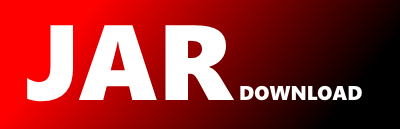
com.amazonaws.services.simpleworkflow.flow.DynamicWorkflowClientImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-java-sdk Show documentation
Show all versions of aws-java-sdk Show documentation
The Amazon Web Services SDK for Java provides Java APIs for building software on AWS' cost-effective, scalable, and reliable infrastructure products. The AWS Java SDK allows developers to code against APIs for all of Amazon's infrastructure web services (Amazon S3, Amazon EC2, Amazon SQS, Amazon Relational Database Service, Amazon AutoScaling, etc).
The newest version!
/*
* Copyright 2012-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not
* use this file except in compliance with the License. A copy of the License is
* located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.simpleworkflow.flow;
import com.amazonaws.services.simpleworkflow.flow.core.Functor;
import com.amazonaws.services.simpleworkflow.flow.core.Promise;
import com.amazonaws.services.simpleworkflow.flow.core.Settable;
import com.amazonaws.services.simpleworkflow.flow.core.Task;
import com.amazonaws.services.simpleworkflow.flow.core.TryFinally;
import com.amazonaws.services.simpleworkflow.flow.generic.GenericWorkflowClient;
import com.amazonaws.services.simpleworkflow.flow.generic.SignalExternalWorkflowParameters;
import com.amazonaws.services.simpleworkflow.flow.generic.StartChildWorkflowExecutionParameters;
import com.amazonaws.services.simpleworkflow.flow.generic.StartChildWorkflowReply;
import com.amazonaws.services.simpleworkflow.model.WorkflowExecution;
import com.amazonaws.services.simpleworkflow.model.WorkflowType;
public class DynamicWorkflowClientImpl implements DynamicWorkflowClient {
protected WorkflowType workflowType;
protected GenericWorkflowClient genericClient;
protected StartWorkflowOptions schedulingOptions;
protected DataConverter dataConverter;
protected WorkflowExecution workflowExecution;
protected DecisionContextProvider decisionContextProvider = new DecisionContextProviderImpl();
protected Settable runId = new Settable();
public DynamicWorkflowClientImpl() {
this(null, null, null, null, null);
}
public DynamicWorkflowClientImpl(WorkflowExecution workflowExecution) {
this(workflowExecution, null, null, null, null);
}
public DynamicWorkflowClientImpl(WorkflowExecution workflowExecution, WorkflowType workflowType) {
this(workflowExecution, workflowType, null, null, null);
}
public DynamicWorkflowClientImpl(WorkflowExecution workflowExecution, WorkflowType workflowType, StartWorkflowOptions options) {
this(workflowExecution, workflowType, options, null, null);
}
public DynamicWorkflowClientImpl(WorkflowExecution workflowExecution, WorkflowType workflowType,
StartWorkflowOptions options, DataConverter dataConverter) {
this(workflowExecution, workflowType, options, dataConverter, null);
}
public DynamicWorkflowClientImpl(WorkflowExecution workflowExecution, WorkflowType workflowType,
StartWorkflowOptions options, DataConverter dataConverter, GenericWorkflowClient genericClient) {
this.workflowType = workflowType;
this.workflowExecution = workflowExecution;
if (dataConverter == null) {
this.dataConverter = new JsonDataConverter();
}
else {
this.dataConverter = dataConverter;
}
this.schedulingOptions = options;
this.genericClient = genericClient;
if (workflowExecution.getRunId() != null) {
this.runId.set(workflowExecution.getRunId());
}
}
@Override
public DataConverter getDataConverter() {
return dataConverter;
}
@Override
public StartWorkflowOptions getSchedulingOptions() {
return schedulingOptions;
}
@Override
public GenericWorkflowClient getGenericClient() {
return genericClient;
}
@Override
public Promise getRunId() {
return runId;
}
@Override
public WorkflowExecution getWorkflowExecution() {
return workflowExecution;
}
@Override
public WorkflowType getWorkflowType() {
return workflowType;
}
public void setWorkflowType(WorkflowType workflowType) {
this.workflowType = workflowType;
}
public void setGenericClient(GenericWorkflowClient genericClient) {
this.genericClient = genericClient;
}
public void setSchedulingOptions(StartWorkflowOptions schedulingOptions) {
this.schedulingOptions = schedulingOptions;
}
public void setDataConverter(DataConverter dataConverter) {
this.dataConverter = dataConverter;
}
@Override
public void requestCancelWorkflowExecution(Promise>... waitFor) {
checkWorkflowExecution();
new Task(waitFor) {
@Override
protected void doExecute() throws Throwable {
GenericWorkflowClient client = getGenericClientToUse();
client.requestCancelWorkflowExecution(workflowExecution);
}
};
}
private void checkWorkflowExecution() {
if (workflowExecution == null) {
throw new IllegalStateException("required property workflowExecution is null");
}
}
public Promise startWorkflowExecution(final Promise
© 2015 - 2025 Weber Informatics LLC | Privacy Policy