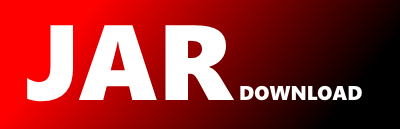
com.enterprisemath.dao.relational.Entity Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of em-dao Show documentation
Show all versions of em-dao Show documentation
Simple and powerful data access layer.
package com.enterprisemath.dao.relational;
import java.util.Collections;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import com.enterprisemath.utils.DomainUtils;
import com.enterprisemath.utils.ValidationUtils;
/**
* Entity object. This is a base object for everything.
* This is expected to be translated into more appropriate objects.
*
* @author radek.hecl
*
*/
public final class Entity {
/**
* Builder object.
*/
public static class Builder {
/**
* Code of the entity. This is unique identifier of each entity.
*/
private String code;
/**
* Name of the entity. This is user friendly name, not mandatory.
*/
private String name;
/**
* Created timestamp.
*/
private Date createdTimestamp;
/**
* Entity type.
*/
private String type;
/**
* Values from the joined tabled. Each key of the map has format table_name.column_name.
*/
private Map joins = new HashMap();
/**
* Code of the parent entity. It there is no parent entity, then this value is null.
*/
private String parentCode;
/**
* Sets code of the entity. This is unique identifier.
*
* @param code code of the entity
* @return this instance
*/
public Builder setCode(String code) {
this.code = code;
return this;
}
/**
* Sets name of the entity.
*
* @param name name of the entity
* @return this instance
*/
public Builder setName(String name) {
this.name = name;
return this;
}
/**
* Sets created timestamp. This is timestamp without time zone.
* It is up to the application to choose the appropriate time zone.
*
* @param createdTimestamp created timestamp
* @return this instance
*/
public Builder setCreatedTimestamp(Date createdTimestamp) {
this.createdTimestamp = DomainUtils.copyDate(createdTimestamp);
return this;
}
/**
* Sets type of the entity.
*
* @param type type of the entity
* @return this instance
*/
public Builder setType(String type) {
this.type = type;
return this;
}
/**
* Sets values from the join tables of the entity. Each key in the map has format table_name.column_name.
*
* @param joins values from join tables
* @return this instance
*/
public Builder setJoins(Map joins) {
this.joins = DomainUtils.softCopyMap(joins);
return this;
}
/**
* Adds join table value.
*
* @param key key of the property
* @param value value of the property
* @return this instance
*/
public Builder addJoin(String key, Object value) {
this.joins.put(key, value);
return this;
}
/**
* Sets code of the parent entity. If there is no parent, then this value is null.
*
* @param parentCode code of the parent entity
* @return this instance
*/
public Builder setParentCode(String parentCode) {
this.parentCode = parentCode;
return this;
}
/**
* Builds the result object.
*
* @return created object
*/
public Entity build() {
return new Entity(this);
}
}
/**
* Code of this entity. This is unique identifier of each entity.
*/
private String code;
/**
* Name of this entity. This is user friendly name, not mandatory.
*/
private String name;
/**
* Created timestamp.
*/
private Date createdTimestamp;
/**
* Entity type.
*/
private String type;
/**
* Values from the joined tabled. Each key of the map has format table_name.column_name.
*/
private Map joins;
/**
* Code of the parent entity. It there is no parent entity, then this value is null.
*/
private String parentCode;
/**
* Creates new instance.
*
* @param builder builder object
*/
public Entity(Builder builder) {
code = builder.code;
name = builder.name;
createdTimestamp = DomainUtils.copyDate(builder.createdTimestamp);
type = builder.type;
joins = Collections.unmodifiableMap(DomainUtils.softCopyMap(builder.joins));
parentCode = builder.parentCode;
guardInvariants();
}
/**
* Guards this object to be consistent. Throws exception if this is not the case.
*/
private void guardInvariants() {
ValidationUtils.guardNotEmpty(code, "code cannot be empty");
ValidationUtils.guardNotNull(name, "name cannot be null");
ValidationUtils.guardNotNull(createdTimestamp, "createdTimestamp cannot be null");
ValidationUtils.guardNotEmpty(type, "type cannot be empty");
ValidationUtils.guardNotEmptyStringInCollection(joins.keySet(), "joins cannot have empty key");
if (parentCode != null) {
ValidationUtils.guardNotEmpty(parentCode, "parentCode cannot be empty if defined");
}
}
/**
* Returns code of this entity. This is the unique identifier.
*
* @return code of this entity as a unique identifier
*/
public String getCode() {
return code;
}
/**
* Returns name of this entity.
*
* @return name of this entity
*/
public String getName() {
return name;
}
/**
* Returns created timestamp. This is timestamp without time zone.
* It is up to the application to choose the appropriate time zone.
*
* @return created timestamp
*/
public Date getCreatedTimestamp() {
return createdTimestamp;
}
/**
* Returns type of this entity.
*
* @return type of this entity
*/
public String getType() {
return type;
}
/**
* Returns joins of this entity. Each key of the result has format table_name.column_name.
*
* @return joins of this entity
*/
public Map getJoins() {
return joins;
}
/**
* Returns code of the parent entity. Returns null if there is no parent entity
*
* @return code of the parent entity or null
*/
public String getParentCode() {
return parentCode;
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
@Override
public boolean equals(Object obj) {
return EqualsBuilder.reflectionEquals(this, obj);
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy