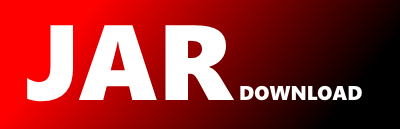
com.epam.jdi.light.ui.html.asserts.NumberAssert Maven / Gradle / Ivy
package com.epam.jdi.light.ui.html.asserts;
import com.epam.jdi.light.asserts.generic.ITextAssert;
import com.epam.jdi.light.asserts.generic.UIAssert;
import com.epam.jdi.light.common.JDIAction;
import com.epam.jdi.light.ui.html.elements.common.NumberSelector;
import org.hamcrest.Matcher;
import org.hamcrest.Matchers;
import static com.epam.jdi.light.asserts.core.SoftAssert.jdiAssert;
import static com.epam.jdi.light.ui.html.HtmlUtils.getDouble;
/**
* Created by Roman Iovlev on 14.02.2018
* Email: [email protected]; Skype: roman.iovlev
*/
public class NumberAssert extends UIAssert
implements ITextAssert {
@JDIAction("Assert that '{name}' text {0}")
public NumberAssert text(Matcher condition) {
jdiAssert(element().attr("value"), condition);
return this;
}
@JDIAction("Assert that '{name}' minValue {0}")
public NumberAssert min(Matcher min) {
jdiAssert(element().min(), min);
return this;
}
public NumberAssert min(double min) { return min(Matchers.is(min)); }
@JDIAction("Assert that '{name}' maxValue {0}")
public NumberAssert max(Matcher max) {
jdiAssert(element().max(), max);
return this;
}
public NumberAssert max(double max) { return max(Matchers.is(max)); }
@JDIAction("Assert that '{name}' step {0}")
public NumberAssert step(Matcher step) {
jdiAssert(element().step(), step);
return this;
}
public NumberAssert step(double step) { return step(Matchers.is(step)); }
@JDIAction("Assert that '{name}' placeholder {0}")
public NumberAssert placeholder(Matcher placeholder) {
jdiAssert(element().placeholder(), placeholder);
return this;
}
public NumberAssert placeholder(String placeholder) { return placeholder(Matchers.is(placeholder)); }
@JDIAction("Assert that '{name}' number {0}")
public NumberAssert number(Matcher number) {
jdiAssert(getDouble(element().value()), number);
return this;
}
public NumberAssert number(double number) { return number(Matchers.is(number)); }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy