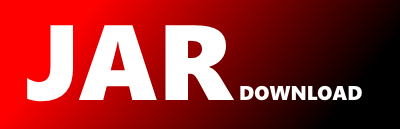
com.enterprisemath.dao.big.BigEntity Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of em-dao Show documentation
Show all versions of em-dao Show documentation
Simple and powerful data access layer.
package com.enterprisemath.dao.big;
import com.enterprisemath.utils.DomainUtils;
import com.enterprisemath.utils.ValidationUtils;
import java.util.HashMap;
import java.util.Map;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
/**
* Big entity object. This is the
*
* @author radek.hecl
*/
public class BigEntity {
/**
* Builder object.
*/
public static class Builder {
/**
* Unique identification code.
*/
public String code;
/**
* Fields.
*/
private Map fields = new HashMap();
/**
* Sets code of the entity. This is unique identifier.
*
* @param code code of the entity
* @return this instance
*/
public Builder setCode(String code) {
this.code = code;
return this;
}
/**
* Adds generic field.
*
* @param key key
* @param value value
* @return this instance
*/
public Builder addField(String key, Object value) {
fields.put(key, value);
return this;
}
/**
* Adds string field.
*
* @param key key
* @param value value
* @return this instance
*/
public Builder addStringField(String key, String value) {
fields.put(key, value);
return this;
}
/**
* Sets fields.
*
* @param fields fields
* @return this instance
*/
public Builder setFields(Map fields) {
this.fields = DomainUtils.softCopyMap(fields);
return this;
}
/**
* Builds the result object.
*
* @return create object
*/
public BigEntity build() {
return new BigEntity(this);
}
}
/**
* Unique identification code.
*/
public String code;
/**
* Fields.
*/
private Map fields;
/**
* Creates new instance.
*
* @param builder builder object
*/
public BigEntity(Builder builder) {
code = builder.code;
fields = DomainUtils.softCopyUnmodifiableMap(builder.fields);
guardInvariants();
}
/**
* Guards this object to be consistent. Throws exception if this is not the case.
*/
private void guardInvariants() {
ValidationUtils.guardNotEmpty(code, "code cannot be empty");
ValidationUtils.guardNotEmptyStringInCollection(fields.keySet(), "fields cannot have empty key");
}
/**
* Returns code of this entity. This is the unique identifier.
*
* @return code of this entity as a unique identifier
*/
public String getCode() {
return code;
}
/**
* Returns fields.
*
* @return fields
*/
public Map getFields() {
return fields;
}
/**
* Returns string fields.
*
* @param key key
* @return property value
*/
public String getStringField(String key) {
return (String) fields.get(key);
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
@Override
public boolean equals(Object obj) {
return EqualsBuilder.reflectionEquals(this, obj);
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy