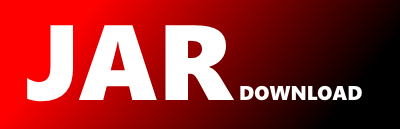
com.enterprisemath.utils.CsvUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of em-utils Show documentation
Show all versions of em-utils Show documentation
Collection of utility classes for large scale projects focusing on robust and testable code.
package com.enterprisemath.utils;
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.nio.charset.Charset;
import java.util.ArrayList;
import java.util.List;
import org.apache.commons.io.IOUtils;
import org.apache.commons.lang3.StringUtils;
/**
* Class which contains utility methods to work with CSV files.
*
* @author radek.hecl
*/
public final class CsvUtils {
/**
* Prevent construction.
*/
private CsvUtils() {
}
/**
* Parses data. Encoding is UFT-8.
*
* @param data data
* @return csv records
*/
public static List> parse(byte[] data) {
ByteArrayInputStream bis = null;
List lines = null;
try {
bis = new ByteArrayInputStream(data);
lines = IOUtils.readLines(bis, "utf-8");
bis.close();
} catch (IOException e) {
throw new RuntimeException(e);
} finally {
IOUtils.closeQuietly(bis);
}
List> res = new ArrayList>(lines.size() - 1);
List csvLine = new ArrayList();
boolean escapedCell = false;
StringBuilder cellBuilder = null;
for (int l = 0; l < lines.size(); ++l) {
StringParser parser = new StringParser(lines.get(l));
while (parser.hasNext()) {
if (cellBuilder == null) {
String chr = parser.readCharacter();
if (chr.equals(",")) {
csvLine.add("");
}
else if (chr.equals("\"")) {
escapedCell = true;
cellBuilder = new StringBuilder();
}
else {
escapedCell = false;
cellBuilder = new StringBuilder();
cellBuilder.append(chr);
}
}
else {
if (escapedCell) {
String str = parser.readTillStop("\"");
cellBuilder.append(str);
if (parser.hasNext()) {
parser.readCharacter();
if (parser.hasNext()) {
String next = parser.readCharacter();
if (next.equals("\"")) {
cellBuilder.append("\"");
}
else if (next.equals(",")) {
csvLine.add(cellBuilder.toString());
cellBuilder = null;
}
}
else {
csvLine.add(cellBuilder.toString());
cellBuilder = null;
}
}
}
else {
String str = parser.readTillStop(",");
cellBuilder.append(str);
csvLine.add(cellBuilder.toString());
cellBuilder = null;
if (parser.hasNext()) {
parser.readCharacter();
}
}
}
}
if (cellBuilder == null) {
res.add(csvLine);
csvLine = new ArrayList();
}
else {
cellBuilder.append("\n");
}
}
if (cellBuilder != null) {
throw new IllegalArgumentException("last cell in file is not closed: line = " + lines.size());
}
return res;
}
/**
* Formats data. Encoding is UFT-8.
*
* @param data data
* @return csv file
*/
public static byte[] format(List> data) {
StringBuilder res = new StringBuilder();
for (List line : data) {
for (int i = 0; i < line.size(); ++i) {
String cell = StringUtils.defaultString(line.get(i));
cell = cell.replaceAll("\"", "\"\"");
res.append("\"").append(cell).append("\"");
if (i == line.size() - 1) {
res.append("\n");
}
else {
res.append(",");
}
}
}
return res.toString().getBytes(Charset.forName("utf-8"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy