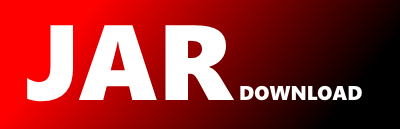
com.enterprisemath.utils.Dates Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of em-utils Show documentation
Show all versions of em-utils Show documentation
Collection of utility classes for large scale projects focusing on robust and testable code.
package com.enterprisemath.utils;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Arrays;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.TimeZone;
import org.apache.commons.lang3.time.DateUtils;
/**
* Class with utility methods for work with dates and times.
*
* @author radek.hecl
*
*/
public class Dates {
/**
* Prevents construction from outside.
*/
private Dates() {
}
/**
* Returns the current timestamp.
*
* @return current timestamp
*/
public static Date now() {
return new Date();
}
/**
* Returns todays date. This is current timestamp which is truncated to days.
*
* @return todays date
*/
public static Date today() {
return DateUtils.truncate(new Date(), Calendar.DATE);
}
/**
* Returns the today's date in the specified time zone.
* Example: JVM local time zone is JST (Japan Standard Time) and provided time zone is PST (Pacific Standard Time).
* Result will be date truncated to the day in JST (as all java.util.Date are in local time zone).
* But as PST is 17 hours behind the JST, the returned date can be today or yesterday.
*
* @param timezone time zone to use
* @return created date
*/
@Deprecated
public static Date todayWithSchiftedTimeZone(TimeZone timezone) {
Calendar calendar = Calendar.getInstance(timezone);
Month month = null;
switch (calendar.get(Calendar.MONTH)) {
case 0:
month = Month.JANUARY;
break;
case 1:
month = Month.FEBRUARY;
break;
case 2:
month = Month.MARCH;
break;
case 3:
month = Month.APRIL;
break;
case 4:
month = Month.MAY;
break;
case 5:
month = Month.JUNE;
break;
case 6:
month = Month.JULY;
break;
case 7:
month = Month.AUGUST;
break;
case 8:
month = Month.SEPTEMBER;
break;
case 9:
month = Month.OCTOBER;
break;
case 10:
month = Month.NOVEMBER;
break;
case 11:
month = Month.DECEMBER;
break;
default:
throw new IllegalArgumentException("unsupported month: calendar = " + calendar);
}
return Dates.createDate(calendar.get(Calendar.YEAR), month, calendar.get(Calendar.DAY_OF_MONTH));
}
/**
* Returns the yesterdays date. This is truncated to date.
*
* @return yesterdays date
*/
public static Date yesterday() {
return DateUtils.addDays(today(), -1);
}
/**
* Returns the tomorrows date. This is truncated to date.
*
* @return tomorrows date
*/
public static Date tomorrow() {
return DateUtils.addDays(today(), 1);
}
/**
* Returns the date which is shifted for a particular number of days from
* today. This is truncated to date.
*
* @param numDays the number of days to shift
* @return date shifted from today
*/
public static Date fromToday(int numDays) {
return DateUtils.addDays(today(), numDays);
}
/**
* Creates date according the Gregorian calendar input.
*
* @param year year
* @param month month
* @param day day of the month (1-31, range depends on the month and year)
* @return created date
*/
public static Date createDate(int year, Month month, int day) {
return createTime(year, month, day, 0, 0, 0, 0);
}
/**
* Creates time according the Gregorian calendar input.
*
* @param year year
* @param month month
* @param day day of the month (1-31, range depends on the month and year)
* @param hour hour (0 - 23)
* @param minute minute (0 - 59)
* @param second second (0 - 59)
* @return created time
*/
public static Date createTime(int year, Month month, int day, int hour, int minute, int second) {
return createTime(year, month, day, hour, minute, second, 0);
}
/**
* Creates time according the Gregorian calendar input.
*
* @param year year
* @param month month
* @param day day of the month (1-31, range depends on the month and year)
* @param hour hour (0 - 23)
* @param minute minute (0 - 59)
* @param second second (0 - 59)
* @param millisecond millisecond (0-999)
* @return created time
*/
public static Date createTime(int year, Month month, int day, int hour, int minute, int second, int millisecond) {
Calendar calendar = new GregorianCalendar();
calendar.clear();
calendar.set(Calendar.YEAR, year);
switch (month) {
case JANUARY:
calendar.set(Calendar.MONTH, 0);
break;
case FEBRUARY:
calendar.set(Calendar.MONTH, 1);
break;
case MARCH:
calendar.set(Calendar.MONTH, 2);
break;
case APRIL:
calendar.set(Calendar.MONTH, 3);
break;
case MAY:
calendar.set(Calendar.MONTH, 4);
break;
case JUNE:
calendar.set(Calendar.MONTH, 5);
break;
case JULY:
calendar.set(Calendar.MONTH, 6);
break;
case AUGUST:
calendar.set(Calendar.MONTH, 7);
break;
case SEPTEMBER:
calendar.set(Calendar.MONTH, 8);
break;
case OCTOBER:
calendar.set(Calendar.MONTH, 9);
break;
case NOVEMBER:
calendar.set(Calendar.MONTH, 10);
break;
case DECEMBER:
calendar.set(Calendar.MONTH, 11);
break;
default:
throw new IllegalArgumentException("unknown month: month = " + month);
}
calendar.set(Calendar.DATE, day);
calendar.set(Calendar.HOUR_OF_DAY, hour);
calendar.set(Calendar.MINUTE, minute);
calendar.set(Calendar.SECOND, second);
calendar.set(Calendar.MILLISECOND, millisecond);
return calendar.getTime();
}
/**
* Takes the time in a given date and returns how many minutes past from the
* specified day from 0 : 0 : 0.
* Examples:
*
* - 1st January 2012 00 : 00 : 00 => 0
* - 1st January 2012 00 : 00 : 14 => 0
* - 1st January 2012 00 : 59 : 14 => 59
* - 1st January 2012 01 : 00 : 35 => 60
* - 1st January 2012 01 : 12 : 35 => 72
* - 1st January 2012 23 : 59 : 59 => 1439 (23 * 60 + 59)
*
*
* @param date input date
* @return how many minutes past from the noon of the day in the specified timestamp
*/
public static int getMinutesInDay(Date date) {
Calendar calendar = new GregorianCalendar();
calendar.setTime(date);
return calendar.get(Calendar.HOUR_OF_DAY) * 60 + calendar.get(Calendar.MINUTE);
}
/**
* Takes the time in a given date and returns how many hours past from the
* specified day from 0 : 0 : 0.
* Examples:
*
* - 1st January 2012 00 : 00 : 00 => 0
* - 1st January 2012 00 : 00 : 14 => 0
* - 1st January 2012 00 : 59 : 14 => 0
* - 1st January 2012 01 : 00 : 35 => 1
* - 1st January 2012 01 : 12 : 35 => 1
* - 1st January 2012 23 : 59 : 59 => 23
*
*
* @param date input date
* @return how many hours past from the noon of the day in the specified timestamp
*/
public static int getHoursInDay(Date date) {
Calendar calendar = new GregorianCalendar();
calendar.setTime(date);
return calendar.get(Calendar.HOUR_OF_DAY);
}
/**
* Takes the time in a given date and returns how many days past from the beginning of the year.
* This is value in range <0, 364> for the year which does not have 29 February and value
* in rage <0, 365> for the year which has 29 February.
* Examples:
*
* - 1st January 2011 => 0
* - 31th January 2011 => 30
* - 1st August 2011 => 212
* - 31st December 2011 => 364
* - 1st January 2012 => 0
* - 31th January 2012 => 30
* - 1st August 2012 => 213
* - 31st December 2012 => 365
*
*
* @param date input date
* @return how many days past from the beginning of the year
*/
public static int getDaysInYear(Date date) {
Calendar calendar = new GregorianCalendar();
calendar.setTime(date);
return calendar.get(Calendar.DAY_OF_YEAR) - 1;
}
/**
* Returns the difference in days between two dates. Only the date and more
* significant fields are included in the calculation. The formula is:
* numberOfDays(truncToDays(second) - truncToDays(first)).
* Examples:
*
* - (1st January 2012 00 : 00 : 00, 1st January 2012 00 : 00 : 00) => 0
* - (1st January 2012 00 : 00 : 00, 1st January 2012 23 : 59 : 59) => 0
* - (1st January 2012 23 : 59 : 59, 1st January 2012 00 : 00 : 00) => 0
* - (1st January 2012 00 : 00 : 00, 2nd January 2012 00 : 00 : 00) => 1
* - (1st January 2012 23 : 59 : 59, 2nd January 2012 00 : 00 : 00) => 1
* - (1st January 2012 23 : 59 : 59, 2nd January 2012 23 : 59 : 59) => 1
* - (2nd January 2012 00 : 00 : 00, 1st January 2012 23 : 59 : 59) => -1
* - (1st January 2012 00 : 00 : 00, 1st February 2012 00 : 00 : 00) => 31
*
*
* @param first first date
* @param second second date
* @return the days difference
*/
public static int getDifferenceInDays(Date first, Date second) {
Date firstDay = DateUtils.truncate(first, Calendar.DATE);
Date secondDay = DateUtils.truncate(second, Calendar.DATE);
return Long.valueOf((secondDay.getTime() - firstDay.getTime()) / 1000 / 60 / 60 / 24).intValue();
}
/**
* Returns the string with the formatted date with the specified pattern.
* This method is deprecated and will be removed. Use format instead.
*
* @param date date which will be formatted
* @param pattern pattern which will be used
* @return string with formatted date
*/
@Deprecated
public static String getFormattedDate(Date date, String pattern) {
return format(date, pattern);
}
/**
* Formats date into string.
*
* @param date date which will be formatted
* @param pattern pattern which will be used
* @return string with formatted date
*/
public static String format(Date date, String pattern) {
SimpleDateFormat sdf = new SimpleDateFormat(pattern);
return sdf.format(date);
}
/**
* Returns the date which is created from the sting and specified format.
* This method is deprecated and will be removed. Use parse instead.
*
* @param date date string which will be parsed
* @param pattern pattern which will be used
* @return created date
*/
@Deprecated
public static Date getDateFromString(String date, String pattern) {
return parse(date, pattern);
}
/**
* Parses date.
*
* @param date date string which will be parsed
* @param patterns patterns which will be used, result is returned from the first pattern which can parse the string
* @return created date
*/
public static Date parse(String date, String... patterns) {
for (String pattern : patterns) {
SimpleDateFormat sdf = new SimpleDateFormat(pattern);
try {
return sdf.parse(date);
} catch (ParseException e) {
// ignore this as another pattern is in use
// if no pattern matches, then exception is thrown t the end of the loop
}
}
throw new IllegalArgumentException("no pattern matches the date format: " +
"date = " + date + ", patterns = " + Arrays.asList(patterns));
}
/**
* Null safe equals method which ignores anything less than seconds.
* Returns true if both objects are null or represents the same date up to the seconds.
* Ignores anything less than second.
* Examples:
*
* - (null, null) => true
* - (2012/01/02 00:00:01.000, null) => false
* - (null, 2012/01/02 00:00:01.999) => false
* - (2012/01/02 00:00:01.000, 2012/01/02 00:00:01.999) => true
* - (2012/01/02 00:00:01.000, 2012/01/02 00:00:00.999) => false
* - (2012/01/02 00:00:01.000, 2012/01/02 00:00:02.000) => false
*
*
* @param date1 first date
* @param date2 second date
* @return true if both dates are null or if they equals up to second, false otherwise
*/
public static boolean safeEqualsToSeconds(Date date1, Date date2) {
if (date1 == null && date2 == null) {
return true;
}
if (date1 == null || date2 == null) {
return false;
}
return DateUtils.truncatedEquals(date1, date2, Calendar.SECOND);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy