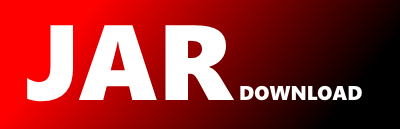
com.amazonaws.services.storagegateway.model.CreateTapesRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk Show documentation
/*
* Copyright 2010-2014 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.storagegateway.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
* Container for the parameters to the {@link com.amazonaws.services.storagegateway.AWSStorageGateway#createTapes(CreateTapesRequest) CreateTapes operation}.
*
* Creates one or more virtual tapes. You write data to the virtual tapes
* and then archive the tapes.
*
*
* NOTE:Cache storage must be allocated to the gateway before you
* can create virtual tapes. Use the AddCache operation to add cache
* storage to a gateway.
*
*
* @see com.amazonaws.services.storagegateway.AWSStorageGateway#createTapes(CreateTapesRequest)
*/
public class CreateTapesRequest extends AmazonWebServiceRequest implements Serializable {
/**
* The unique Amazon Resource Name(ARN) that represents the gateway to
* associate the virtual tapes with. Use the ListGateways
* operation to return a list of gateways for your account and region.
*
* Constraints:
* Length: 50 - 500
*/
private String gatewayARN;
/**
* The size, in bytes, of the virtual tapes you want to create. The
* size must be gigabyte (1024*1024*1024 byte) aligned.
*
* Constraints:
* Range: 107374182400 - 2748779069440
*/
private Long tapeSizeInBytes;
/**
* A unique identifier that you use to retry a request. If you retry a
* request, use the same ClientToken
you specified in the
* initial request. Using the same ClientToken
* prevents creating the tape multiple times.
*
* Constraints:
* Length: 5 - 100
*/
private String clientToken;
/**
* The number of virtual tapes you want to create.
*
* Constraints:
* Range: 1 - 10
*/
private Integer numTapesToCreate;
/**
* A prefix you append to the barcode of the virtual tape you are
* creating. This makes a barcode unique. The prefix must be 1 to 4
* characters in length and must be upper-case letters A-Z.
*
* Constraints:
* Length: 1 - 4
* Pattern: ^[A-Z]*$
*/
private String tapeBarcodePrefix;
/**
* The unique Amazon Resource Name(ARN) that represents the gateway to
* associate the virtual tapes with. Use the ListGateways
* operation to return a list of gateways for your account and region.
*
* Constraints:
* Length: 50 - 500
*
* @return The unique Amazon Resource Name(ARN) that represents the gateway to
* associate the virtual tapes with. Use the ListGateways
* operation to return a list of gateways for your account and region.
*/
public String getGatewayARN() {
return gatewayARN;
}
/**
* The unique Amazon Resource Name(ARN) that represents the gateway to
* associate the virtual tapes with. Use the ListGateways
* operation to return a list of gateways for your account and region.
*
* Constraints:
* Length: 50 - 500
*
* @param gatewayARN The unique Amazon Resource Name(ARN) that represents the gateway to
* associate the virtual tapes with. Use the ListGateways
* operation to return a list of gateways for your account and region.
*/
public void setGatewayARN(String gatewayARN) {
this.gatewayARN = gatewayARN;
}
/**
* The unique Amazon Resource Name(ARN) that represents the gateway to
* associate the virtual tapes with. Use the ListGateways
* operation to return a list of gateways for your account and region.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 50 - 500
*
* @param gatewayARN The unique Amazon Resource Name(ARN) that represents the gateway to
* associate the virtual tapes with. Use the ListGateways
* operation to return a list of gateways for your account and region.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateTapesRequest withGatewayARN(String gatewayARN) {
this.gatewayARN = gatewayARN;
return this;
}
/**
* The size, in bytes, of the virtual tapes you want to create. The
* size must be gigabyte (1024*1024*1024 byte) aligned.
*
* Constraints:
* Range: 107374182400 - 2748779069440
*
* @return The size, in bytes, of the virtual tapes you want to create. The
* size must be gigabyte (1024*1024*1024 byte) aligned.
*/
public Long getTapeSizeInBytes() {
return tapeSizeInBytes;
}
/**
* The size, in bytes, of the virtual tapes you want to create. The
* size must be gigabyte (1024*1024*1024 byte) aligned.
*
* Constraints:
* Range: 107374182400 - 2748779069440
*
* @param tapeSizeInBytes The size, in bytes, of the virtual tapes you want to create. The
* size must be gigabyte (1024*1024*1024 byte) aligned.
*/
public void setTapeSizeInBytes(Long tapeSizeInBytes) {
this.tapeSizeInBytes = tapeSizeInBytes;
}
/**
* The size, in bytes, of the virtual tapes you want to create. The
* size must be gigabyte (1024*1024*1024 byte) aligned.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Range: 107374182400 - 2748779069440
*
* @param tapeSizeInBytes The size, in bytes, of the virtual tapes you want to create. The
* size must be gigabyte (1024*1024*1024 byte) aligned.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateTapesRequest withTapeSizeInBytes(Long tapeSizeInBytes) {
this.tapeSizeInBytes = tapeSizeInBytes;
return this;
}
/**
* A unique identifier that you use to retry a request. If you retry a
* request, use the same ClientToken
you specified in the
* initial request. Using the same ClientToken
* prevents creating the tape multiple times.
*
* Constraints:
* Length: 5 - 100
*
* @return A unique identifier that you use to retry a request. If you retry a
* request, use the same ClientToken
you specified in the
* initial request. Using the same ClientToken
* prevents creating the tape multiple times.
*/
public String getClientToken() {
return clientToken;
}
/**
* A unique identifier that you use to retry a request. If you retry a
* request, use the same ClientToken
you specified in the
* initial request. Using the same ClientToken
* prevents creating the tape multiple times.
*
* Constraints:
* Length: 5 - 100
*
* @param clientToken A unique identifier that you use to retry a request. If you retry a
* request, use the same ClientToken
you specified in the
* initial request. Using the same ClientToken
* prevents creating the tape multiple times.
*/
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
/**
* A unique identifier that you use to retry a request. If you retry a
* request, use the same ClientToken
you specified in the
* initial request. Using the same ClientToken
* prevents creating the tape multiple times.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 5 - 100
*
* @param clientToken A unique identifier that you use to retry a request. If you retry a
* request, use the same ClientToken
you specified in the
* initial request. Using the same ClientToken
* prevents creating the tape multiple times.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateTapesRequest withClientToken(String clientToken) {
this.clientToken = clientToken;
return this;
}
/**
* The number of virtual tapes you want to create.
*
* Constraints:
* Range: 1 - 10
*
* @return The number of virtual tapes you want to create.
*/
public Integer getNumTapesToCreate() {
return numTapesToCreate;
}
/**
* The number of virtual tapes you want to create.
*
* Constraints:
* Range: 1 - 10
*
* @param numTapesToCreate The number of virtual tapes you want to create.
*/
public void setNumTapesToCreate(Integer numTapesToCreate) {
this.numTapesToCreate = numTapesToCreate;
}
/**
* The number of virtual tapes you want to create.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Range: 1 - 10
*
* @param numTapesToCreate The number of virtual tapes you want to create.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateTapesRequest withNumTapesToCreate(Integer numTapesToCreate) {
this.numTapesToCreate = numTapesToCreate;
return this;
}
/**
* A prefix you append to the barcode of the virtual tape you are
* creating. This makes a barcode unique. The prefix must be 1 to 4
* characters in length and must be upper-case letters A-Z.
*
* Constraints:
* Length: 1 - 4
* Pattern: ^[A-Z]*$
*
* @return A prefix you append to the barcode of the virtual tape you are
* creating. This makes a barcode unique. The prefix must be 1 to 4
* characters in length and must be upper-case letters A-Z.
*/
public String getTapeBarcodePrefix() {
return tapeBarcodePrefix;
}
/**
* A prefix you append to the barcode of the virtual tape you are
* creating. This makes a barcode unique. The prefix must be 1 to 4
* characters in length and must be upper-case letters A-Z.
*
* Constraints:
* Length: 1 - 4
* Pattern: ^[A-Z]*$
*
* @param tapeBarcodePrefix A prefix you append to the barcode of the virtual tape you are
* creating. This makes a barcode unique. The prefix must be 1 to 4
* characters in length and must be upper-case letters A-Z.
*/
public void setTapeBarcodePrefix(String tapeBarcodePrefix) {
this.tapeBarcodePrefix = tapeBarcodePrefix;
}
/**
* A prefix you append to the barcode of the virtual tape you are
* creating. This makes a barcode unique. The prefix must be 1 to 4
* characters in length and must be upper-case letters A-Z.
*
* Returns a reference to this object so that method calls can be chained together.
*
* Constraints:
* Length: 1 - 4
* Pattern: ^[A-Z]*$
*
* @param tapeBarcodePrefix A prefix you append to the barcode of the virtual tape you are
* creating. This makes a barcode unique. The prefix must be 1 to 4
* characters in length and must be upper-case letters A-Z.
*
* @return A reference to this updated object so that method calls can be chained
* together.
*/
public CreateTapesRequest withTapeBarcodePrefix(String tapeBarcodePrefix) {
this.tapeBarcodePrefix = tapeBarcodePrefix;
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getGatewayARN() != null) sb.append("GatewayARN: " + getGatewayARN() + ",");
if (getTapeSizeInBytes() != null) sb.append("TapeSizeInBytes: " + getTapeSizeInBytes() + ",");
if (getClientToken() != null) sb.append("ClientToken: " + getClientToken() + ",");
if (getNumTapesToCreate() != null) sb.append("NumTapesToCreate: " + getNumTapesToCreate() + ",");
if (getTapeBarcodePrefix() != null) sb.append("TapeBarcodePrefix: " + getTapeBarcodePrefix() );
sb.append("}");
return sb.toString();
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getGatewayARN() == null) ? 0 : getGatewayARN().hashCode());
hashCode = prime * hashCode + ((getTapeSizeInBytes() == null) ? 0 : getTapeSizeInBytes().hashCode());
hashCode = prime * hashCode + ((getClientToken() == null) ? 0 : getClientToken().hashCode());
hashCode = prime * hashCode + ((getNumTapesToCreate() == null) ? 0 : getNumTapesToCreate().hashCode());
hashCode = prime * hashCode + ((getTapeBarcodePrefix() == null) ? 0 : getTapeBarcodePrefix().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null) return false;
if (obj instanceof CreateTapesRequest == false) return false;
CreateTapesRequest other = (CreateTapesRequest)obj;
if (other.getGatewayARN() == null ^ this.getGatewayARN() == null) return false;
if (other.getGatewayARN() != null && other.getGatewayARN().equals(this.getGatewayARN()) == false) return false;
if (other.getTapeSizeInBytes() == null ^ this.getTapeSizeInBytes() == null) return false;
if (other.getTapeSizeInBytes() != null && other.getTapeSizeInBytes().equals(this.getTapeSizeInBytes()) == false) return false;
if (other.getClientToken() == null ^ this.getClientToken() == null) return false;
if (other.getClientToken() != null && other.getClientToken().equals(this.getClientToken()) == false) return false;
if (other.getNumTapesToCreate() == null ^ this.getNumTapesToCreate() == null) return false;
if (other.getNumTapesToCreate() != null && other.getNumTapesToCreate().equals(this.getNumTapesToCreate()) == false) return false;
if (other.getTapeBarcodePrefix() == null ^ this.getTapeBarcodePrefix() == null) return false;
if (other.getTapeBarcodePrefix() != null && other.getTapeBarcodePrefix().equals(this.getTapeBarcodePrefix()) == false) return false;
return true;
}
}