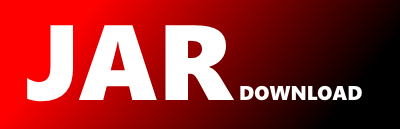
com.emc.storageos.model.block.UnManagedVolumeRestRep Maven / Gradle / Ivy
/*
* Copyright (c) 2008-2013 EMC Corporation
* All Rights Reserved
*/
package com.emc.storageos.model.block;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElementWrapper;
import javax.xml.bind.annotation.XmlRootElement;
import com.emc.storageos.model.DataObjectRestRep;
import com.emc.storageos.model.RelatedResourceRep;
import com.emc.storageos.model.StringHashMapEntry;
import com.emc.storageos.model.adapters.StringSetMapAdapter;
@XmlRootElement(name = "unmanaged_volume")
@XmlAccessorType(XmlAccessType.PROPERTY)
public class UnManagedVolumeRestRep extends DataObjectRestRep {
/**
* The native GUID of a discovered unmanaged volume which
* has not yet been ingested into ViPR.
*
* @valid none
*/
private String nativeGuid;
/**
* Information about the unmanaged volume. For example, allocated capacity,
* provisioned capacity, disk technology, and whether or not the volume is
* thinly provisioned.
*
* @valid none
*/
private List volumeInformation;
/**
* Characteristics of the unmanaged volume, such as is it mapped,
* is it a composite, and is it a snapshot.
*
* @valid none
*/
private List volumeCharacteristics;
/**
* List of UnManagedExportMasks associated with this UnManagedVolume.
*/
private List unManagedExportMasks;
/**
* List of initiator URIs associated with this UnManagedVolume.
*/
private List initiatorUris;
/**
* List of initiator network IDs associated with this UnManagedVolume.
*/
private List initiatorNetworkIds;
/**
* List of storage port URIs associated with this UnManagedVolume.
*/
private List storagePortUris;
/**
* List of supported VPool URIs associated with this UnManagedVolume.
*/
private List supportedVPoolUris;
/**
* The storage system to which this volume belongs.
*
* @valid none
*/
private RelatedResourceRep storageSystem;
/**
* The storage pool to which this volume belongs.
*
* @valid none
*/
private RelatedResourceRep storagePool;
/**
* WWN of the Volume
*
* @valid none
*/
private String wwn;
@XmlElement(name = "native_guid")
public String getNativeGuid() {
return nativeGuid;
}
public void setNativeGuid(String nativeGuid) {
this.nativeGuid = nativeGuid;
}
@XmlElement(name = "storage_system")
public RelatedResourceRep getStorageSystem() {
return storageSystem;
}
public void setStorageSystem(RelatedResourceRep storageSystem) {
this.storageSystem = storageSystem;
}
@XmlElement(name = "storage_pool")
public RelatedResourceRep getStoragePool() {
return storagePool;
}
public void setStoragePool(RelatedResourceRep storagePool) {
this.storagePool = storagePool;
}
@XmlElementWrapper(name = "unmanaged_volumes_characterstics")
@XmlElement(name = "unmanaged_volume_characterstic")
public List getVolumeCharacteristics() {
if (volumeCharacteristics == null) {
volumeCharacteristics = new ArrayList();
}
return volumeCharacteristics;
}
public void setVolumeCharacteristics(List volumeCharacteristics) {
this.volumeCharacteristics = volumeCharacteristics;
}
@XmlElementWrapper(name = "unmanaged_volumes_info")
@XmlElement(name = "unmanaged_volume_info")
public List getVolumeInformation() {
if (volumeInformation == null) {
volumeInformation = new ArrayList();
}
return volumeInformation;
}
public void setVolumeInformation(List volumeInformation) {
this.volumeInformation = volumeInformation;
}
@XmlElementWrapper(name = "unmanaged_export_masks")
@XmlElement(name = "unmanaged_export_mask")
public List getUnManagedExportMasks() {
if (unManagedExportMasks == null) {
unManagedExportMasks = new ArrayList();
}
return unManagedExportMasks;
}
public void setUnManagedExportMasks(List unManagedExportMasks) {
this.unManagedExportMasks = unManagedExportMasks;
}
@XmlElementWrapper(name = "initiators")
@XmlElement(name = "initiator")
public List getInitiatorUris() {
if (initiatorUris == null) {
initiatorUris = new ArrayList();
}
return initiatorUris;
}
public void setInitiatorUris(List initiatorUris) {
this.initiatorUris = initiatorUris;
}
@XmlElementWrapper(name = "initiator_network_ids")
@XmlElement(name = "initiator_network_id")
public List getInitiatorNetworkIds() {
if (initiatorNetworkIds == null) {
initiatorNetworkIds = new ArrayList();
}
return initiatorNetworkIds;
}
public void setInitiatorNetworkIds(List initiatorNetworkIds) {
this.initiatorNetworkIds = initiatorNetworkIds;
}
@XmlElementWrapper(name = "storage_ports")
@XmlElement(name = "storage_port")
public List getStoragePortUris() {
if (storagePortUris == null) {
storagePortUris = new ArrayList();
}
return storagePortUris;
}
public void setStoragePortUris(List storagePortUris) {
this.storagePortUris = storagePortUris;
}
@XmlElementWrapper(name = "supported_virtual_pools")
@XmlElement(name = "virtual_pool")
public List getSupportedVPoolUris() {
if (supportedVPoolUris == null) {
supportedVPoolUris = new ArrayList();
}
return supportedVPoolUris;
}
public void setSupportedVPoolUris(List supportedVPoolUris) {
this.supportedVPoolUris = supportedVPoolUris;
}
public void setWWN(String wwn) {
this.wwn = wwn;
}
@XmlElement(name = "wwn")
public String getWWN() {
return wwn;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy