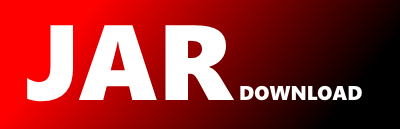
com.enterprisemath.dao.big.BigEntityUpdate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of em-dao Show documentation
Show all versions of em-dao Show documentation
Simple and powerful data access layer.
package com.enterprisemath.dao.big;
import com.enterprisemath.utils.DomainUtils;
import com.enterprisemath.utils.ValidationUtils;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
/**
* Defines update for the big entity.
*
* @author radek.hecl
*/
public class BigEntityUpdate {
/**
* Builder object.
*/
public static class Builder {
/**
* Fields which should be updated.
*/
public Map updateFields = new HashMap();
/**
* Fields which should be dropped.
*/
public Set dropFields = new HashSet();
/**
* Adds generic field to be updated.
*
* @param key key
* @param value value
* @return this instance
*/
public Builder addUpdateField(String key, Object value) {
updateFields.put(key, value);
return this;
}
/**
* Adds field to be updated as a string.
*
* @param key key
* @param value value
* @return this instance
*/
public Builder addStringUpdateField(String key, String value) {
updateFields.put(key, value);
return this;
}
/**
* Adds field to be dropped.
*
* @param key key
* @return this instance
*/
public Builder addDropField(String key) {
dropFields.add(key);
return this;
}
/**
* Builds the result object.
*
* @return create object
*/
public BigEntityUpdate build() {
return new BigEntityUpdate(this);
}
}
/**
* Fields which should be updated.
*/
public Map updateFields = new HashMap();
/**
* Fields which should be dropped.
*/
public Set dropFields = new HashSet();
/**
* Creates new instance.
*
* @param builder builder object
*/
public BigEntityUpdate(Builder builder) {
updateFields = DomainUtils.softCopyUnmodifiableMap(builder.updateFields);
dropFields = DomainUtils.softCopyUnmodifiableSet(builder.dropFields);
guardInvariants();
}
/**
* Guards this object to be consistent. Throws exception if this is not the case.
*/
private void guardInvariants() {
ValidationUtils.guardNotEmptyStringInCollection(updateFields.keySet(), "updateFields cannot have empty key");
ValidationUtils.guardNotEmptyStringInCollection(dropFields, "dropFields cannot have empty element");
Set pom = DomainUtils.softCopySet(updateFields.keySet());
pom.retainAll(dropFields);
ValidationUtils.guardEquals(0, pom.size(),
"field cannot be in both - update and drop keys: drop = " + dropFields + "; update = " + updateFields.keySet());
}
/**
* Returns fields for update.
*
* @return fields for update
*/
public Map getUpdateFields() {
return updateFields;
}
/**
* Returns fields for drop.
*
* @return fields for drop
*/
public Set getDropFields() {
return dropFields;
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
@Override
public boolean equals(Object obj) {
return EqualsBuilder.reflectionEquals(this, obj);
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy