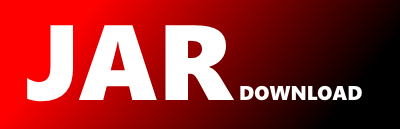
com.enterprisemath.dao.big.MyBatisBigEntityMapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of em-dao Show documentation
Show all versions of em-dao Show documentation
Simple and powerful data access layer.
package com.enterprisemath.dao.big;
import com.enterprisemath.dao.filter.Criterium;
import com.enterprisemath.dao.filter.Filter;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.apache.ibatis.annotations.Param;
/**
* My batis mapper for big entity objects.
*
* @author radek.hecl
*
*/
public interface MyBatisBigEntityMapper {
/**
* Inserts big entity into table.
*
* @param table table
* @param fields field names
* @param values values
*/
public void insertBigEntity(@Param("table") String table, @Param("fields") List fields, @Param("values") List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy