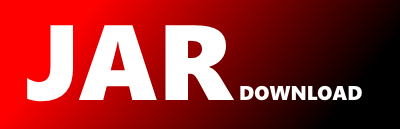
com.enterprisemath.utils.image.PauseFrameImageAnimation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of em-utils Show documentation
Show all versions of em-utils Show documentation
Collection of utility classes for large scale projects focusing on robust and testable code.
package com.enterprisemath.utils.image;
import java.awt.image.RenderedImage;
import org.apache.commons.lang3.builder.ToStringBuilder;
import com.enterprisemath.utils.ValidationUtils;
/**
* Implementation of image animation which takes frame from another animation
* and pauses it for a specified number of frames.
*
* @author radek.hecl
*
*/
public class PauseFrameImageAnimation implements ImageAnimation {
/**
* Builder object.
*/
public static class Builder {
/**
* Source animation.
*/
private ImageAnimation source;
/**
* Index of frame which is used from source animation.
*/
private Integer frameIndex;
/**
* Number of frames. This means how many times the frame is used.
*/
private Integer numFrames;
/**
* Sets source animation.
*
* @param source source animation
* @return this instance
*/
public Builder setSource(ImageAnimation source) {
this.source = source;
return this;
}
/**
* Sets index of frame which is used from the source animation.
*
* @param frameIndex index of frame which is used from the source animation
* @return this instance
*/
public Builder setFrameIndex(Integer frameIndex) {
this.frameIndex = frameIndex;
return this;
}
/**
* Sets number of frames. This means how many times the frame is reused.
*
* @param numFrames number of frames
* @return this instance
*/
public Builder setNumFrames(Integer numFrames) {
this.numFrames = numFrames;
return this;
}
/**
* Builds the result object.
*
* @return created object
*/
public PauseFrameImageAnimation build() {
return new PauseFrameImageAnimation(this);
}
}
/**
* Source animation.
*/
private ImageAnimation source;
/**
* Index of frame which is used from source animation.
*/
private Integer frameIndex;
/**
* Number of frames. This means how many times the frame is used.
*/
private Integer numFrames;
/**
* Creates new instance.
*
* @param builder builder object
*/
public PauseFrameImageAnimation(Builder builder) {
source = builder.source;
frameIndex = builder.frameIndex;
numFrames = builder.numFrames;
guardInvariants();
}
/**
* Guards this object to be consistent. Throws exception if this is not the case.
*/
private void guardInvariants() {
ValidationUtils.guardNotNull(source, "source cannot be null");
ValidationUtils.guardNotNull(frameIndex, "frameIndex cannot be null");
ValidationUtils.guardNotNegativeInt(numFrames, "numFrames cannot be negative");
}
@Override
public int getFrameWidth() {
return source.getFrameWidth();
}
@Override
public int getFrameHeight() {
return source.getFrameHeight();
}
@Override
public int getNumFrames() {
return numFrames;
}
@Override
public int getFrameDuration() {
return source.getFrameDuration();
}
@Override
public RenderedImage getFrame(int index) {
return source.getFrame(frameIndex);
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this);
}
/**
* Creates new instance.
*
* @param source source animation
* @param frameIndex index of frame to be used
* @param numFrames number of frames
* @return created object
*/
public static PauseFrameImageAnimation create(ImageAnimation source, int frameIndex, int numFrames) {
return new PauseFrameImageAnimation.Builder().
setSource(source).
setFrameIndex(frameIndex).
setNumFrames(numFrames).
build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy