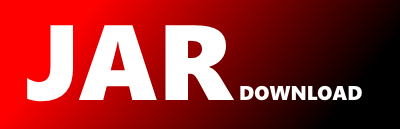
com.emc.storageos.model.adapters.StringSetMapAdapter Maven / Gradle / Ivy
/*
* Copyright (c) 2008-2013 EMC Corporation
* All Rights Reserved
*/
package com.emc.storageos.model.adapters;
import java.util.*;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.adapters.XmlAdapter;
public class StringSetMapAdapter extends XmlAdapter, Map>> {
public static class EntryList {
public List entryList = new ArrayList();
}
public static class Entry {
private String key;
private String value;
@XmlElement(name = "name")
public String getKey() {
return key;
}
public void setKey(String key) {
this.key = key;
}
@XmlElement(name = "value")
public String getValue() {
return value;
}
public void setValue(String value) {
this.value = value;
}
}
@Override
public List marshal(Map> map) throws Exception {
List entryList = new ArrayList();
for (Map.Entry> mapEntry : map.entrySet()) {
for (String value : mapEntry.getValue()) {
Entry entry = new Entry();
entry.setKey(mapEntry.getKey());
entry.setValue(value);
entryList.add(entry);
}
}
return entryList;
}
@Override
public Map> unmarshal(List entryList)
throws Exception {
Map> map = new HashMap>();
for (Entry entry : entryList) {
if (map.containsKey(entry.getKey())) {
map.get(entry.getKey()).add(entry.getValue());
} else {
Set valueSet = new HashSet();
valueSet.add(entry.getValue());
map.put(entry.getKey(), valueSet);
}
}
return map;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy