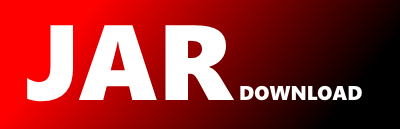
com.envisioniot.enos.enosapi.common.request.EnOSRequest Maven / Gradle / Ivy
package com.envisioniot.enos.enosapi.common.request;
import com.envisioniot.enos.enosapi.common.exception.EnOSClientException;
import com.envisioniot.enos.enosapi.common.exception.EnOSRuleException;
import com.envisioniot.enos.enosapi.common.util.*;
import com.envisioniot.enos.enosapi.common.response.EnOSResponse;
import java.lang.annotation.Annotation;
import java.lang.reflect.Field;
import java.lang.reflect.Modifier;
import java.lang.reflect.Type;
import java.util.Map;
/**
* @Description: EnOS_API_V2的request请求接口
*/
public abstract class EnOSRequest {
public abstract String getRequestMethod();
public abstract String getApiMethodName();
/**
* @return java.util.Map
* @Description 获取Request中放在url中的基本类型参数, 不包括PathVariable
**/
public Map getUrlParams() {
EnOSHashMap urlParams = new EnOSHashMap();
Field[] fields = this.getClass().getDeclaredFields();
if (fields != null) {
for (Field field : fields) {
field.setAccessible(true);//防止私有变量访问异常
if (Modifier.isStatic(field.getModifiers())) {
continue;// 不传输静态变量
}
try {
String fieldName = field.getName();
Object fieldValue = field.get(this);
Class fieldType = field.getType();
String fieldTypeName = fieldType.getSimpleName();
boolean isPathVariable = field.getAnnotation(EnOSPathVariable.class) != null;
if ("API_METHOD".equals(fieldName)) {
continue;//不传输API_METHOD字段
}
if (fieldValue == null) {
continue;
}
if (isPathVariable) {
continue;
}
if (TypeReflectUtils.isBasicType(fieldTypeName)) {
urlParams.put(fieldName, fieldValue);
}
} catch (IllegalAccessException e) {
e.printStackTrace();
}
}
}
return urlParams;
}
/**
* @return java.util.Map
* @Description 获取Request中放在url中的PathVariable(@EnOSPathVariable标注的变量)
**/
public Map getPathParams() {
EnOSHashMap pathParams = new EnOSHashMap();
Field[] fields = this.getClass().getDeclaredFields();
if (fields != null) {
for (Field field : fields) {
field.setAccessible(true);//防止私有变量访问异常
if (Modifier.isStatic(field.getModifiers())) {
continue;// 不传输静态变量
}
try {
String fieldName = field.getName();
Object fieldValue = field.get(this);
EnOSPathVariable pathVariableAnno = field.getAnnotation(EnOSPathVariable.class);
if (pathVariableAnno != null) {
String pathParamName = StringUtils.isEmpty(pathVariableAnno.name()) ? fieldName : pathVariableAnno.name();
pathParams.put(pathParamName, fieldValue);
}
} catch (IllegalAccessException e) {
e.printStackTrace();
}
}
}
return pathParams;
}
/**
* @return java.lang.String
* @throws EnOSClientException 当对象参数超过一个时,抛出此异常
* @Description 获取Request中放在JsonBody中的参数
**/
public String getJsonParam() throws EnOSClientException {
int jsonCount = 0;
String jsonParam = null;
Field[] fields = this.getClass().getDeclaredFields();
if (fields != null) {
for (Field field : fields) {
field.setAccessible(true);//防止私有变量访问异常
if (Modifier.isStatic(field.getModifiers())) {
continue;// 不传输静态变量
}
try {
String fieldName = field.getName();
Object fieldValue = field.get(this);
Class fieldType = field.getType();
String fieldTypeName = fieldType.getSimpleName();
if (fieldValue == null) {
continue;
}
if (!TypeReflectUtils.isBasicType(fieldTypeName)) {
jsonCount++;
jsonParam = JsonParser.toJson(fieldValue);
if (jsonCount >= 2) {
throw new EnOSClientException(EnOSErrorCode.INVALID_ARGUMENT, "only support one json body");
}
}
} catch (IllegalAccessException e) {
e.printStackTrace();
}
}
}
return jsonParam;
}
/**
* @return java.lang.reflect.Type 当前实例的泛型类型
* @Description 获取当前Request实例的泛型类型(T extends EnOSResponse)
**/
public Type getResponseType() {
return TypeReflectUtils.getActualGenricType(this.getClass(), 0);
}
public abstract void check() throws EnOSRuleException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy