmailRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public SendRawEmailResult call() throws Exception {
return sendRawEmail(sendRawEmailRequest);
}
});
}
/**
*
* Sends an email message, with header and content specified by the
* client. The SendRawEmail
action is useful for sending
* multipart MIME emails. The raw text of the message must comply with
* Internet email standards; otherwise, the message cannot be sent.
*
*
* IMPORTANT: You can only send email from verified email
* addresses and domains. If you have not requested production access to
* Amazon SES, you must also verify every recipient email address except
* for the recipients provided by the Amazon SES mailbox simulator. For
* more information, go to the Amazon SES Developer Guide.
*
*
* The total size of the message cannot exceed 10 MB. This includes any
* attachments that are part of the message.
*
*
* Amazon SES has a limit on the total number of recipients per message:
* The combined number of To:, CC: and BCC: email addresses cannot exceed
* 50. If you need to send an email message to a larger audience, you can
* divide your recipient list into groups of 50 or fewer, and then call
* Amazon SES repeatedly to send the message to each group.
*
*
* For every message that you send, the total number of recipients (To:,
* CC: and BCC:) is counted against your sending quota - the
* maximum number of emails you can send in a 24-hour period. For
* information about your sending quota, go to the
* Amazon SES Developer Guide
* .
*
*
* @param sendRawEmailRequest Container for the necessary parameters to
* execute the SendRawEmail operation on AmazonSimpleEmailService.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* SendRawEmail service method, as returned by AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future sendRawEmailAsync(
final SendRawEmailRequest sendRawEmailRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public SendRawEmailResult call() throws Exception {
SendRawEmailResult result;
try {
result = sendRawEmail(sendRawEmailRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(sendRawEmailRequest, result);
return result;
}
});
}
/**
*
* Returns a list containing all of the identities (email addresses and
* domains) for a specific AWS Account, regardless of verification
* status.
*
*
* This action is throttled at one request per second.
*
*
* @param listIdentitiesRequest Container for the necessary parameters to
* execute the ListIdentities operation on AmazonSimpleEmailService.
*
* @return A Java Future object containing the response from the
* ListIdentities service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listIdentitiesAsync(final ListIdentitiesRequest listIdentitiesRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ListIdentitiesResult call() throws Exception {
return listIdentities(listIdentitiesRequest);
}
});
}
/**
*
* Returns a list containing all of the identities (email addresses and
* domains) for a specific AWS Account, regardless of verification
* status.
*
*
* This action is throttled at one request per second.
*
*
* @param listIdentitiesRequest Container for the necessary parameters to
* execute the ListIdentities operation on AmazonSimpleEmailService.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListIdentities service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listIdentitiesAsync(
final ListIdentitiesRequest listIdentitiesRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public ListIdentitiesResult call() throws Exception {
ListIdentitiesResult result;
try {
result = listIdentities(listIdentitiesRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(listIdentitiesRequest, result);
return result;
}
});
}
/**
*
* Given a list of identities (email addresses and/or domains), returns
* the verification status and (for domain identities) the verification
* token for each identity.
*
*
* This action is throttled at one request per second.
*
*
* @param getIdentityVerificationAttributesRequest Container for the
* necessary parameters to execute the GetIdentityVerificationAttributes
* operation on AmazonSimpleEmailService.
*
* @return A Java Future object containing the response from the
* GetIdentityVerificationAttributes service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getIdentityVerificationAttributesAsync(final GetIdentityVerificationAttributesRequest getIdentityVerificationAttributesRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetIdentityVerificationAttributesResult call() throws Exception {
return getIdentityVerificationAttributes(getIdentityVerificationAttributesRequest);
}
});
}
/**
*
* Given a list of identities (email addresses and/or domains), returns
* the verification status and (for domain identities) the verification
* token for each identity.
*
*
* This action is throttled at one request per second.
*
*
* @param getIdentityVerificationAttributesRequest Container for the
* necessary parameters to execute the GetIdentityVerificationAttributes
* operation on AmazonSimpleEmailService.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* GetIdentityVerificationAttributes service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getIdentityVerificationAttributesAsync(
final GetIdentityVerificationAttributesRequest getIdentityVerificationAttributesRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetIdentityVerificationAttributesResult call() throws Exception {
GetIdentityVerificationAttributesResult result;
try {
result = getIdentityVerificationAttributes(getIdentityVerificationAttributesRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(getIdentityVerificationAttributesRequest, result);
return result;
}
});
}
/**
*
* Enables or disables Easy DKIM signing of email sent from an identity:
*
*
*
* - If Easy DKIM signing is enabled for a domain name identity (e.g.,
*
example.com
), then Amazon SES will DKIM-sign all email
* sent by addresses under that domain name (e.g.,
* [email protected]
).
* - If Easy DKIM signing is enabled for an email address, then Amazon
* SES will DKIM-sign all email sent by that email address.
*
*
*
* For email addresses (e.g., [email protected]
), you can
* only enable Easy DKIM signing if the corresponding domain (e.g.,
* example.com
) has been set up for Easy DKIM using the AWS
* Console or the VerifyDomainDkim
action.
*
*
* This action is throttled at one request per second.
*
*
* For more information about Easy DKIM signing, go to the
* Amazon SES Developer Guide
* .
*
*
* @param setIdentityDkimEnabledRequest Container for the necessary
* parameters to execute the SetIdentityDkimEnabled operation on
* AmazonSimpleEmailService.
*
* @return A Java Future object containing the response from the
* SetIdentityDkimEnabled service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future setIdentityDkimEnabledAsync(final SetIdentityDkimEnabledRequest setIdentityDkimEnabledRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public SetIdentityDkimEnabledResult call() throws Exception {
return setIdentityDkimEnabled(setIdentityDkimEnabledRequest);
}
});
}
/**
*
* Enables or disables Easy DKIM signing of email sent from an identity:
*
*
*
* - If Easy DKIM signing is enabled for a domain name identity (e.g.,
*
example.com
), then Amazon SES will DKIM-sign all email
* sent by addresses under that domain name (e.g.,
* [email protected]
).
* - If Easy DKIM signing is enabled for an email address, then Amazon
* SES will DKIM-sign all email sent by that email address.
*
*
*
* For email addresses (e.g., [email protected]
), you can
* only enable Easy DKIM signing if the corresponding domain (e.g.,
* example.com
) has been set up for Easy DKIM using the AWS
* Console or the VerifyDomainDkim
action.
*
*
* This action is throttled at one request per second.
*
*
* For more information about Easy DKIM signing, go to the
* Amazon SES Developer Guide
* .
*
*
* @param setIdentityDkimEnabledRequest Container for the necessary
* parameters to execute the SetIdentityDkimEnabled operation on
* AmazonSimpleEmailService.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* SetIdentityDkimEnabled service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future setIdentityDkimEnabledAsync(
final SetIdentityDkimEnabledRequest setIdentityDkimEnabledRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public SetIdentityDkimEnabledResult call() throws Exception {
SetIdentityDkimEnabledResult result;
try {
result = setIdentityDkimEnabled(setIdentityDkimEnabledRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(setIdentityDkimEnabledRequest, result);
return result;
}
});
}
/**
*
* Returns the user's current sending limits.
*
*
* This action is throttled at one request per second.
*
*
* @param getSendQuotaRequest Container for the necessary parameters to
* execute the GetSendQuota operation on AmazonSimpleEmailService.
*
* @return A Java Future object containing the response from the
* GetSendQuota service method, as returned by AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getSendQuotaAsync(final GetSendQuotaRequest getSendQuotaRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetSendQuotaResult call() throws Exception {
return getSendQuota(getSendQuotaRequest);
}
});
}
/**
*
* Returns the user's current sending limits.
*
*
* This action is throttled at one request per second.
*
*
* @param getSendQuotaRequest Container for the necessary parameters to
* execute the GetSendQuota operation on AmazonSimpleEmailService.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* GetSendQuota service method, as returned by AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getSendQuotaAsync(
final GetSendQuotaRequest getSendQuotaRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public GetSendQuotaResult call() throws Exception {
GetSendQuotaResult result;
try {
result = getSendQuota(getSendQuotaRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(getSendQuotaRequest, result);
return result;
}
});
}
/**
*
* Given an identity (email address or domain), enables or disables
* whether Amazon SES forwards feedback notifications as email. Feedback
* forwarding may only be disabled when both complaint and bounce topics
* are set.
*
*
* This action is throttled at one request per second.
*
*
* For more information about feedback notification, see the
* Amazon SES Developer Guide
* .
*
*
* @param setIdentityFeedbackForwardingEnabledRequest Container for the
* necessary parameters to execute the
* SetIdentityFeedbackForwardingEnabled operation on
* AmazonSimpleEmailService.
*
* @return A Java Future object containing the response from the
* SetIdentityFeedbackForwardingEnabled service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future setIdentityFeedbackForwardingEnabledAsync(final SetIdentityFeedbackForwardingEnabledRequest setIdentityFeedbackForwardingEnabledRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public SetIdentityFeedbackForwardingEnabledResult call() throws Exception {
return setIdentityFeedbackForwardingEnabled(setIdentityFeedbackForwardingEnabledRequest);
}
});
}
/**
*
* Given an identity (email address or domain), enables or disables
* whether Amazon SES forwards feedback notifications as email. Feedback
* forwarding may only be disabled when both complaint and bounce topics
* are set.
*
*
* This action is throttled at one request per second.
*
*
* For more information about feedback notification, see the
* Amazon SES Developer Guide
* .
*
*
* @param setIdentityFeedbackForwardingEnabledRequest Container for the
* necessary parameters to execute the
* SetIdentityFeedbackForwardingEnabled operation on
* AmazonSimpleEmailService.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* SetIdentityFeedbackForwardingEnabled service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future setIdentityFeedbackForwardingEnabledAsync(
final SetIdentityFeedbackForwardingEnabledRequest setIdentityFeedbackForwardingEnabledRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public SetIdentityFeedbackForwardingEnabledResult call() throws Exception {
SetIdentityFeedbackForwardingEnabledResult result;
try {
result = setIdentityFeedbackForwardingEnabled(setIdentityFeedbackForwardingEnabledRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(setIdentityFeedbackForwardingEnabledRequest, result);
return result;
}
});
}
/**
*
* Verifies a domain.
*
*
* This action is throttled at one request per second.
*
*
* @param verifyDomainIdentityRequest Container for the necessary
* parameters to execute the VerifyDomainIdentity operation on
* AmazonSimpleEmailService.
*
* @return A Java Future object containing the response from the
* VerifyDomainIdentity service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future verifyDomainIdentityAsync(final VerifyDomainIdentityRequest verifyDomainIdentityRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public VerifyDomainIdentityResult call() throws Exception {
return verifyDomainIdentity(verifyDomainIdentityRequest);
}
});
}
/**
*
* Verifies a domain.
*
*
* This action is throttled at one request per second.
*
*
* @param verifyDomainIdentityRequest Container for the necessary
* parameters to execute the VerifyDomainIdentity operation on
* AmazonSimpleEmailService.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* VerifyDomainIdentity service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future verifyDomainIdentityAsync(
final VerifyDomainIdentityRequest verifyDomainIdentityRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public VerifyDomainIdentityResult call() throws Exception {
VerifyDomainIdentityResult result;
try {
result = verifyDomainIdentity(verifyDomainIdentityRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(verifyDomainIdentityRequest, result);
return result;
}
});
}
/**
*
* Composes an email message based on input data, and then immediately
* queues the message for sending.
*
*
* IMPORTANT: You can only send email from verified email
* addresses and domains. If you have not requested production access to
* Amazon SES, you must also verify every recipient email address except
* for the recipients provided by the Amazon SES mailbox simulator. For
* more information, go to the Amazon SES Developer Guide.
*
*
* The total size of the message cannot exceed 10 MB.
*
*
* Amazon SES has a limit on the total number of recipients per message:
* The combined number of To:, CC: and BCC: email addresses cannot exceed
* 50. If you need to send an email message to a larger audience, you can
* divide your recipient list into groups of 50 or fewer, and then call
* Amazon SES repeatedly to send the message to each group.
*
*
* For every message that you send, the total number of recipients (To:,
* CC: and BCC:) is counted against your sending quota - the
* maximum number of emails you can send in a 24-hour period. For
* information about your sending quota, go to the
* Amazon SES Developer Guide
* .
*
*
* @param sendEmailRequest Container for the necessary parameters to
* execute the SendEmail operation on AmazonSimpleEmailService.
*
* @return A Java Future object containing the response from the
* SendEmail service method, as returned by AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future sendEmailAsync(final SendEmailRequest sendEmailRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public SendEmailResult call() throws Exception {
return sendEmail(sendEmailRequest);
}
});
}
/**
*
* Composes an email message based on input data, and then immediately
* queues the message for sending.
*
*
* IMPORTANT: You can only send email from verified email
* addresses and domains. If you have not requested production access to
* Amazon SES, you must also verify every recipient email address except
* for the recipients provided by the Amazon SES mailbox simulator. For
* more information, go to the Amazon SES Developer Guide.
*
*
* The total size of the message cannot exceed 10 MB.
*
*
* Amazon SES has a limit on the total number of recipients per message:
* The combined number of To:, CC: and BCC: email addresses cannot exceed
* 50. If you need to send an email message to a larger audience, you can
* divide your recipient list into groups of 50 or fewer, and then call
* Amazon SES repeatedly to send the message to each group.
*
*
* For every message that you send, the total number of recipients (To:,
* CC: and BCC:) is counted against your sending quota - the
* maximum number of emails you can send in a 24-hour period. For
* information about your sending quota, go to the
* Amazon SES Developer Guide
* .
*
*
* @param sendEmailRequest Container for the necessary parameters to
* execute the SendEmail operation on AmazonSimpleEmailService.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* SendEmail service method, as returned by AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future sendEmailAsync(
final SendEmailRequest sendEmailRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public SendEmailResult call() throws Exception {
SendEmailResult result;
try {
result = sendEmail(sendEmailRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(sendEmailRequest, result);
return result;
}
});
}
/**
*
* Deletes the specified email address from the list of verified
* addresses.
*
*
* IMPORTANT:The DeleteVerifiedEmailAddress action is deprecated
* as of the May 15, 2012 release of Domain Verification. The
* DeleteIdentity action is now preferred.
*
*
* This action is throttled at one request per second.
*
*
* @param deleteVerifiedEmailAddressRequest Container for the necessary
* parameters to execute the DeleteVerifiedEmailAddress operation on
* AmazonSimpleEmailService.
*
* @return A Java Future object containing the response from the
* DeleteVerifiedEmailAddress service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteVerifiedEmailAddressAsync(final DeleteVerifiedEmailAddressRequest deleteVerifiedEmailAddressRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
deleteVerifiedEmailAddress(deleteVerifiedEmailAddressRequest);
return null;
}
});
}
/**
*
* Deletes the specified email address from the list of verified
* addresses.
*
*
* IMPORTANT:The DeleteVerifiedEmailAddress action is deprecated
* as of the May 15, 2012 release of Domain Verification. The
* DeleteIdentity action is now preferred.
*
*
* This action is throttled at one request per second.
*
*
* @param deleteVerifiedEmailAddressRequest Container for the necessary
* parameters to execute the DeleteVerifiedEmailAddress operation on
* AmazonSimpleEmailService.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeleteVerifiedEmailAddress service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deleteVerifiedEmailAddressAsync(
final DeleteVerifiedEmailAddressRequest deleteVerifiedEmailAddressRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public Void call() throws Exception {
try {
deleteVerifiedEmailAddress(deleteVerifiedEmailAddressRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(deleteVerifiedEmailAddressRequest, null);
return null;
}
});
}
/**
*
* Given an identity (email address or domain), sets the Amazon SNS topic
* to which Amazon SES will publish bounce and complaint notifications
* for emails sent with that identity as the Source
.
* Publishing to topics may only be disabled when feedback
* forwarding is enabled.
*
*
* This action is throttled at one request per second.
*
*
* For more information about feedback notification, see the
* Amazon SES Developer Guide
* .
*
*
* @param setIdentityNotificationTopicRequest Container for the necessary
* parameters to execute the SetIdentityNotificationTopic operation on
* AmazonSimpleEmailService.
*
* @return A Java Future object containing the response from the
* SetIdentityNotificationTopic service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future setIdentityNotificationTopicAsync(final SetIdentityNotificationTopicRequest setIdentityNotificationTopicRequest)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public SetIdentityNotificationTopicResult call() throws Exception {
return setIdentityNotificationTopic(setIdentityNotificationTopicRequest);
}
});
}
/**
*
* Given an identity (email address or domain), sets the Amazon SNS topic
* to which Amazon SES will publish bounce and complaint notifications
* for emails sent with that identity as the Source
.
* Publishing to topics may only be disabled when feedback
* forwarding is enabled.
*
*
* This action is throttled at one request per second.
*
*
* For more information about feedback notification, see the
* Amazon SES Developer Guide
* .
*
*
* @param setIdentityNotificationTopicRequest Container for the necessary
* parameters to execute the SetIdentityNotificationTopic operation on
* AmazonSimpleEmailService.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* SetIdentityNotificationTopic service method, as returned by
* AmazonSimpleEmailService.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleEmailService indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future setIdentityNotificationTopicAsync(
final SetIdentityNotificationTopicRequest setIdentityNotificationTopicRequest,
final AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException {
return executorService.submit(new Callable() {
public SetIdentityNotificationTopicResult call() throws Exception {
SetIdentityNotificationTopicResult result;
try {
result = setIdentityNotificationTopic(setIdentityNotificationTopicRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(setIdentityNotificationTopicRequest, result);
return result;
}
});
}
}