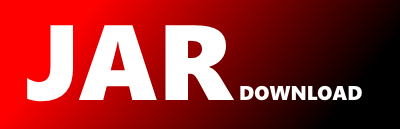
com.enterprisemath.dao.relational.MyBatisEntityMapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of em-dao Show documentation
Show all versions of em-dao Show documentation
Simple and powerful data access layer.
package com.enterprisemath.dao.relational;
import java.util.Date;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.apache.ibatis.annotations.Param;
import com.enterprisemath.dao.filter.Criterium;
import com.enterprisemath.dao.filter.Filter;
import java.util.Collection;
/**
* My batis mapper for entity objects.
* This is internal class and might be changed or removed at any time.
*
* @author radek.hecl
*
*/
public interface MyBatisEntityMapper {
/**
* Inserts the entity core.
*
* @param code code of the entity, this is the unique identifier
* @param name name of the entity
* @param createdTimestamp timestamp when entity was created
* @param parentCode code of the parent entity
* @param type type of the entity
*/
public void insertEntityCore(
@Param("code") String code,
@Param("name") String name,
@Param("createdTimestamp") Date createdTimestamp,
@Param("parent_code") String parentCode,
@Param("type") String type);
/**
* Insert joins into specified table for the specified entity.
*
* @param code code of the entity
* @param table table name for join
* @param join join values for the table
*/
public void insertEntityJoins(@Param("code") String code, @Param("table") String table, @Param("join") Map join);
/**
* Deletes joins from the specified entity and table.
*
* @param code identification code
* @param table join table
*/
public void deleteEntityJoins(@Param("code") String code, @Param("table") String table);
/**
* Selects entities core according the filter.
*
* @param joins joins which are selected together with entities
* @param filter filter to be used
* @return list with selected entities
*/
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy