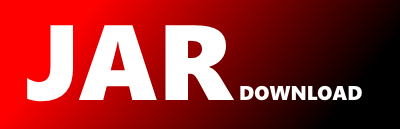
com.enterprisemath.utils.cache.ExpiringInMemoryObjectCache Maven / Gradle / Ivy
package com.enterprisemath.utils.cache;
import com.enterprisemath.utils.DomainUtils;
import com.enterprisemath.utils.ValidationUtils;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.NoSuchElementException;
import java.util.Set;
import org.apache.commons.lang3.builder.ToStringBuilder;
/**
* Implementation of object cache which stores everything in memory and objects expires after
* defined period of time.
*
* @author radek.hecl
*/
public class ExpiringInMemoryObjectCache implements ObjectCache {
/**
* Builder object.
*/
public static class Builder {
/**
* Timeout for object expiration. In milliseconds.
*/
private int expireTimeout = 0;
/**
* Whether timeout should be renewed when calling get method.
*/
private Boolean renewOnGet = null;
/**
* Sets timeout for object expiration.
*
* @param expireTimeout timeout for object expiration, in milliseconds
* @return this instance
*/
public Builder setExpireTimeout(int expireTimeout) {
this.expireTimeout = expireTimeout;
return this;
}
/**
* Sets whether timeout should be renewed when calling get method.
*
* @param renewOnGet whether timeout should be renewed when calling get method
* @return this instance
*/
public Builder setRenewOnGet(Boolean renewOnGet) {
this.renewOnGet = renewOnGet;
return this;
}
/**
* Builds the result object.
*
* @return created object
*/
public ExpiringInMemoryObjectCache build() {
return new ExpiringInMemoryObjectCache(this);
}
}
/**
* Timeout for object expiration. In milliseconds.
*/
private int expireTimeout;
/**
* Whether timeout should be renewed when calling get method.
*/
private Boolean renewOnGet;
/**
* Buffer map.
*/
private Map> buffer;
/**
* Object for locking.
*/
private final Object lock = new Object();
/**
* Creates new instance.
*
* @param builder builder object
*/
public ExpiringInMemoryObjectCache(Builder builder) {
expireTimeout = builder.expireTimeout;
renewOnGet = builder.renewOnGet;
guardInvariants();
buffer = new HashMap>();
}
/**
* Guards this object to be consistent. Throws exception if this is not the case.
*/
private void guardInvariants() {
ValidationUtils.guardPositiveInt(expireTimeout, "expireTimeout must be positive");
ValidationUtils.guardNotNull(renewOnGet, "renewOnGet cannot be null");
}
@Override
public void put(String key, Object object) {
synchronized (lock) {
expireOld();
Long et = System.currentTimeMillis() + expireTimeout;
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy