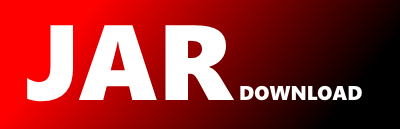
com.enterprisemath.utils.messaging.Attachment Maven / Gradle / Ivy
package com.enterprisemath.utils.messaging;
import com.enterprisemath.utils.ValidationUtils;
import java.io.ByteArrayInputStream;
import java.io.InputStream;
import java.util.Arrays;
import org.apache.commons.lang3.builder.EqualsBuilder;
import org.apache.commons.lang3.builder.HashCodeBuilder;
import org.apache.commons.lang3.builder.ToStringBuilder;
/**
* Message attachment.
*
* @author radek.hecl
*/
public class Attachment {
/**
* Builder object.
*/
public static class Builder {
/**
* Data content.
*/
private byte[] buf;
/**
* File name.
*/
private String name;
/**
* File MIME type.
*/
private String mime;
/**
* Sets data buffer.
*
* @param buf data buffer
* @return this instance
*/
public Builder setBuf(byte[] buf) {
this.buf = Arrays.copyOf(buf, buf.length);
return this;
}
/**
* Sets attachment name.
*
* @param name attachment name
* @return this instance
*/
public Builder setName(String name) {
this.name = name;
return this;
}
/**
* Sets attachment MIME type.
*
* @param mime MIME type
* @return this instance
*/
public Builder setMime(String mime) {
this.mime = mime;
return this;
}
/**
* Builds the object.
*
* @return created object
*/
public Attachment build() {
return new Attachment(this);
}
}
/**
* Data content.
*/
private byte[] buf;
/**
* File name.
*/
private String name;
/**
* File MIME type.
*/
private String mime;
/**
* Creates new object.
*
* @param builder builder object
*/
public Attachment(Builder builder) {
buf = Arrays.copyOf(builder.buf, builder.buf.length);
name = builder.name;
mime = builder.mime;
guardInvariants();
}
/**
* Guards this object to be consistent. Throws exception if this is not the case.s
*/
private void guardInvariants() {
ValidationUtils.guardNotNull(buf, "buffer cannot be null");
ValidationUtils.guardNotEmpty(name, "name cannot be empty");
ValidationUtils.guardNotNull(mime, "mime cannot be null");
}
/**
* Returns data buffer.
*
* @return data buffer
*/
public byte[] getBuf() {
return Arrays.copyOf(buf, buf.length);
}
/**
* Returns name.
*
* @return name
*/
public String getName() {
return name;
}
/**
* Returns mime type.
*
* @return
*/
public String getMime() {
return mime;
}
@Override
public int hashCode() {
return HashCodeBuilder.reflectionHashCode(this);
}
@Override
public boolean equals(Object obj) {
return EqualsBuilder.reflectionEquals(this, obj);
}
@Override
public String toString() {
return ToStringBuilder.reflectionToString(this);
}
/**
* Creates new attachment.
*
* @param name name
* @param mime mime type
* @param buf data buffer
* @return attachment
*/
public static Attachment create(String name, String mime, byte[] buf) {
return new Attachment.Builder().
setName(name).
setMime(mime).
setBuf(buf).
build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy