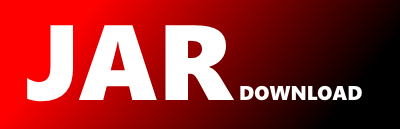
com.envision.event.rule.EventRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of eos-event-api-new Show documentation
Show all versions of eos-event-api-new Show documentation
1.0 初版
1.1 增加了event的flag和custom_attr字段。
The newest version!
package com.envision.event.rule;
import com.envision.event.bean.DataPoint;
import java.util.Date;
/**
* 事件规则对象基类
*
* @author kang.ouyang
*
*/
public abstract class EventRule {
/**
* id
*/
protected int id;
protected String category;
protected String tag;
/**
* domain:领域点,device:设备点
*/
protected String objectType;
/**
* objectType=device,objectId为设备id objectType=domain,objectId为domain id
*/
protected String objectId;
/**
* pointId,比如"INV.FaultCode"
*/
protected String pointId;
/**
* 客户作用域:'*': 全局
*/
protected String customerId = "*";
/**
* 站点作用域:'*': 客户下全部站点
*/
protected String siteId = "*";
/**
* 设备作用域:'*': 站点下全部设备
*/
protected String deviceId = "*";
/**
* 告警类型
*/
protected String alarmTypeId;
/**
* 告警子类型
*/
protected String childAlarmTypeId;
/**
* 规则触发的状态,亦即告警内容
*/
protected String status;
/**
* 规则优先级,对应告警等级
*/
protected String rank;
/**
* 0:告警事件,1:告警恢复事件,其他值:普通事件
*/
protected int eventType;
/**
* 0:规则暂不执行 1:实时触发 2 延时触发 4:脚本触发,默认是实时触发
*/
protected String ruleType;
/**
* 更新时间
*/
protected Date updateTime;
/**
* 规则描述
*/
protected String description;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getCategory() {
return category;
}
public void setCategory(String category) {
this.category = category;
}
public String getTag() {
return tag;
}
public void setTag(String tag) {
this.tag = tag;
}
public String getObjectType() {
return objectType;
}
public void setObjectType(String objectType) {
this.objectType = objectType;
}
public String getObjectId() {
return objectId;
}
public void setObjectId(String objectId) {
this.objectId = objectId;
}
public String getPointId() {
return pointId;
}
public void setPointId(String pointId) {
this.pointId = pointId;
}
public String getCustomerId() {
return customerId;
}
public void setCustomerId(String customerId) {
this.customerId = customerId;
}
public String getSiteId() {
return siteId;
}
public void setSiteId(String siteId) {
this.siteId = siteId;
}
public String getDeviceId() {
return deviceId;
}
public void setDeviceId(String deviceId) {
this.deviceId = deviceId;
}
public String getAlarmTypeId() {
return alarmTypeId;
}
public void setAlarmTypeId(String alarmTypeId) {
this.alarmTypeId = alarmTypeId;
}
public String getChildAlarmTypeId() {
return childAlarmTypeId;
}
public void setChildAlarmTypeId(String childAlarmTypeId) {
this.childAlarmTypeId = childAlarmTypeId;
}
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
public String getRank() {
return rank;
}
public void setRank(String rank) {
this.rank = rank;
}
public int getEventType() {
return eventType;
}
public void setEventType(int eventType) {
this.eventType = eventType;
}
public String getRuleType() {
return ruleType;
}
public void setRuleType(String ruleType) {
this.ruleType = ruleType;
}
public Date getUpdateTime() {
return updateTime;
}
public void setUpdateTime(Date updateTime) {
this.updateTime = updateTime;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
/**
* 判断一个数据点是否满足这条规则
*
* @param point
* @return
*/
public abstract boolean accept(DataPoint point);
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
builder.append("id: ").append(id).append(" category: ").append(category).append(" tag: ").append(tag).append(" objectType: ").append(objectType)
.append(" objectId: ").append(objectId).append(" pointId: ").append(pointId).append(" customerId: ").append(customerId).append(" siteId: ")
.append(siteId).append(" deviceId: ").append(deviceId).append(" alarmType :").append(alarmTypeId).append(" childAlarmType: ").append(childAlarmTypeId)
.append(" status: ").append(status).append(" rank: ").append(rank).append(" eventType: ").append(eventType).append(" ruleType:").append(ruleType)
.append(" updateTime: ").append(updateTime).append(" description: ").append(description);
return builder.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy