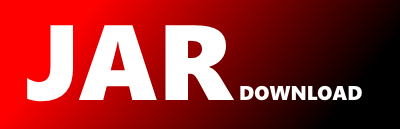
com.enterprisemath.math.nn.Synapse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of em-math Show documentation
Show all versions of em-math Show documentation
Advanced mathematical algorithms.
The newest version!
package com.enterprisemath.math.nn;
import com.enterprisemath.utils.ValidationUtils;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
/**
* Definition of synapse. This is connection between 2 neurons.
*
* @author radek.hecl
*/
public class Synapse {
/**
* Builder object.
*/
public static class Builder {
/**
* Id of neuron where synapse starts.
*/
private String startId;
/**
* Id of neuron where synapse ends.
*/
private String endId;
/**
* Weight.
*/
private double weight;
/**
* Sets id of the neuron where synapse starts.
*
* @param startId start neuron id
* @return this instance
*/
public Builder setStartId(String startId) {
this.startId = startId;
return this;
}
/**
* Sets id of the neuron where synapse end.
*
* @param endId end neuron id
* @return this instance
*/
public Builder setEndId(String endId) {
this.endId = endId;
return this;
}
/**
* Sets weight.
*
* @param weight weight
* @return this instance
*/
public Builder setWeight(double weight) {
this.weight = weight;
return this;
}
/**
* Builds the result object.
*
* @return created object
*/
public Synapse build() {
return new Synapse(this);
}
}
/**
* Id of neuron where synapse starts.
*/
private String startId;
/**
* Id of neuron where synapse ends.
*/
private String endId;
/**
* Weight.
*/
private double weight;
/**
* Creates new instance.
*
* @param builder builder object
*/
public Synapse(Builder builder) {
this.startId = builder.startId;
this.endId = builder.endId;
this.weight = builder.weight;
guardInvariants();
}
/**
* Guards this object to be consistent. Throws exception if this is not the case.
*/
private void guardInvariants() {
ValidationUtils.guardNotEmpty(startId, "startId cannot be empty");
ValidationUtils.guardNotEmpty(endId, "endId cannot be empty");
}
/**
* Returns neuron id where synapse starts.
*
* @return neuron id where synapse starts
*/
public String getStartId() {
return startId;
}
/**
* Returns neuron id where synapse ends.
*
* @return neuron id where synapse ends
*/
public String getEndId() {
return endId;
}
/**
* Returns wheight.
*
* @return weight
*/
public double getWeight() {
return weight;
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
@Override
public boolean equals(Object obj) {
return EqualsBuilder.reflectionEquals(this, obj);
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this);
}
/**
* Creates new instance.
*
* @param startId identification of start neuron
* @param endId identification of end neuron
* @param weight weight
* @return created instance
*/
public static Synapse create(String startId, String endId, double weight) {
return new Synapse.Builder().
setStartId(startId).
setEndId(endId).
setWeight(weight).
build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy