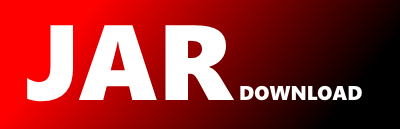
com.enterprisemath.eac.ServletRequestParameterModel Maven / Gradle / Ivy
package com.enterprisemath.eac;
import com.enterprisemath.utils.Dates;
import com.enterprisemath.utils.ValidationUtils;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import javax.servlet.ServletRequest;
import org.apache.commons.lang.StringUtils;
import org.apache.commons.lang3.builder.ToStringBuilder;
/**
* Implementation of request parameter model through servlet requests.
* Lists have notation of [propertyName[0], propertyName[1]...].
*
* @author radek.hecl
*/
public class ServletRequestParameterModel implements RequestParameterModel {
/**
* Source request object.
*/
private ServletRequest request;
/**
* Creates new instance.
*/
private ServletRequestParameterModel() {
}
/**
* Guards this object to be consistent. Throws exception if this is not the case.
*/
private void guardInvariants() {
ValidationUtils.guardNotNull(request, "request cannot be null");
}
@Override
public boolean isEmpty(String name) {
return StringUtils.isEmpty(request.getParameter(name));
}
@Override
public boolean isNotEmpty(String name) {
return StringUtils.isNotEmpty(request.getParameter(name));
}
@Override
public boolean getBoolean(String name) {
String res = request.getParameter(name);
if (res == null) {
throw new IndexOutOfBoundsException("parameter doesn't exists: " + name);
}
return Boolean.valueOf(res);
}
@Override
public Boolean getBooleanNonStrict(String name, Boolean def) {
String res = request.getParameter(name);
return res == null ? def : Boolean.valueOf(res);
}
@Override
public int getInt(String name) {
String res = request.getParameter(name);
if (res == null) {
throw new IndexOutOfBoundsException("parameter doesn't exists: " + name);
}
return Integer.valueOf(res);
}
@Override
public Integer getIntNonStrict(String name, Integer def) {
String res = request.getParameter(name);
return res == null ? def : Integer.valueOf(res);
}
@Override
public long getLong(String name) {
String res = request.getParameter(name);
if (res == null) {
throw new IndexOutOfBoundsException("parameter doesn't exists: " + name);
}
return Long.valueOf(res);
}
@Override
public Long getLongNonStrict(String name, Long def) {
String res = request.getParameter(name);
return res == null ? def : Long.valueOf(res);
}
@Override
public double getDouble(String name) {
String res = request.getParameter(name);
if (res == null) {
throw new IndexOutOfBoundsException("parameter doesn't exists: " + name);
}
return Double.valueOf(res);
}
@Override
public Double getDoubleNonStrict(String name, Double def) {
String res = request.getParameter(name);
return res == null ? def : Double.valueOf(res);
}
@Override
public String getString(String name) {
String res = request.getParameter(name);
if (res == null) {
throw new IndexOutOfBoundsException("parameter doesn't exists: " + name);
}
return res;
}
@Override
public String getStringNonStrict(String name, String def) {
String res = request.getParameter(name);
return res == null ? def : res;
}
@Override
public T getEnum(String name, Class clazz) {
String res = request.getParameter(name);
if (res == null) {
throw new IndexOutOfBoundsException("parameter doesn't exists: " + name);
}
return (T) createEnum(clazz, res);
}
@Override
public T getEnumNonStrict(String name, T def, Class clazz) {
String res = request.getParameter(name);
return res == null ? def : (T) createEnum(clazz, res);
}
@Override
public Date getDate(String name, String... patterns) {
String res = request.getParameter(name);
if (res == null) {
throw new IndexOutOfBoundsException("parameter doesn't exists: " + name);
}
return Dates.parse(res, patterns);
}
@Override
public Date getDateNonStrict(String name, Date def, String... patterns) {
String res = request.getParameter(name);
return res == null ? def : Dates.parse(res, patterns);
}
@Override
public List getStringList(String name) {
List res = new ArrayList<>();
for (int i = 0; i < Integer.MAX_VALUE; ++i) {
String p = getStringNonStrict(name + "[" + i + "]", null);
if (p == null) {
break;
}
res.add(p);
}
return res;
}
@Override
public List getEnumList(String name, Class clazz) {
List res = new ArrayList<>();
for (int i = 0; i < Integer.MAX_VALUE; ++i) {
String p = getStringNonStrict(name + "[" + i + "]", null);
if (p == null) {
break;
}
res.add((T) createEnum(clazz, p));
}
return res;
}
/**
* Creates enumeration.
*
* @param clazz enumeration class
* @param value string representation
* @return created enumeration
*/
private Object createEnum(Class> clazz, String value) {
try {
Method method = clazz.getMethod("valueOf", String.class);
Object res = method.invoke(null, value);
return res;
} catch (NoSuchMethodException e) {
throw new RuntimeException(e);
} catch (SecurityException e) {
throw new RuntimeException(e);
} catch (IllegalAccessException e) {
throw new RuntimeException(e);
} catch (IllegalArgumentException e) {
throw new RuntimeException(e);
} catch (InvocationTargetException e) {
throw new RuntimeException(e);
}
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this);
}
/**
* Creates new instance.
*
* @param request request
* @return created instance
*/
public static ServletRequestParameterModel create(ServletRequest request) {
ServletRequestParameterModel res = new ServletRequestParameterModel();
res.request = request;
res.guardInvariants();
return res;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy