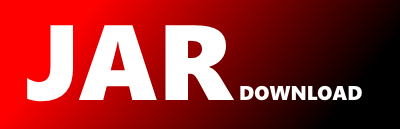
com.amazonaws.services.simpleworkflow.AmazonSimpleWorkflowAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.simpleworkflow;
import java.util.concurrent.Future;
import com.amazonaws.AmazonClientException;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.handlers.AsyncHandler;
import com.amazonaws.services.simpleworkflow.model.*;
/**
* Interface for accessing AmazonSimpleWorkflow asynchronously.
* Each asynchronous method will return a Java Future object, and users are also allowed
* to provide a callback handler.
* Amazon Simple Workflow Service
* The Amazon Simple Workflow Service API Reference is intended for
* programmers who need detailed information about the Amazon SWF actions
* and data types.
*
*
* For an broader overview of the Amazon SWF programming model, please
* go to the
* Amazon SWF Developer Guide
* .
*
*
* This section provides an overview of Amazon SWF actions.
*
*
* Action Categories
*
*
* The Amazon SWF actions can be grouped into the following major
* categories.
*
*
*
* -
* Actions related to Activities
*
*
* -
* Actions related to Deciders
*
*
* -
* Actions related to Workflow Executions
*
*
* -
* Actions related to Administration
*
*
* -
* Actions related to Visibility
*
*
*
*
*
* Actions related to Activities
*
*
* The following are actions that are performed by activity workers:
*
*
*
* -
* PollForActivityTask
*
*
* -
* RespondActivityTaskCompleted
*
*
* -
* RespondActivityTaskFailed
*
*
* -
* RespondActivityTaskCanceled
*
*
* -
* RecordActivityTaskHeartbeat
*
*
*
*
*
* Activity workers use the PollForActivityTask to get new activity
* tasks. After a worker receives an activity task from Amazon SWF, it
* performs the task and responds using RespondActivityTaskCompleted if
* successful or RespondActivityTaskFailed if unsuccessful.
*
*
* Actions related to Deciders
*
*
* The following are actions that are performed by deciders:
*
*
*
* -
* PollForDecisionTask
*
*
* -
* RespondDecisionTaskCompleted
*
*
*
*
*
* Deciders use PollForDecisionTask to get decision tasks. After a
* decider receives a decision task from Amazon SWF, it examines its
* workflow execution history and decides what to do next. It calls
* RespondDecisionTaskCompletedto complete the decision task and provide
* zero or more next decisions.
*
*
* Actions related to Workflow Executions
*
*
* The following actions operate on a workflow execution:
*
*
*
* -
* RequestCancelWorkflowExecution
*
*
* -
* StartWorkflowExecution
*
*
* -
* SignalWorkflowExecution
*
*
* -
* TerminateWorkflowExecution
*
*
*
*
*
* Actions related to Administration
*
*
* Although you can perform administrative tasks from the Amazon SWF
* console, you can use the actions in this section to automate functions
* or build your own administrative tools.
*
*
* Activity Management
*
*
*
* -
* RegisterActivityType
*
*
* -
* DeprecateActivityType
*
*
*
*
*
* Workflow Management
*
*
*
* -
* RegisterWorkflowType
*
*
* -
* DeprecateWorkflowType
*
*
*
*
*
* Domain Management
*
*
*
* -
* RegisterDomain
*
*
* -
* DeprecateDomain
*
*
*
*
*
* Workflow Execution Management
*
*
*
* -
* RequestCancelWorkflowExecution
*
*
* -
* TerminateWorkflowExecution
*
*
*
*
*
* Visibility Actions
*
*
* Although you can perform visibility actions from the Amazon SWF
* console, you can use the actions in this section to build your own
* console or administrative tools.
*
*
*
*
*
*
* Activity Visibility
*
*
*
* -
* ListActivityTypes
*
*
* -
* DescribeActivity
*
*
*
*
*
* Workflow Visibility
*
*
*
* -
* ListWorkflowTypes
*
*
* -
* DescribeWorkflowType
*
*
*
*
*
* Workflow Execution Visibility
*
*
*
* -
* DescribeWorkflowExecution
*
*
* -
* ListOpenWorkflowExecutions
*
*
* -
* ListClosedWorkflowExecutions
*
*
* -
* CountOpenWorkflowExecutions
*
*
* -
* CountClosedWorkflowExecutions
*
*
* -
* GetWorkflowExecutionHistory
*
*
*
*
*
* Domain Visibility
*
*
*
* -
* ListDomains
*
*
* -
* DescribeDomain
*
*
*
*
*
* Task List Visibility
*
*
*
* -
* CountPendingActivityTasks
*
*
* -
* CountPendingDecisionTasks
*
*
*
*
*/
public interface AmazonSimpleWorkflowAsync extends AmazonSimpleWorkflow {
/**
*
* Deprecates the specified workflow type . After a workflow type
* has been deprecated, you cannot create new executions of that type.
* Executions that were started before the type was deprecated will
* continue to run. A deprecated workflow type may still be used when
* calling visibility actions.
*
*
* NOTE: This operation is eventually consistent. The results are
* best effort and may not exactly reflect recent updates and changes.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Constrain the following parameters by using a
*
Condition
element with the appropriate keys.
*
* -
workflowType.name
: String constraint. The key is
* swf:workflowType.name
.
* -
workflowType.version
: String constraint. The key
* is swf:workflowType.version
.
*
*
*
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param deprecateWorkflowTypeRequest Container for the necessary
* parameters to execute the DeprecateWorkflowType operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* DeprecateWorkflowType service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deprecateWorkflowTypeAsync(DeprecateWorkflowTypeRequest deprecateWorkflowTypeRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deprecates the specified workflow type . After a workflow type
* has been deprecated, you cannot create new executions of that type.
* Executions that were started before the type was deprecated will
* continue to run. A deprecated workflow type may still be used when
* calling visibility actions.
*
*
* NOTE: This operation is eventually consistent. The results are
* best effort and may not exactly reflect recent updates and changes.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Constrain the following parameters by using a
*
Condition
element with the appropriate keys.
*
* -
workflowType.name
: String constraint. The key is
* swf:workflowType.name
.
* -
workflowType.version
: String constraint. The key
* is swf:workflowType.version
.
*
*
*
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param deprecateWorkflowTypeRequest Container for the necessary
* parameters to execute the DeprecateWorkflowType operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeprecateWorkflowType service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deprecateWorkflowTypeAsync(DeprecateWorkflowTypeRequest deprecateWorkflowTypeRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deprecates the specified domain. After a domain has been deprecated
* it cannot be used to create new workflow executions or register new
* types. However, you can still use visibility actions on this domain.
* Deprecating a domain also deprecates all activity and workflow types
* registered in the domain. Executions that were started before the
* domain was deprecated will continue to run.
*
*
* NOTE: This operation is eventually consistent. The results are
* best effort and may not exactly reflect recent updates and changes.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param deprecateDomainRequest Container for the necessary parameters
* to execute the DeprecateDomain operation on AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* DeprecateDomain service method, as returned by AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deprecateDomainAsync(DeprecateDomainRequest deprecateDomainRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deprecates the specified domain. After a domain has been deprecated
* it cannot be used to create new workflow executions or register new
* types. However, you can still use visibility actions on this domain.
* Deprecating a domain also deprecates all activity and workflow types
* registered in the domain. Executions that were started before the
* domain was deprecated will continue to run.
*
*
* NOTE: This operation is eventually consistent. The results are
* best effort and may not exactly reflect recent updates and changes.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param deprecateDomainRequest Container for the necessary parameters
* to execute the DeprecateDomain operation on AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeprecateDomain service method, as returned by AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deprecateDomainAsync(DeprecateDomainRequest deprecateDomainRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Registers a new workflow type and its configuration settings
* in the specified domain.
*
*
* The retention period for the workflow history is set by the
* RegisterDomain action.
*
*
* IMPORTANT: If the type already exists, then a TypeAlreadyExists
* fault is returned. You cannot change the configuration settings of a
* workflow type once it is registered and it must be registered as a new
* version.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Constrain the following parameters by using a
*
Condition
element with the appropriate keys.
*
* -
defaultTaskList
: String constraint. The key is
* swf:defaultTaskList.name
.
* -
name
: String constraint. The key is
* swf:name
.
* -
version
: String constraint. The key is
* swf:version
.
*
*
*
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param registerWorkflowTypeRequest Container for the necessary
* parameters to execute the RegisterWorkflowType operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* RegisterWorkflowType service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future registerWorkflowTypeAsync(RegisterWorkflowTypeRequest registerWorkflowTypeRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Registers a new workflow type and its configuration settings
* in the specified domain.
*
*
* The retention period for the workflow history is set by the
* RegisterDomain action.
*
*
* IMPORTANT: If the type already exists, then a TypeAlreadyExists
* fault is returned. You cannot change the configuration settings of a
* workflow type once it is registered and it must be registered as a new
* version.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Constrain the following parameters by using a
*
Condition
element with the appropriate keys.
*
* -
defaultTaskList
: String constraint. The key is
* swf:defaultTaskList.name
.
* -
name
: String constraint. The key is
* swf:name
.
* -
version
: String constraint. The key is
* swf:version
.
*
*
*
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param registerWorkflowTypeRequest Container for the necessary
* parameters to execute the RegisterWorkflowType operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RegisterWorkflowType service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future registerWorkflowTypeAsync(RegisterWorkflowTypeRequest registerWorkflowTypeRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about workflow types in the specified domain. The
* results may be split into multiple pages that can be retrieved by
* making the call repeatedly.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param listWorkflowTypesRequest Container for the necessary parameters
* to execute the ListWorkflowTypes operation on AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* ListWorkflowTypes service method, as returned by AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listWorkflowTypesAsync(ListWorkflowTypesRequest listWorkflowTypesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about workflow types in the specified domain. The
* results may be split into multiple pages that can be retrieved by
* making the call repeatedly.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param listWorkflowTypesRequest Container for the necessary parameters
* to execute the ListWorkflowTypes operation on AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListWorkflowTypes service method, as returned by AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listWorkflowTypesAsync(ListWorkflowTypesRequest listWorkflowTypesRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Starts an execution of the workflow type in the specified domain
* using the provided workflowId
and input data.
*
*
* This action returns the newly started workflow execution.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Constrain the following parameters by using a
*
Condition
element with the appropriate keys.
*
* -
tagList.member.0
: The key is
* swf:tagList.member.0
.
* -
tagList.member.1
: The key is
* swf:tagList.member.1
.
* -
tagList.member.2
: The key is
* swf:tagList.member.2
.
* -
tagList.member.3
: The key is
* swf:tagList.member.3
.
* -
tagList.member.4
: The key is
* swf:tagList.member.4
.
* -
taskList
: String constraint. The key is
* swf:taskList.name
.
* -
name
: String constraint. The key is
* swf:workflowType.name
.
* -
version
: String constraint. The key is
* swf:workflowType.version
.
*
*
*
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param startWorkflowExecutionRequest Container for the necessary
* parameters to execute the StartWorkflowExecution operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* StartWorkflowExecution service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future startWorkflowExecutionAsync(StartWorkflowExecutionRequest startWorkflowExecutionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Starts an execution of the workflow type in the specified domain
* using the provided workflowId
and input data.
*
*
* This action returns the newly started workflow execution.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Constrain the following parameters by using a
*
Condition
element with the appropriate keys.
*
* -
tagList.member.0
: The key is
* swf:tagList.member.0
.
* -
tagList.member.1
: The key is
* swf:tagList.member.1
.
* -
tagList.member.2
: The key is
* swf:tagList.member.2
.
* -
tagList.member.3
: The key is
* swf:tagList.member.3
.
* -
tagList.member.4
: The key is
* swf:tagList.member.4
.
* -
taskList
: String constraint. The key is
* swf:taskList.name
.
* -
name
: String constraint. The key is
* swf:workflowType.name
.
* -
version
: String constraint. The key is
* swf:workflowType.version
.
*
*
*
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param startWorkflowExecutionRequest Container for the necessary
* parameters to execute the StartWorkflowExecution operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* StartWorkflowExecution service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future startWorkflowExecutionAsync(StartWorkflowExecutionRequest startWorkflowExecutionRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Records a WorkflowExecutionSignaled
event in the
* workflow execution history and creates a decision task for the
* workflow execution identified by the given domain, workflowId and
* runId. The event is recorded with the specified user defined
* signalName and input (if provided).
*
*
* NOTE: If a runId is not specified, then the
* WorkflowExecutionSignaled event is recorded in the history of the
* current open workflow with the matching workflowId in the domain.
*
*
* NOTE: If the specified workflow execution is not open, this
* method fails with UnknownResource.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param signalWorkflowExecutionRequest Container for the necessary
* parameters to execute the SignalWorkflowExecution operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* SignalWorkflowExecution service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future signalWorkflowExecutionAsync(SignalWorkflowExecutionRequest signalWorkflowExecutionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Records a WorkflowExecutionSignaled
event in the
* workflow execution history and creates a decision task for the
* workflow execution identified by the given domain, workflowId and
* runId. The event is recorded with the specified user defined
* signalName and input (if provided).
*
*
* NOTE: If a runId is not specified, then the
* WorkflowExecutionSignaled event is recorded in the history of the
* current open workflow with the matching workflowId in the domain.
*
*
* NOTE: If the specified workflow execution is not open, this
* method fails with UnknownResource.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param signalWorkflowExecutionRequest Container for the necessary
* parameters to execute the SignalWorkflowExecution operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* SignalWorkflowExecution service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future signalWorkflowExecutionAsync(SignalWorkflowExecutionRequest signalWorkflowExecutionRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the list of domains registered in the account. The results
* may be split into multiple pages. To retrieve subsequent pages, make
* the call again using the nextPageToken returned by the initial call.
*
*
* NOTE: This operation is eventually consistent. The results are
* best effort and may not exactly reflect recent updates and changes.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains. The element must be set to
* arn:aws:swf::AccountID:domain/*"
, where ???AccountID" is
* the account ID, with no dashes.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param listDomainsRequest Container for the necessary parameters to
* execute the ListDomains operation on AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* ListDomains service method, as returned by AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listDomainsAsync(ListDomainsRequest listDomainsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the list of domains registered in the account. The results
* may be split into multiple pages. To retrieve subsequent pages, make
* the call again using the nextPageToken returned by the initial call.
*
*
* NOTE: This operation is eventually consistent. The results are
* best effort and may not exactly reflect recent updates and changes.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains. The element must be set to
* arn:aws:swf::AccountID:domain/*"
, where ???AccountID" is
* the account ID, with no dashes.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param listDomainsRequest Container for the necessary parameters to
* execute the ListDomains operation on AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListDomains service method, as returned by AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listDomainsAsync(ListDomainsRequest listDomainsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Records a WorkflowExecutionCancelRequested
event in the
* currently running workflow execution identified by the given domain,
* workflowId, and runId. This logically requests the cancellation of the
* workflow execution as a whole. It is up to the decider to take
* appropriate actions when it receives an execution history with this
* event.
*
*
* NOTE: If the runId is not specified, the
* WorkflowExecutionCancelRequested event is recorded in the history of
* the current open workflow execution with the specified workflowId in
* the domain.
*
*
* NOTE: Because this action allows the workflow to properly clean
* up and gracefully close, it should be used instead of
* TerminateWorkflowExecution when possible.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param requestCancelWorkflowExecutionRequest Container for the
* necessary parameters to execute the RequestCancelWorkflowExecution
* operation on AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* RequestCancelWorkflowExecution service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future requestCancelWorkflowExecutionAsync(RequestCancelWorkflowExecutionRequest requestCancelWorkflowExecutionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Records a WorkflowExecutionCancelRequested
event in the
* currently running workflow execution identified by the given domain,
* workflowId, and runId. This logically requests the cancellation of the
* workflow execution as a whole. It is up to the decider to take
* appropriate actions when it receives an execution history with this
* event.
*
*
* NOTE: If the runId is not specified, the
* WorkflowExecutionCancelRequested event is recorded in the history of
* the current open workflow execution with the specified workflowId in
* the domain.
*
*
* NOTE: Because this action allows the workflow to properly clean
* up and gracefully close, it should be used instead of
* TerminateWorkflowExecution when possible.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param requestCancelWorkflowExecutionRequest Container for the
* necessary parameters to execute the RequestCancelWorkflowExecution
* operation on AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RequestCancelWorkflowExecution service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future requestCancelWorkflowExecutionAsync(RequestCancelWorkflowExecutionRequest requestCancelWorkflowExecutionRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about the specified workflow type . This
* includes configuration settings specified when the type was registered
* and other information such as creation date, current status, etc.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Constrain the following parameters by using a
*
Condition
element with the appropriate keys.
*
* -
workflowType.name
: String constraint. The key is
* swf:workflowType.name
.
* -
workflowType.version
: String constraint. The key
* is swf:workflowType.version
.
*
*
*
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param describeWorkflowTypeRequest Container for the necessary
* parameters to execute the DescribeWorkflowType operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* DescribeWorkflowType service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeWorkflowTypeAsync(DescribeWorkflowTypeRequest describeWorkflowTypeRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about the specified workflow type . This
* includes configuration settings specified when the type was registered
* and other information such as creation date, current status, etc.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Constrain the following parameters by using a
*
Condition
element with the appropriate keys.
*
* -
workflowType.name
: String constraint. The key is
* swf:workflowType.name
.
* -
workflowType.version
: String constraint. The key
* is swf:workflowType.version
.
*
*
*
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param describeWorkflowTypeRequest Container for the necessary
* parameters to execute the DescribeWorkflowType operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeWorkflowType service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeWorkflowTypeAsync(DescribeWorkflowTypeRequest describeWorkflowTypeRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deprecates the specified activity type .
* After an activity type has been deprecated, you cannot create
* new tasks of that activity type. Tasks of this type that were
* scheduled before the type was deprecated will continue to run.
*
*
* NOTE: This operation is eventually consistent. The results are
* best effort and may not exactly reflect recent updates and changes.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Constrain the following parameters by using a
*
Condition
element with the appropriate keys.
*
* -
activityType.name
: String constraint. The key is
* swf:activityType.name
.
* -
activityType.version
: String constraint. The key
* is swf:activityType.version
.
*
*
*
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param deprecateActivityTypeRequest Container for the necessary
* parameters to execute the DeprecateActivityType operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* DeprecateActivityType service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deprecateActivityTypeAsync(DeprecateActivityTypeRequest deprecateActivityTypeRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Deprecates the specified activity type .
* After an activity type has been deprecated, you cannot create
* new tasks of that activity type. Tasks of this type that were
* scheduled before the type was deprecated will continue to run.
*
*
* NOTE: This operation is eventually consistent. The results are
* best effort and may not exactly reflect recent updates and changes.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Constrain the following parameters by using a
*
Condition
element with the appropriate keys.
*
* -
activityType.name
: String constraint. The key is
* swf:activityType.name
.
* -
activityType.version
: String constraint. The key
* is swf:activityType.version
.
*
*
*
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param deprecateActivityTypeRequest Container for the necessary
* parameters to execute the DeprecateActivityType operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DeprecateActivityType service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future deprecateActivityTypeAsync(DeprecateActivityTypeRequest deprecateActivityTypeRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the number of closed workflow executions within the given
* domain that meet the specified filtering criteria.
*
*
* NOTE: This operation is eventually consistent. The results are
* best effort and may not exactly reflect recent updates and changes.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Constrain the following parameters by using a
*
Condition
element with the appropriate keys.
*
* -
tag
: String constraint. The key is
* swf:tagFilter.tag
.
* -
typeFilter.name
: String constraint. String
* constraint. The key is swf:typeFilter.name
.
* -
typeFilter.version
: String constraint. String
* constraint. The key is swf:typeFilter.version
.
*
*
*
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param countClosedWorkflowExecutionsRequest Container for the
* necessary parameters to execute the CountClosedWorkflowExecutions
* operation on AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* CountClosedWorkflowExecutions service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future countClosedWorkflowExecutionsAsync(CountClosedWorkflowExecutionsRequest countClosedWorkflowExecutionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the number of closed workflow executions within the given
* domain that meet the specified filtering criteria.
*
*
* NOTE: This operation is eventually consistent. The results are
* best effort and may not exactly reflect recent updates and changes.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Constrain the following parameters by using a
*
Condition
element with the appropriate keys.
*
* -
tag
: String constraint. The key is
* swf:tagFilter.tag
.
* -
typeFilter.name
: String constraint. String
* constraint. The key is swf:typeFilter.name
.
* -
typeFilter.version
: String constraint. String
* constraint. The key is swf:typeFilter.version
.
*
*
*
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param countClosedWorkflowExecutionsRequest Container for the
* necessary parameters to execute the CountClosedWorkflowExecutions
* operation on AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CountClosedWorkflowExecutions service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future countClosedWorkflowExecutionsAsync(CountClosedWorkflowExecutionsRequest countClosedWorkflowExecutionsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the estimated number of activity tasks in the specified task
* list. The count returned is an approximation and is not guaranteed to
* be exact. If you specify a task list that no activity task was ever
* scheduled in then 0 will be returned.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Use a Condition element with the
*
swf:taskList.name
key to allow the action to access only
* certain task lists.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param countPendingActivityTasksRequest Container for the necessary
* parameters to execute the CountPendingActivityTasks operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* CountPendingActivityTasks service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future countPendingActivityTasksAsync(CountPendingActivityTasksRequest countPendingActivityTasksRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the estimated number of activity tasks in the specified task
* list. The count returned is an approximation and is not guaranteed to
* be exact. If you specify a task list that no activity task was ever
* scheduled in then 0 will be returned.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Use a Condition element with the
*
swf:taskList.name
key to allow the action to access only
* certain task lists.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param countPendingActivityTasksRequest Container for the necessary
* parameters to execute the CountPendingActivityTasks operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CountPendingActivityTasks service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future countPendingActivityTasksAsync(CountPendingActivityTasksRequest countPendingActivityTasksRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Used by workers to tell the service that the ActivityTask identified
* by the taskToken
was successfully canceled. Additional
* details
can be optionally provided using the
* details
argument.
*
*
* These details
(if provided) appear in the
* ActivityTaskCanceled
event added to the workflow history.
*
*
* IMPORTANT: Only use this operation if the canceled flag of a
* RecordActivityTaskHeartbeat request returns true and if the activity
* can be safely undone or abandoned.
*
*
* A task is considered open from the time that it is scheduled until it
* is closed. Therefore a task is reported as open while a worker is
* processing it. A task is closed after it has been specified in a call
* to RespondActivityTaskCompleted, RespondActivityTaskCanceled,
* RespondActivityTaskFailed, or the task has
* timed out
* .
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param respondActivityTaskCanceledRequest Container for the necessary
* parameters to execute the RespondActivityTaskCanceled operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* RespondActivityTaskCanceled service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future respondActivityTaskCanceledAsync(RespondActivityTaskCanceledRequest respondActivityTaskCanceledRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Used by workers to tell the service that the ActivityTask identified
* by the taskToken
was successfully canceled. Additional
* details
can be optionally provided using the
* details
argument.
*
*
* These details
(if provided) appear in the
* ActivityTaskCanceled
event added to the workflow history.
*
*
* IMPORTANT: Only use this operation if the canceled flag of a
* RecordActivityTaskHeartbeat request returns true and if the activity
* can be safely undone or abandoned.
*
*
* A task is considered open from the time that it is scheduled until it
* is closed. Therefore a task is reported as open while a worker is
* processing it. A task is closed after it has been specified in a call
* to RespondActivityTaskCompleted, RespondActivityTaskCanceled,
* RespondActivityTaskFailed, or the task has
* timed out
* .
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param respondActivityTaskCanceledRequest Container for the necessary
* parameters to execute the RespondActivityTaskCanceled operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RespondActivityTaskCanceled service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future respondActivityTaskCanceledAsync(RespondActivityTaskCanceledRequest respondActivityTaskCanceledRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Used by deciders to tell the service that the DecisionTask identified
* by the taskToken
has successfully completed. The
* decisions
argument specifies the list of decisions made
* while processing the task.
*
*
* A
* DecisionTaskCompleted
event is added
* to the workflow history. The executionContext
specified
* is attached to the event in the workflow execution history.
*
*
* Access Control
*
*
* If an IAM policy grants permission to use
* RespondDecisionTaskCompleted
, it can express permissions
* for the list of decisions in the decisions
parameter in
* the same way as for the regular API. This approach maintains a uniform
* conceptual model and helps keep policies readable. For more
* information, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param respondDecisionTaskCompletedRequest Container for the necessary
* parameters to execute the RespondDecisionTaskCompleted operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* RespondDecisionTaskCompleted service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future respondDecisionTaskCompletedAsync(RespondDecisionTaskCompletedRequest respondDecisionTaskCompletedRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Used by deciders to tell the service that the DecisionTask identified
* by the taskToken
has successfully completed. The
* decisions
argument specifies the list of decisions made
* while processing the task.
*
*
* A
* DecisionTaskCompleted
event is added
* to the workflow history. The executionContext
specified
* is attached to the event in the workflow execution history.
*
*
* Access Control
*
*
* If an IAM policy grants permission to use
* RespondDecisionTaskCompleted
, it can express permissions
* for the list of decisions in the decisions
parameter in
* the same way as for the regular API. This approach maintains a uniform
* conceptual model and helps keep policies readable. For more
* information, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param respondDecisionTaskCompletedRequest Container for the necessary
* parameters to execute the RespondDecisionTaskCompleted operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RespondDecisionTaskCompleted service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future respondDecisionTaskCompletedAsync(RespondDecisionTaskCompletedRequest respondDecisionTaskCompletedRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Used by workers to tell the service that the ActivityTask identified
* by the taskToken
completed successfully with a
* result
(if provided). The result
appears in
* the ActivityTaskCompleted
event in the workflow history.
*
*
* IMPORTANT: If the requested task does not complete
* successfully, use RespondActivityTaskFailed instead. If the worker
* finds that the task is canceled through the canceled flag returned by
* RecordActivityTaskHeartbeat, it should cancel the task, clean up and
* then call RespondActivityTaskCanceled.
*
*
* A task is considered open from the time that it is scheduled until it
* is closed. Therefore a task is reported as open while a worker is
* processing it. A task is closed after it has been specified in a call
* to RespondActivityTaskCompleted, RespondActivityTaskCanceled,
* RespondActivityTaskFailed, or the task has
* timed out
* .
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param respondActivityTaskCompletedRequest Container for the necessary
* parameters to execute the RespondActivityTaskCompleted operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* RespondActivityTaskCompleted service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future respondActivityTaskCompletedAsync(RespondActivityTaskCompletedRequest respondActivityTaskCompletedRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Used by workers to tell the service that the ActivityTask identified
* by the taskToken
completed successfully with a
* result
(if provided). The result
appears in
* the ActivityTaskCompleted
event in the workflow history.
*
*
* IMPORTANT: If the requested task does not complete
* successfully, use RespondActivityTaskFailed instead. If the worker
* finds that the task is canceled through the canceled flag returned by
* RecordActivityTaskHeartbeat, it should cancel the task, clean up and
* then call RespondActivityTaskCanceled.
*
*
* A task is considered open from the time that it is scheduled until it
* is closed. Therefore a task is reported as open while a worker is
* processing it. A task is closed after it has been specified in a call
* to RespondActivityTaskCompleted, RespondActivityTaskCanceled,
* RespondActivityTaskFailed, or the task has
* timed out
* .
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param respondActivityTaskCompletedRequest Container for the necessary
* parameters to execute the RespondActivityTaskCompleted operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RespondActivityTaskCompleted service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future respondActivityTaskCompletedAsync(RespondActivityTaskCompletedRequest respondActivityTaskCompletedRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Used by workers to get an ActivityTask from the specified activity
* taskList
.
* This initiates a long poll, where the service holds the HTTP
* connection open and responds as soon as a task becomes available. The
* maximum time the service holds on to the request before responding is
* 60 seconds. If no task is available within 60 seconds, the poll will
* return an empty result. An empty result, in this context, means that
* an ActivityTask is returned, but that the value of taskToken is an
* empty string. If a task is returned, the worker should use its type to
* identify and process it correctly.
*
*
* IMPORTANT: Workers should set their client side socket timeout
* to at least 70 seconds (10 seconds higher than the maximum time
* service may hold the poll request).
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Use a Condition element with the
*
swf:taskList.name
key to allow the action to access only
* certain task lists.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param pollForActivityTaskRequest Container for the necessary
* parameters to execute the PollForActivityTask operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* PollForActivityTask service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future pollForActivityTaskAsync(PollForActivityTaskRequest pollForActivityTaskRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Used by workers to get an ActivityTask from the specified activity
* taskList
.
* This initiates a long poll, where the service holds the HTTP
* connection open and responds as soon as a task becomes available. The
* maximum time the service holds on to the request before responding is
* 60 seconds. If no task is available within 60 seconds, the poll will
* return an empty result. An empty result, in this context, means that
* an ActivityTask is returned, but that the value of taskToken is an
* empty string. If a task is returned, the worker should use its type to
* identify and process it correctly.
*
*
* IMPORTANT: Workers should set their client side socket timeout
* to at least 70 seconds (10 seconds higher than the maximum time
* service may hold the poll request).
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Use a Condition element with the
*
swf:taskList.name
key to allow the action to access only
* certain task lists.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param pollForActivityTaskRequest Container for the necessary
* parameters to execute the PollForActivityTask operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* PollForActivityTask service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future pollForActivityTaskAsync(PollForActivityTaskRequest pollForActivityTaskRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the number of open workflow executions within the given
* domain that meet the specified filtering criteria.
*
*
* NOTE: This operation is eventually consistent. The results are
* best effort and may not exactly reflect recent updates and changes.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Constrain the following parameters by using a
*
Condition
element with the appropriate keys.
*
* -
tag
: String constraint. The key is
* swf:tagFilter.tag
.
* -
typeFilter.name
: String constraint. String
* constraint. The key is swf:typeFilter.name
.
* -
typeFilter.version
: String constraint. String
* constraint. The key is swf:typeFilter.version
.
*
*
*
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param countOpenWorkflowExecutionsRequest Container for the necessary
* parameters to execute the CountOpenWorkflowExecutions operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* CountOpenWorkflowExecutions service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future countOpenWorkflowExecutionsAsync(CountOpenWorkflowExecutionsRequest countOpenWorkflowExecutionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the number of open workflow executions within the given
* domain that meet the specified filtering criteria.
*
*
* NOTE: This operation is eventually consistent. The results are
* best effort and may not exactly reflect recent updates and changes.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Constrain the following parameters by using a
*
Condition
element with the appropriate keys.
*
* -
tag
: String constraint. The key is
* swf:tagFilter.tag
.
* -
typeFilter.name
: String constraint. String
* constraint. The key is swf:typeFilter.name
.
* -
typeFilter.version
: String constraint. String
* constraint. The key is swf:typeFilter.version
.
*
*
*
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param countOpenWorkflowExecutionsRequest Container for the necessary
* parameters to execute the CountOpenWorkflowExecutions operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CountOpenWorkflowExecutions service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future countOpenWorkflowExecutionsAsync(CountOpenWorkflowExecutionsRequest countOpenWorkflowExecutionsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about the specified activity type. This includes
* configuration settings provided at registration time as well as other
* general information about the type.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Constrain the following parameters by using a
*
Condition
element with the appropriate keys.
*
* -
activityType.name
: String constraint. The key is
* swf:activityType.name
.
* -
activityType.version
: String constraint. The key
* is swf:activityType.version
.
*
*
*
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param describeActivityTypeRequest Container for the necessary
* parameters to execute the DescribeActivityType operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* DescribeActivityType service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeActivityTypeAsync(DescribeActivityTypeRequest describeActivityTypeRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about the specified activity type. This includes
* configuration settings provided at registration time as well as other
* general information about the type.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Constrain the following parameters by using a
*
Condition
element with the appropriate keys.
*
* -
activityType.name
: String constraint. The key is
* swf:activityType.name
.
* -
activityType.version
: String constraint. The key
* is swf:activityType.version
.
*
*
*
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param describeActivityTypeRequest Container for the necessary
* parameters to execute the DescribeActivityType operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeActivityType service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeActivityTypeAsync(DescribeActivityTypeRequest describeActivityTypeRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of open workflow executions in the specified domain
* that meet the filtering criteria. The results may be split into
* multiple pages. To retrieve subsequent pages, make the call again
* using the nextPageToken returned by the initial call.
*
*
* NOTE: This operation is eventually consistent. The results are
* best effort and may not exactly reflect recent updates and changes.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Constrain the following parameters by using a
*
Condition
element with the appropriate keys.
*
* -
tag
: String constraint. The key is
* swf:tagFilter.tag
.
* -
typeFilter.name
: String constraint. String
* constraint. The key is swf:typeFilter.name
.
* -
typeFilter.version
: String constraint. String
* constraint. The key is swf:typeFilter.version
.
*
*
*
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param listOpenWorkflowExecutionsRequest Container for the necessary
* parameters to execute the ListOpenWorkflowExecutions operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* ListOpenWorkflowExecutions service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listOpenWorkflowExecutionsAsync(ListOpenWorkflowExecutionsRequest listOpenWorkflowExecutionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of open workflow executions in the specified domain
* that meet the filtering criteria. The results may be split into
* multiple pages. To retrieve subsequent pages, make the call again
* using the nextPageToken returned by the initial call.
*
*
* NOTE: This operation is eventually consistent. The results are
* best effort and may not exactly reflect recent updates and changes.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Constrain the following parameters by using a
*
Condition
element with the appropriate keys.
*
* -
tag
: String constraint. The key is
* swf:tagFilter.tag
.
* -
typeFilter.name
: String constraint. String
* constraint. The key is swf:typeFilter.name
.
* -
typeFilter.version
: String constraint. String
* constraint. The key is swf:typeFilter.version
.
*
*
*
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param listOpenWorkflowExecutionsRequest Container for the necessary
* parameters to execute the ListOpenWorkflowExecutions operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListOpenWorkflowExecutions service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listOpenWorkflowExecutionsAsync(ListOpenWorkflowExecutionsRequest listOpenWorkflowExecutionsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the history of the specified workflow execution. The results
* may be split into multiple pages. To retrieve subsequent pages, make
* the call again using the nextPageToken
returned by the
* initial call.
*
*
* NOTE: This operation is eventually consistent. The results are
* best effort and may not exactly reflect recent updates and changes.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param getWorkflowExecutionHistoryRequest Container for the necessary
* parameters to execute the GetWorkflowExecutionHistory operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* GetWorkflowExecutionHistory service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getWorkflowExecutionHistoryAsync(GetWorkflowExecutionHistoryRequest getWorkflowExecutionHistoryRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the history of the specified workflow execution. The results
* may be split into multiple pages. To retrieve subsequent pages, make
* the call again using the nextPageToken
returned by the
* initial call.
*
*
* NOTE: This operation is eventually consistent. The results are
* best effort and may not exactly reflect recent updates and changes.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param getWorkflowExecutionHistoryRequest Container for the necessary
* parameters to execute the GetWorkflowExecutionHistory operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* GetWorkflowExecutionHistory service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future getWorkflowExecutionHistoryAsync(GetWorkflowExecutionHistoryRequest getWorkflowExecutionHistoryRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Registers a new domain.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - You cannot use an IAM policy to control domain access for this
* action. The name of the domain being registered is available as the
* resource of this action.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
*
*
* @param registerDomainRequest Container for the necessary parameters to
* execute the RegisterDomain operation on AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* RegisterDomain service method, as returned by AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future registerDomainAsync(RegisterDomainRequest registerDomainRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Registers a new domain.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - You cannot use an IAM policy to control domain access for this
* action. The name of the domain being registered is available as the
* resource of this action.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
*
*
* @param registerDomainRequest Container for the necessary parameters to
* execute the RegisterDomain operation on AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RegisterDomain service method, as returned by AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future registerDomainAsync(RegisterDomainRequest registerDomainRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Registers a new activity type along with its configuration
* settings in the specified domain.
*
*
* IMPORTANT: A TypeAlreadyExists fault is returned if the type
* already exists in the domain. You cannot change any configuration
* settings of the type after its registration, and it must be registered
* as a new version.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Constrain the following parameters by using a
*
Condition
element with the appropriate keys.
*
* -
defaultTaskList
: String constraint. The key is
* swf:defaultTaskList.name
.
* -
name
: String constraint. The key is
* swf:name
.
* -
version
: String constraint. The key is
* swf:version
.
*
*
*
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param registerActivityTypeRequest Container for the necessary
* parameters to execute the RegisterActivityType operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* RegisterActivityType service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future registerActivityTypeAsync(RegisterActivityTypeRequest registerActivityTypeRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Registers a new activity type along with its configuration
* settings in the specified domain.
*
*
* IMPORTANT: A TypeAlreadyExists fault is returned if the type
* already exists in the domain. You cannot change any configuration
* settings of the type after its registration, and it must be registered
* as a new version.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Constrain the following parameters by using a
*
Condition
element with the appropriate keys.
*
* -
defaultTaskList
: String constraint. The key is
* swf:defaultTaskList.name
.
* -
name
: String constraint. The key is
* swf:name
.
* -
version
: String constraint. The key is
* swf:version
.
*
*
*
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param registerActivityTypeRequest Container for the necessary
* parameters to execute the RegisterActivityType operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RegisterActivityType service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future registerActivityTypeAsync(RegisterActivityTypeRequest registerActivityTypeRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of closed workflow executions in the specified domain
* that meet the filtering criteria. The results may be split into
* multiple pages. To retrieve subsequent pages, make the call again
* using the nextPageToken returned by the initial call.
*
*
* NOTE: This operation is eventually consistent. The results are
* best effort and may not exactly reflect recent updates and changes.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Constrain the following parameters by using a
*
Condition
element with the appropriate keys.
*
* -
tag
: String constraint. The key is
* swf:tagFilter.tag
.
* -
typeFilter.name
: String constraint. String
* constraint. The key is swf:typeFilter.name
.
* -
typeFilter.version
: String constraint. String
* constraint. The key is swf:typeFilter.version
.
*
*
*
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param listClosedWorkflowExecutionsRequest Container for the necessary
* parameters to execute the ListClosedWorkflowExecutions operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* ListClosedWorkflowExecutions service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listClosedWorkflowExecutionsAsync(ListClosedWorkflowExecutionsRequest listClosedWorkflowExecutionsRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns a list of closed workflow executions in the specified domain
* that meet the filtering criteria. The results may be split into
* multiple pages. To retrieve subsequent pages, make the call again
* using the nextPageToken returned by the initial call.
*
*
* NOTE: This operation is eventually consistent. The results are
* best effort and may not exactly reflect recent updates and changes.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Constrain the following parameters by using a
*
Condition
element with the appropriate keys.
*
* -
tag
: String constraint. The key is
* swf:tagFilter.tag
.
* -
typeFilter.name
: String constraint. String
* constraint. The key is swf:typeFilter.name
.
* -
typeFilter.version
: String constraint. String
* constraint. The key is swf:typeFilter.version
.
*
*
*
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param listClosedWorkflowExecutionsRequest Container for the necessary
* parameters to execute the ListClosedWorkflowExecutions operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListClosedWorkflowExecutions service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listClosedWorkflowExecutionsAsync(ListClosedWorkflowExecutionsRequest listClosedWorkflowExecutionsRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Used by activity workers to report to the service that the
* ActivityTask represented by the specified taskToken
is
* still making progress. The worker can also (optionally) specify
* details of the progress, for example percent complete, using the
* details
parameter. This action can also be used by the
* worker as a mechanism to check if cancellation is being requested for
* the activity task. If a cancellation is being attempted for the
* specified task, then the boolean cancelRequested
flag
* returned by the service is set to true
.
*
*
*
* This action resets the taskHeartbeatTimeout
clock. The
* taskHeartbeatTimeout
is specified in
* RegisterActivityType.
*
*
* This action does not in itself create an event in the workflow
* execution history. However, if the task times out, the workflow
* execution history will contain a ActivityTaskTimedOut
* event that contains the information from the last heartbeat generated
* by the activity worker.
*
*
* NOTE: The taskStartToCloseTimeout of an activity type is the
* maximum duration of an activity task, regardless of the number of
* RecordActivityTaskHeartbeat requests received. The
* taskStartToCloseTimeout is also specified in RegisterActivityType.
*
*
* NOTE: This operation is only useful for long-lived activities
* to report liveliness of the task and to determine if a cancellation is
* being attempted.
*
*
* IMPORTANT: If the cancelRequested flag returns true, a
* cancellation is being attempted. If the worker can cancel the
* activity, it should respond with RespondActivityTaskCanceled.
* Otherwise, it should ignore the cancellation request.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param recordActivityTaskHeartbeatRequest Container for the necessary
* parameters to execute the RecordActivityTaskHeartbeat operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* RecordActivityTaskHeartbeat service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future recordActivityTaskHeartbeatAsync(RecordActivityTaskHeartbeatRequest recordActivityTaskHeartbeatRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Used by activity workers to report to the service that the
* ActivityTask represented by the specified taskToken
is
* still making progress. The worker can also (optionally) specify
* details of the progress, for example percent complete, using the
* details
parameter. This action can also be used by the
* worker as a mechanism to check if cancellation is being requested for
* the activity task. If a cancellation is being attempted for the
* specified task, then the boolean cancelRequested
flag
* returned by the service is set to true
.
*
*
*
* This action resets the taskHeartbeatTimeout
clock. The
* taskHeartbeatTimeout
is specified in
* RegisterActivityType.
*
*
* This action does not in itself create an event in the workflow
* execution history. However, if the task times out, the workflow
* execution history will contain a ActivityTaskTimedOut
* event that contains the information from the last heartbeat generated
* by the activity worker.
*
*
* NOTE: The taskStartToCloseTimeout of an activity type is the
* maximum duration of an activity task, regardless of the number of
* RecordActivityTaskHeartbeat requests received. The
* taskStartToCloseTimeout is also specified in RegisterActivityType.
*
*
* NOTE: This operation is only useful for long-lived activities
* to report liveliness of the task and to determine if a cancellation is
* being attempted.
*
*
* IMPORTANT: If the cancelRequested flag returns true, a
* cancellation is being attempted. If the worker can cancel the
* activity, it should respond with RespondActivityTaskCanceled.
* Otherwise, it should ignore the cancellation request.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param recordActivityTaskHeartbeatRequest Container for the necessary
* parameters to execute the RecordActivityTaskHeartbeat operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RecordActivityTaskHeartbeat service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future recordActivityTaskHeartbeatAsync(RecordActivityTaskHeartbeatRequest recordActivityTaskHeartbeatRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Used by deciders to get a DecisionTask from the specified decision
* taskList
.
* A decision task may be returned for any open workflow execution
* that is using the specified task list. The task includes a paginated
* view of the history of the workflow execution. The decider should use
* the workflow type and the history to determine how to properly handle
* the task.
*
*
* This action initiates a long poll, where the service holds the HTTP
* connection open and responds as soon a task becomes available. If no
* decision task is available in the specified task list before the
* timeout of 60 seconds expires, an empty result is returned. An empty
* result, in this context, means that a DecisionTask is returned, but
* that the value of taskToken is an empty string.
*
*
* IMPORTANT: Deciders should set their client side socket timeout
* to at least 70 seconds (10 seconds higher than the timeout).
*
*
* IMPORTANT: Because the number of workflow history events for a
* single workflow execution might be very large, the result returned
* might be split up across a number of pages. To retrieve subsequent
* pages, make additional calls to PollForDecisionTask using the
* nextPageToken returned by the initial call. Note that you do not call
* GetWorkflowExecutionHistory with this nextPageToken. Instead, call
* PollForDecisionTask again.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Use a Condition element with the
*
swf:taskList.name
key to allow the action to access only
* certain task lists.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param pollForDecisionTaskRequest Container for the necessary
* parameters to execute the PollForDecisionTask operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* PollForDecisionTask service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future pollForDecisionTaskAsync(PollForDecisionTaskRequest pollForDecisionTaskRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Used by deciders to get a DecisionTask from the specified decision
* taskList
.
* A decision task may be returned for any open workflow execution
* that is using the specified task list. The task includes a paginated
* view of the history of the workflow execution. The decider should use
* the workflow type and the history to determine how to properly handle
* the task.
*
*
* This action initiates a long poll, where the service holds the HTTP
* connection open and responds as soon a task becomes available. If no
* decision task is available in the specified task list before the
* timeout of 60 seconds expires, an empty result is returned. An empty
* result, in this context, means that a DecisionTask is returned, but
* that the value of taskToken is an empty string.
*
*
* IMPORTANT: Deciders should set their client side socket timeout
* to at least 70 seconds (10 seconds higher than the timeout).
*
*
* IMPORTANT: Because the number of workflow history events for a
* single workflow execution might be very large, the result returned
* might be split up across a number of pages. To retrieve subsequent
* pages, make additional calls to PollForDecisionTask using the
* nextPageToken returned by the initial call. Note that you do not call
* GetWorkflowExecutionHistory with this nextPageToken. Instead, call
* PollForDecisionTask again.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Use a Condition element with the
*
swf:taskList.name
key to allow the action to access only
* certain task lists.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param pollForDecisionTaskRequest Container for the necessary
* parameters to execute the PollForDecisionTask operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* PollForDecisionTask service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future pollForDecisionTaskAsync(PollForDecisionTaskRequest pollForDecisionTaskRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about all activities registered in the specified
* domain that match the specified name and registration status. The
* result includes information like creation date, current status of the
* activity, etc. The results may be split into multiple pages. To
* retrieve subsequent pages, make the call again using the
* nextPageToken
returned by the initial call.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param listActivityTypesRequest Container for the necessary parameters
* to execute the ListActivityTypes operation on AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* ListActivityTypes service method, as returned by AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listActivityTypesAsync(ListActivityTypesRequest listActivityTypesRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about all activities registered in the specified
* domain that match the specified name and registration status. The
* result includes information like creation date, current status of the
* activity, etc. The results may be split into multiple pages. To
* retrieve subsequent pages, make the call again using the
* nextPageToken
returned by the initial call.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param listActivityTypesRequest Container for the necessary parameters
* to execute the ListActivityTypes operation on AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* ListActivityTypes service method, as returned by AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future listActivityTypesAsync(ListActivityTypesRequest listActivityTypesRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about the specified domain including description
* and status.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param describeDomainRequest Container for the necessary parameters to
* execute the DescribeDomain operation on AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* DescribeDomain service method, as returned by AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeDomainAsync(DescribeDomainRequest describeDomainRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about the specified domain including description
* and status.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param describeDomainRequest Container for the necessary parameters to
* execute the DescribeDomain operation on AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeDomain service method, as returned by AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeDomainAsync(DescribeDomainRequest describeDomainRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Used by workers to tell the service that the ActivityTask identified
* by the taskToken
has failed with reason
(if
* specified). The reason
and details
appear in
* the ActivityTaskFailed
event added to the workflow
* history.
*
*
* A task is considered open from the time that it is scheduled until it
* is closed. Therefore a task is reported as open while a worker is
* processing it. A task is closed after it has been specified in a call
* to RespondActivityTaskCompleted, RespondActivityTaskCanceled,
* RespondActivityTaskFailed, or the task has
* timed out
* .
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param respondActivityTaskFailedRequest Container for the necessary
* parameters to execute the RespondActivityTaskFailed operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* RespondActivityTaskFailed service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future respondActivityTaskFailedAsync(RespondActivityTaskFailedRequest respondActivityTaskFailedRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Used by workers to tell the service that the ActivityTask identified
* by the taskToken
has failed with reason
(if
* specified). The reason
and details
appear in
* the ActivityTaskFailed
event added to the workflow
* history.
*
*
* A task is considered open from the time that it is scheduled until it
* is closed. Therefore a task is reported as open while a worker is
* processing it. A task is closed after it has been specified in a call
* to RespondActivityTaskCompleted, RespondActivityTaskCanceled,
* RespondActivityTaskFailed, or the task has
* timed out
* .
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param respondActivityTaskFailedRequest Container for the necessary
* parameters to execute the RespondActivityTaskFailed operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* RespondActivityTaskFailed service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future respondActivityTaskFailedAsync(RespondActivityTaskFailedRequest respondActivityTaskFailedRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the estimated number of decision tasks in the specified task
* list. The count returned is an approximation and is not guaranteed to
* be exact. If you specify a task list that no decision task was ever
* scheduled in then 0 will be returned.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Use a Condition element with the
*
swf:taskList.name
key to allow the action to access only
* certain task lists.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param countPendingDecisionTasksRequest Container for the necessary
* parameters to execute the CountPendingDecisionTasks operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* CountPendingDecisionTasks service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future countPendingDecisionTasksAsync(CountPendingDecisionTasksRequest countPendingDecisionTasksRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns the estimated number of decision tasks in the specified task
* list. The count returned is an approximation and is not guaranteed to
* be exact. If you specify a task list that no decision task was ever
* scheduled in then 0 will be returned.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - Use a Condition element with the
*
swf:taskList.name
key to allow the action to access only
* certain task lists.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param countPendingDecisionTasksRequest Container for the necessary
* parameters to execute the CountPendingDecisionTasks operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* CountPendingDecisionTasks service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future countPendingDecisionTasksAsync(CountPendingDecisionTasksRequest countPendingDecisionTasksRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Records a WorkflowExecutionTerminated
event and forces
* closure of the workflow execution identified by the given domain,
* runId, and workflowId. The child policy, registered with the workflow
* type or specified when starting this execution, is applied to any open
* child workflow executions of this workflow execution.
*
*
* IMPORTANT: If the identified workflow execution was in
* progress, it is terminated immediately.
*
*
* NOTE: If a runId is not specified, then the
* WorkflowExecutionTerminated event is recorded in the history of the
* current open workflow with the matching workflowId in the domain.
*
*
* NOTE: You should consider using RequestCancelWorkflowExecution
* action instead because it allows the workflow to gracefully close
* while TerminateWorkflowExecution does not.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param terminateWorkflowExecutionRequest Container for the necessary
* parameters to execute the TerminateWorkflowExecution operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* TerminateWorkflowExecution service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future terminateWorkflowExecutionAsync(TerminateWorkflowExecutionRequest terminateWorkflowExecutionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Records a WorkflowExecutionTerminated
event and forces
* closure of the workflow execution identified by the given domain,
* runId, and workflowId. The child policy, registered with the workflow
* type or specified when starting this execution, is applied to any open
* child workflow executions of this workflow execution.
*
*
* IMPORTANT: If the identified workflow execution was in
* progress, it is terminated immediately.
*
*
* NOTE: If a runId is not specified, then the
* WorkflowExecutionTerminated event is recorded in the history of the
* current open workflow with the matching workflowId in the domain.
*
*
* NOTE: You should consider using RequestCancelWorkflowExecution
* action instead because it allows the workflow to gracefully close
* while TerminateWorkflowExecution does not.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param terminateWorkflowExecutionRequest Container for the necessary
* parameters to execute the TerminateWorkflowExecution operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* TerminateWorkflowExecution service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future terminateWorkflowExecutionAsync(TerminateWorkflowExecutionRequest terminateWorkflowExecutionRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about the specified workflow execution including
* its type and some statistics.
*
*
* NOTE: This operation is eventually consistent. The results are
* best effort and may not exactly reflect recent updates and changes.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param describeWorkflowExecutionRequest Container for the necessary
* parameters to execute the DescribeWorkflowExecution operation on
* AmazonSimpleWorkflow.
*
* @return A Java Future object containing the response from the
* DescribeWorkflowExecution service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeWorkflowExecutionAsync(DescribeWorkflowExecutionRequest describeWorkflowExecutionRequest)
throws AmazonServiceException, AmazonClientException;
/**
*
* Returns information about the specified workflow execution including
* its type and some statistics.
*
*
* NOTE: This operation is eventually consistent. The results are
* best effort and may not exactly reflect recent updates and changes.
*
*
* Access Control
*
*
* You can use IAM policies to control this action's access to Amazon SWF
* resources as follows:
*
*
*
* - Use a
Resource
element with the domain name to limit
* the action to only specified domains.
* - Use an
Action
element to allow or deny permission to
* call this action.
* - You cannot use an IAM policy to constrain this action's
* parameters.
*
*
*
* If the caller does not have sufficient permissions to invoke the
* action, or the parameter values fall outside the specified
* constraints, the action fails by throwing
* OperationNotPermitted
. For details and example IAM
* policies, see
* Using IAM to Manage Access to Amazon SWF Workflows
* .
*
*
* @param describeWorkflowExecutionRequest Container for the necessary
* parameters to execute the DescribeWorkflowExecution operation on
* AmazonSimpleWorkflow.
* @param asyncHandler Asynchronous callback handler for events in the
* life-cycle of the request. Users could provide the implementation of
* the four callback methods in this interface to process the operation
* result or handle the exception.
*
* @return A Java Future object containing the response from the
* DescribeWorkflowExecution service method, as returned by
* AmazonSimpleWorkflow.
*
*
* @throws AmazonClientException
* If any internal errors are encountered inside the client while
* attempting to make the request or handle the response. For example
* if a network connection is not available.
* @throws AmazonServiceException
* If an error response is returned by AmazonSimpleWorkflow indicating
* either a problem with the data in the request, or a server side issue.
*/
public Future describeWorkflowExecutionAsync(DescribeWorkflowExecutionRequest describeWorkflowExecutionRequest,
AsyncHandler asyncHandler)
throws AmazonServiceException, AmazonClientException;
}