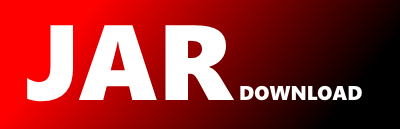
com.amazonaws.services.simpleworkflow.flow.junit.spring.FlowSpringJUnit4ClassRunner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-java-sdk Show documentation
Show all versions of aws-java-sdk Show documentation
The Amazon Web Services SDK for Java provides Java APIs for building software on AWS' cost-effective, scalable, and reliable infrastructure products. The AWS Java SDK allows developers to code against APIs for all of Amazon's infrastructure web services (Amazon S3, Amazon EC2, Amazon SQS, Amazon Relational Database Service, Amazon AutoScaling, etc).
The newest version!
package com.amazonaws.services.simpleworkflow.flow.junit.spring;
import java.util.List;
import org.junit.Test;
import org.junit.rules.MethodRule;
import org.junit.runners.model.FrameworkMethod;
import org.junit.runners.model.InitializationError;
import org.junit.runners.model.Statement;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import com.amazonaws.services.simpleworkflow.flow.junit.WorkflowTestBase;
/**
* To be used instead of {@link SpringJUnit4ClassRunner} when testing
* asynchronous code. Requires {@link SpringWorkflowTest} rule (annotated with
* @Rule) to be present in the tested class.
*
* @author fateev
*/
public class FlowSpringJUnit4ClassRunner extends SpringJUnit4ClassRunner {
private WorkflowTestBase workflowTestRule;
private long timeout;
private Class extends Throwable> expectedException;
public FlowSpringJUnit4ClassRunner(Class> clazz) throws InitializationError {
super(clazz);
}
@Override
protected Statement withPotentialTimeout(final FrameworkMethod method, final Object test, Statement next) {
Test annotation = method.getAnnotation(Test.class);
long timeout = annotation.timeout();
if (timeout > 0) {
long springTimeout = getSpringTimeout(method);
if (workflowTestRule != null && springTimeout == 0) {
workflowTestRule.setTestTimeoutActualTimeMilliseconds(timeout);
}
}
return next;
}
@Override
protected List rules(Object test) {
List result = super.rules(test);
for (MethodRule methodRule : result) {
if (WorkflowTestBase.class.isAssignableFrom(methodRule.getClass())) {
workflowTestRule = (WorkflowTestBase) methodRule;
workflowTestRule.setFlowTestRunner(true);
if (timeout > 0) {
workflowTestRule.setTestTimeoutActualTimeMilliseconds(timeout);
}
if (expectedException != null) {
workflowTestRule.setExpectedException(expectedException);
}
}
}
return result;
}
@Override
protected Statement possiblyExpectingExceptions(FrameworkMethod method, Object test, Statement next) {
Test annotation = method.getAnnotation(Test.class);
Class extends Throwable> expected = annotation.expected();
if (expected != Test.None.class) {
expectedException = expected;
if (workflowTestRule != null) {
workflowTestRule.setExpectedException(expectedException);
}
}
return next;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy