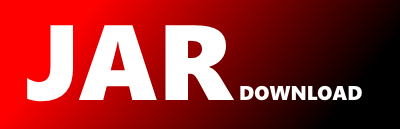
com.enterprisemath.utils.JSONUtils Maven / Gradle / Ivy
package com.enterprisemath.utils;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.SortedMap;
import java.util.TreeMap;
import org.json.JSONArray;
import org.json.JSONObject;
/**
* Utilities to work with JSON.
*
* @author radek.hecl
*/
public class JSONUtils {
/**
* Prevents construction.
*/
private JSONUtils() {
}
/**
* Encodes string map as a JSON object
*
* @param data data
* @return encoded string
*/
public static String encodeStringMap(Map data) {
SortedMap sorted = new TreeMap(PropertyStringComparator.create());
sorted.putAll(data);
List items = new ArrayList();
for (String key : sorted.keySet()) {
JSONObject item = new JSONObject();
item.put("key", key);
item.put("value", sorted.get(key));
items.add(item);
}
JSONArray array = new JSONArray(items);
return array.toString();
}
/**
* Decodes string map from the JSON object.
*
* @param data data
* @return decoded map
*/
public static Map decodeStringMap(String data) {
Map res = new HashMap();
JSONArray array = new JSONArray(data);
for (int i = 0; i < array.length(); ++i) {
JSONObject item = (JSONObject) array.get(i);
String key = item.getString("key");
String value = item.getString("value");
res.put(key, value);
}
return res;
}
/**
* Encodes string list as a JSON object
*
* @param data data
* @return encoded string
*/
public static String encodeStringList(List data) {
JSONArray res = new JSONArray();
for (String elm : data) {
res.put(elm);
}
return res.toString();
}
/**
* Decodes string list from the JSON object.
*
* @param data data
* @return decoded map
*/
public static List decodeStringList(String data) {
List res = new ArrayList();
JSONArray array = new JSONArray(data);
for (int i = 0; i < array.length(); ++i) {
String item = (String) array.get(i);
res.add(item);
}
return res;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy