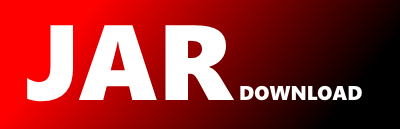
com.enterprisemath.utils.image.ConstantFileImageAnimation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of em-utils Show documentation
Show all versions of em-utils Show documentation
Collection of utility classes for large scale projects focusing on robust and testable code.
package com.enterprisemath.utils.image;
import java.awt.image.BufferedImage;
import java.awt.image.RenderedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
import org.apache.commons.lang3.builder.ToStringBuilder;
import com.enterprisemath.utils.ValidationUtils;
/**
* Implementation of image animation which takes frames from one constant file image.
* One image is used for all frames.
*
* @author radek.hecl
*
*/
public class ConstantFileImageAnimation implements ImageAnimation {
/**
* Builder object.
*/
public static class Builder {
/**
* Frame width.
*/
private Integer frameWidth;
/**
* Frame height.
*/
private Integer frameHeight;
/**
* Frame duration in milliseconds.
*/
private Integer frameDuration;
/**
* Number of frames.
*/
private Integer numFrames;
/**
* Path to the frame file.
*/
private String framePath;
/**
* Sets frame width.
*
* @param frameWidth frame width
* @return this instance
*/
public Builder setFrameWidth(Integer frameWidth) {
this.frameWidth = frameWidth;
return this;
}
/**
* Sets frame height.
*
* @param frameHeight frame height
* @return this instance
*/
public Builder setFrameHeight(Integer frameHeight) {
this.frameHeight = frameHeight;
return this;
}
/**
* Sets frame duration in milliseconds.
*
* @param frameDuration frame duration in milliseconds
* @return this instance
*/
public Builder setFrameDuration(Integer frameDuration) {
this.frameDuration = frameDuration;
return this;
}
/**
* Sets number of frames.
*
* @param numFrames number of frames
* @return this instance
*/
public Builder setNumFrames(Integer numFrames) {
this.numFrames = numFrames;
return this;
}
/**
* Sets path to the frame.
*
* @param framePath path to the frame
* @return this instance
*/
public Builder setFramePath(String framePath) {
this.framePath = framePath;
return this;
}
/**
* Builds the result object.
*
* @return created object
*/
public ConstantFileImageAnimation build() {
return new ConstantFileImageAnimation(this);
}
}
/**
* Frame width.
*/
private Integer frameWidth;
/**
* Frame height.
*/
private Integer frameHeight;
/**
* Frame duration in milliseconds.
*/
private Integer frameDuration;
/**
* Number of frames.
*/
private Integer numFrames;
/**
* Path to the frame file.
*/
private String framePath;
/**
* Creates new instance.
*
* @param builder builder object
*/
public ConstantFileImageAnimation(Builder builder) {
frameWidth = builder.frameWidth;
frameHeight = builder.frameHeight;
frameDuration = builder.frameDuration;
numFrames = builder.numFrames;
framePath = builder.framePath;
guardInvariants();
}
/**
* Guards this object to be consistent. Throws exception if this is not the case.
*/
private void guardInvariants() {
ValidationUtils.guardPositiveInt(frameWidth, "frameWidth must be positive");
ValidationUtils.guardPositiveInt(frameHeight, "frameHeight must be positive");
ValidationUtils.guardPositiveInt(frameDuration, "frameDuration must be positive");
ValidationUtils.guardNotNegativeInt(numFrames, "numFrames cannot be negative");
ValidationUtils.guardNotEmpty(framePath, "framePath cannot be empty");
}
@Override
public int getFrameWidth() {
return frameWidth;
}
@Override
public int getFrameHeight() {
return frameHeight;
}
@Override
public int getNumFrames() {
return numFrames;
}
@Override
public int getFrameDuration() {
return frameDuration;
}
@Override
public RenderedImage getFrame(int index) {
try {
BufferedImage img = ImageIO.read(new File(framePath));
ValidationUtils.guardEquals(frameWidth, img.getWidth(), "image width does not match the frameWidth: " +
"frameWidth = " + frameWidth + "; " +
"imageWidth = " + img.getWidth() + "; " +
"path = " + framePath);
ValidationUtils.guardEquals(frameHeight, img.getHeight(), "image height does not match the frameHeight: " +
"frameHeight = " + frameHeight + "; " +
"imageHeight = " + img.getHeight() + "; " +
"path = " + framePath);
return img;
} catch (IOException e) {
throw new RuntimeException(e);
}
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this);
}
/**
* Creates new instance. frame size is calculated from the image.
*
* @param framePath path to the frame image
* @param frameDuration frame duration
* @param numFrames number of frames
* @return created object
*/
public static ConstantFileImageAnimation create(String framePath, int frameDuration, int numFrames) {
try {
BufferedImage img = ImageIO.read(new File(framePath));
return new ConstantFileImageAnimation.Builder().
setFrameWidth(img.getWidth()).
setFrameHeight(img.getHeight()).
setFrameDuration(frameDuration).
setNumFrames(numFrames).
setFramePath(framePath).
build();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy