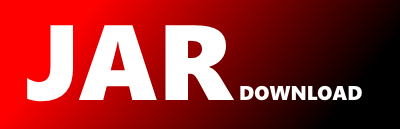
com.enterprisemath.utils.image.SequenceImageAnimation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of em-utils Show documentation
Show all versions of em-utils Show documentation
Collection of utility classes for large scale projects focusing on robust and testable code.
package com.enterprisemath.utils.image;
import java.awt.image.RenderedImage;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import org.apache.commons.lang3.builder.ToStringBuilder;
import com.enterprisemath.utils.DomainUtils;
import com.enterprisemath.utils.ValidationUtils;
/**
* Implementation of image animation which creates sequence from the several animations.
* Precondition is that all animations have same frame size and frame duration.
*
* @author radek.hecl
*
*/
public class SequenceImageAnimation implements ImageAnimation {
/**
* Builder object.
*/
public static class Builder {
/**
* Animations in the sequence.
*/
private List animations = new ArrayList();
/**
* Sets animations in the sequence.
*
* @param animations animations
* @return this instance
*/
public Builder setAnimations(List animations) {
this.animations = DomainUtils.softCopyList(animations);
return this;
}
/**
* Adds animation to the sequence.
*
* @param animation animation
* @return this instance
*/
public Builder addAnimation(ImageAnimation animation) {
animations.add(animation);
return this;
}
/**
* Builds the result object.
*
* @return created object
*/
public SequenceImageAnimation build() {
return new SequenceImageAnimation(this);
}
}
/**
* Animations in the sequence.
*/
private List animations;
/**
* Creates new instance.
*
* @param builder builder object
*/
public SequenceImageAnimation(Builder builder) {
animations = Collections.unmodifiableList(DomainUtils.softCopyList(builder.animations));
guardInvariants();
}
/**
* Guards this object to be consistent. Throws exception if this is not the case.
*/
private void guardInvariants() {
ValidationUtils.guardNotNullCollection(animations, "animations cannot have null elemen");
ValidationUtils.guardPositiveInt(animations.size(), "at least one animation must be defined");
int w = animations.get(0).getFrameWidth();
int h = animations.get(0).getFrameHeight();
int d = animations.get(0).getFrameDuration();
for (ImageAnimation a : animations) {
ValidationUtils.guardEquals(w, a.getFrameWidth(), "frameWidth must be same for all animations in sequence");
ValidationUtils.guardEquals(h, a.getFrameHeight(), "frameHeight must be same for all animations in sequence");
ValidationUtils.guardEquals(d, a.getFrameDuration(), "frameDuration must be same for all animations in sequence");
}
}
@Override
public int getFrameWidth() {
return animations.get(0).getFrameWidth();
}
@Override
public int getFrameHeight() {
return animations.get(0).getFrameHeight();
}
@Override
public int getNumFrames() {
int res = 0;
for (ImageAnimation a : animations) {
res = res + a.getNumFrames();
}
return res;
}
@Override
public int getFrameDuration() {
return animations.get(0).getFrameDuration();
}
@Override
public RenderedImage getFrame(int index) {
for (ImageAnimation a : animations) {
if (index < a.getNumFrames()) {
return a.getFrame(index);
}
index = index - a.getNumFrames();
}
throw new IndexOutOfBoundsException("frame index is out of bound: index = " + index);
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy