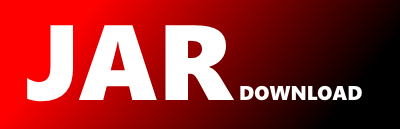
com.enterprisemath.utils.engine.TaskRunSuccessReport Maven / Gradle / Ivy
package com.enterprisemath.utils.engine;
import com.enterprisemath.utils.DomainUtils;
import com.enterprisemath.utils.ValidationUtils;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
/**
* Defines report from the successful task.
*
* @author radek.hecl
*/
public class TaskRunSuccessReport {
/**
* Builder object.
*/
public static class Builder {
/**
* Task identification code.
*/
private String code;
/**
* Task class.
*/
private String taskClass;
/**
* Parameters.
*/
private Map parameters = new HashMap();
/**
* Result.
*/
private Map result = new HashMap();
/**
* Start timestamp.
*/
private Date startTimestamp;
/**
* End timestamp.
*/
private Date endTimestamp;
/**
* Sets identification code.
*
* @param code identification code
* @return this instance
*/
public Builder setCode(String code) {
this.code = code;
return this;
}
/**
* Sets task class.
*
* @param taskClass task class
* @return this instance
*/
public Builder setTaskClass(String taskClass) {
this.taskClass = taskClass;
return this;
}
/**
* Sets parameters.
*
* @param parameters parameters
* @return this instance
*/
public Builder setParameters(Map parameters) {
this.parameters = DomainUtils.softCopyMap(parameters);
return this;
}
/**
* Sets result.
*
* @param result result
* @return this instance
*/
public Builder setResult(Map result) {
this.result = DomainUtils.softCopyMap(result);
return this;
}
/**
* Sets start timestamp.
*
* @param startTimestamp start timestamp
* @return this instance
*/
public Builder setStartTimestamp(Date startTimestamp) {
this.startTimestamp = DomainUtils.copyDate(startTimestamp);
return this;
}
/**
* Sets end timestamp.
*
* @param endTimestamp end timestamp
* @return this instance
*/
public Builder setEndTimestamp(Date endTimestamp) {
this.endTimestamp = DomainUtils.copyDate(endTimestamp);
return this;
}
/**
* Builds the result object.
*
* @return created object
*/
public TaskRunSuccessReport build() {
return new TaskRunSuccessReport(this);
}
}
/**
* Task identification code.
*/
private String code;
/**
* Task class.
*/
private String taskClass;
/**
* Parameters.
*/
private Map parameters;
/**
* Result.
*/
private Map result;
/**
* Start timestamp.
*/
private Date startTimestamp;
/**
* End timestamp.
*/
private Date endTimestamp;
/**
* Creates new instance.
*
* @param builder builder object
*/
public TaskRunSuccessReport(Builder builder) {
code = builder.code;
taskClass = builder.taskClass;
parameters = DomainUtils.softCopyUnmodifiableMap(builder.parameters);
result = DomainUtils.softCopyUnmodifiableMap(builder.result);
startTimestamp = DomainUtils.copyDate(builder.startTimestamp);
endTimestamp = DomainUtils.copyDate(builder.endTimestamp);
guardInvariants();
}
/**
* Guards this object to be consistent. Throws exception if this is not the case.
*/
private void guardInvariants() {
ValidationUtils.guardNotEmpty(code, "code cannot be empty");
ValidationUtils.guardNotEmpty(taskClass, "taskClass cannot be empty");
ValidationUtils.guardNotEmptyNullMap(parameters, "parameters cannot have empty key or null value");
ValidationUtils.guardNotEmptyNullMap(result, "result cannot have empty key or null value");
ValidationUtils.guardNotNull(startTimestamp, "startTimestamp cannot be null");
ValidationUtils.guardNotNull(endTimestamp, "endTimestamp cannot be null");
}
/**
* Returns identification code.
*
* @return identification code
*/
public String getCode() {
return code;
}
/**
* Returns task class.
*
* @return task class
*/
public String getTaskClass() {
return taskClass;
}
/**
* Returns parameters.
*
* @return parameters
*/
public Map getParameters() {
return parameters;
}
/**
* Returns result.
*
* @return result
*/
public Map getResult() {
return result;
}
/**
* Returns start timestamp.
*
* @return start timestamp
*/
public Date getStartTimestamp() {
return startTimestamp;
}
/**
* Returns end timestamp.
*
* @return end timestamp
*/
public Date getEndTimestamp() {
return endTimestamp;
}
/**
* Returns task duration in milliseconds.
*
* @return task duration
*/
public long getDuration() {
return endTimestamp.getTime() - startTimestamp.getTime();
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
@Override
public boolean equals(Object obj) {
return EqualsBuilder.reflectionEquals(this, obj);
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy