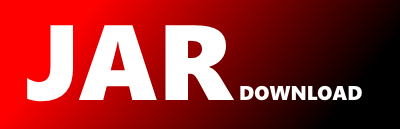
com.emc.storageos.model.adapters.StringMapAdapter Maven / Gradle / Ivy
/*
* Copyright (c) 2008-2011 EMC Corporation
* All Rights Reserved
*/
package com.emc.storageos.model.adapters;
import com.emc.storageos.model.StringHashMapEntry;
import javax.xml.bind.annotation.adapters.XmlAdapter;
import java.util.HashMap;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
/**
* An JAXB XML adapter from Map into List
*
* JAXB automatically converts a Map into a list to marshal it into an XML file.
* The elements of a HashMap are "entry" pairs of key/value. If we want to replace the
* HashMap elements with names "entry", "key", "value" into elements consistent with our
* definition of REST guidelines, we need to translate Maps into Lists ourselves.
* This class provide an adapter from Map> into List
* This class should be used in conjuction with XML annotation "XmlJavaTypeAdapter"
*/
public class StringMapAdapter extends XmlAdapter, Map>
{
@Override
public Map unmarshal(List list) {
if (list == null) {
return (HashMap) null;
}
Map map = new HashMap(2 * list.size());
for (StringHashMapEntry entry : list) {
map.put(entry.getName(), entry.getValue());
}
return map;
}
@Override
public List marshal(Map map) {
if (map == null) {
return (ArrayList) null;
}
List list = new ArrayList();
if (map != null) {
for (Entry entry : map.entrySet()) {
StringHashMapEntry listEnt = new StringHashMapEntry(entry.getKey(), entry.getValue());
list.add(listEnt);
}
}
return list;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy