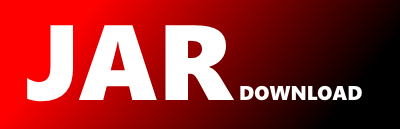
com.enterprisemath.dao.relational.Relation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of em-dao Show documentation
Show all versions of em-dao Show documentation
Simple and powerful data access layer.
package com.enterprisemath.dao.relational;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
import com.enterprisemath.utils.ValidationUtils;
/**
* Relation object. This defines relation ship between subject and object entities.
* It is up to the client to get meaning to the subject, object and
* whether this is one way or two ways relationship.
*
* @author radek.hecl
*
*/
public class Relation {
/**
* Builder object.
*
*/
public static class Builder {
/**
* Identification code.
*/
private String code;
/**
* Subject entity.
*/
private Entity subject;
/**
* Object entity.
*/
private Entity object;
/**
* Type of the relation.
*/
private String type;
/**
* Sets identification code.
*
* @param code identification code
* @return this instance
*/
public Builder setCode(String code) {
this.code = code;
return this;
}
/**
* Sets subject entity.
*
* @param subject subject entity
* @return this instance
*/
public Builder setSubject(Entity subject) {
this.subject = subject;
return this;
}
/**
* Sets object entity.
*
* @param object object entity
* @return this instance
*/
public Builder setObject(Entity object) {
this.object = object;
return this;
}
/**
* Sets relation type.
*
* @param type relation type
* @return this instance
*/
public Builder setType(String type) {
this.type = type;
return this;
}
/**
* Builds the result object.
*
* @return created object
*/
public Relation build() {
return new Relation(this);
}
}
/**
* Identification code.
*/
private String code;
/**
* Subject entity.
*/
private Entity subject;
/**
* Object entity.
*/
private Entity object;
/**
* Type of the relation.
*/
private String type;
/**
* Creates new instance.
*
* @param builder builder object
*/
public Relation(Builder builder) {
code = builder.code;
subject = builder.subject;
object = builder.object;
type = builder.type;
guardInvariants();
}
/**
* Guards this object to be consistent. Throws exception if this is not the case.
*/
private void guardInvariants() {
ValidationUtils.guardNotEmpty(code, "code cannot be empty");
ValidationUtils.guardNotNull(subject, "subject cannot be null");
ValidationUtils.guardNotNull(object, "object cannot be null");
ValidationUtils.guardNotEmpty(type, "type cannot be empty");
}
/**
* Returns identification code.
*
* @return identification code
*/
public String getCode() {
return code;
}
/**
* Returns subject entity.
*
* @return subject entity
*/
public Entity getSubject() {
return subject;
}
/**
* Returns object entity.
*
* @return object entity
*/
public Entity getObject() {
return object;
}
/**
* Returns the relation type.
*
* @return relation type
*/
public String getType() {
return type;
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
@Override
public boolean equals(Object obj) {
return EqualsBuilder.reflectionEquals(this, obj);
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this);
}
/**
* Shortcut to create new relation.
*
* @param code identification code
* @param subject subject
* @param object object
* @param type type
* @return created relation
*/
public static Relation create(String code, Entity subject, Entity object, String type) {
return new Relation.Builder().
setCode(code).
setSubject(subject).
setObject(object).
setType(type).
build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy