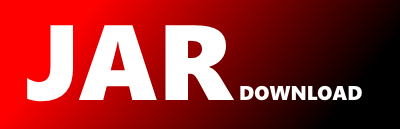
com.epam.eco.commons.kafka.ScalaConversions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of commons-kafka Show documentation
Show all versions of commons-kafka Show documentation
A library of utilities, helpers and higher-level APIs for the
Kafka client library
/*
* Copyright 2019 EPAM Systems
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy
* of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*/
package com.epam.eco.commons.kafka;
import java.util.Arrays;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.Properties;
import java.util.Set;
import org.apache.commons.lang3.Validate;
import org.apache.kafka.common.ConsumerGroupState;
import org.apache.kafka.common.TopicPartition;
import org.apache.kafka.common.resource.PatternType;
import kafka.coordinator.group.GroupState;
import kafka.coordinator.group.GroupTopicPartition;
import kafka.security.auth.Resource;
import kafka.utils.VerifiableProperties;
import scala.Option;
import scala.collection.JavaConverters;
import scala.collection.Seq;
/**
* @author Andrei_Tytsik
*/
public abstract class ScalaConversions {
private ScalaConversions() {
}
@SuppressWarnings("unchecked")
public static Seq asScalaSeq(T ... values) {
return asScalaSeq(Arrays.asList(values));
}
public static Seq asScalaSeq(List list) {
return JavaConverters.asScalaBufferConverter(list).asScala();
}
public static scala.collection.Set asScalaSet(Set set) {
return JavaConverters.asScalaSetConverter(set).asScala();
}
public static List asJavaList(Seq seq) {
return JavaConverters.seqAsJavaListConverter(seq).asJava();
}
public static Set asJavaSet(scala.collection.Set set) {
return new HashSet<>(JavaConverters.setAsJavaSetConverter(set).asJava());
}
public static Map asJavaMap(scala.collection.Map scalaMap) {
return JavaConverters.mapAsJavaMapConverter(scalaMap).asJava();
}
public static TopicPartition asTopicPartition(GroupTopicPartition groupTopicPartition) {
Validate.notNull(groupTopicPartition, "GroupTopicPartition is null");
return new TopicPartition(
groupTopicPartition.topicPartition().topic(),
groupTopicPartition.topicPartition().partition());
}
public static Optional asOptional(Option option) {
Validate.notNull(option, "Option is null");
return Optional.ofNullable(!option.isEmpty() ? option.get() : null);
}
public static VerifiableProperties asVerifiableProperties(Properties properties) {
Validate.notNull(properties, "Properties object is null");
return new VerifiableProperties(properties);
}
public static VerifiableProperties asVerifiableProperties(Map properties) {
Validate.notNull(properties, "Properties map is null");
Properties propertiesTmp = new Properties();
propertiesTmp.putAll(properties);
return new VerifiableProperties(propertiesTmp);
}
public static kafka.security.auth.Resource asScalaResource(
org.apache.kafka.common.resource.ResourceType resourceType,
String resourceName) {
Validate.notNull(resourceType, "Resource type is null");
Validate.notBlank(resourceName, "Resource name is blank");
return new Resource(
asScalaResourceType(resourceType),
resourceName,
PatternType.LITERAL);
}
public static kafka.security.auth.Resource asScalaResource(
org.apache.kafka.common.resource.ResourceType resourceType,
String resourceName,
PatternType patternType) {
Validate.notNull(resourceType, "Resource type is null");
Validate.notBlank(resourceName, "Resource name is blank");
Validate.notNull(patternType, "Pattern type is null");
return new Resource(
asScalaResourceType(resourceType),
resourceName,
patternType);
}
public static kafka.security.auth.ResourceType asScalaResourceType(
org.apache.kafka.common.resource.ResourceType resourceType) {
Validate.notNull(resourceType, "Resource type is null");
return kafka.security.auth.ResourceType$.MODULE$.fromJava(resourceType);
}
public static ConsumerGroupState asJavaGroupState(GroupState groupState) {
Validate.notNull(groupState, "Group state is null");
return ConsumerGroupState.parse(groupState.toString());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy