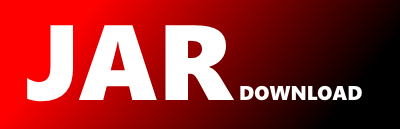
com.epam.jdi.uitests.web.selenium.elements.actions.ElementsActions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jdi-uitest-web Show documentation
Show all versions of jdi-uitest-web Show documentation
Epam UI Automation framework package for Web
package com.epam.jdi.uitests.web.selenium.elements.actions;
/*
* Copyright 2004-2016 EPAM Systems
*
* This file is part of JDI project.
*
* JDI is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* JDI is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY;
* without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with JDI. If not, see .
*/
import com.epam.commons.linqinterfaces.JAction;
import com.epam.commons.linqinterfaces.JFuncTREx;
import com.epam.jdi.uitests.web.selenium.elements.base.BaseElement;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
import static com.epam.commons.LinqUtils.where;
import static com.epam.commons.PrintUtils.print;
import static com.epam.commons.Timer.getByCondition;
import static com.epam.jdi.uitests.core.settings.JDISettings.asserter;
import static java.lang.String.format;
import static java.lang.String.join;
/**
* Created by Roman_Iovlev on 9/3/2015.
*/
public class ElementsActions {
private BaseElement element;
public ElementsActions(BaseElement element) {
this.element = element;
}
public ActionInvoker invoker() {
return element.invoker;
}
// Element Actions
public boolean isDisplayed(Supplier isDisplayed) {
return invoker().doJActionResult("Is element displayed", isDisplayed);
}
public boolean isHidden(Supplier isHidden) {
return invoker().doJActionResult("Is element hidden", isHidden);
}
public void waitDisplayed(Supplier isDisplayed) {
invoker().doJActionResult("Wait element displayed", isDisplayed);
}
public void waitVanished(Supplier isVanished) {
invoker().doJActionResult("Wait element vanished", isVanished);
}
// Value Actions
public String getValue(Supplier getValueFunc) {
return invoker().doJActionResult("Get value", getValueFunc);
}
public void setValue(String value, Consumer setValueAction) {
invoker().doJAction("Set value", () -> setValueAction.accept(value));
}
// Click Action
public void click(JAction clickAction) {
invoker().doJAction("Click on Element", clickAction);
}
// Text Actions
public String getText(Supplier getTextAction) {
return invoker().doJActionResult("Get text", getTextAction);
}
public String waitText(String text, Supplier getTextAction) {
return invoker().doJActionResult(format("Wait text contains '%s'", text),
() -> getByCondition(getTextAction, t -> t.contains(text)));
}
public String waitMatchText(String regEx, Supplier getTextAction) {
return invoker().doJActionResult(format("Wait text match regex '%s'", regEx),
() -> getByCondition(getTextAction, t -> t.matches(regEx)));
}
// Check/Select Actions
public boolean isSelected(Supplier isSelectedAction) {
return invoker().doJActionResult("Is Selected", isSelectedAction);
}
public void check(JAction checkAction) {
invoker().doJAction("Check Checkbox", checkAction);
}
public void uncheck(JAction uncheckAction) {
invoker().doJAction("Uncheck Checkbox", uncheckAction);
}
public boolean isChecked(Supplier isCheckedAction) {
return invoker().doJActionResult("IsChecked",
isCheckedAction,
result -> "Checkbox is " + (result ? "checked" : "unchecked"));
}
// Input Actions
public void inputLines(JAction clearAction, Consumer inputAction, String... textLines) {
invoker().doJAction("Input several lines of text in textarea",
() -> {
clearAction.invoke();
inputAction.accept(join("\n", textLines));
});
}
public void addNewLine(String textLine, Consumer inputAction) {
invoker().doJAction("Add text from new line in textarea",
() -> inputAction.accept("\n" + textLine));
}
public String[] getLines(Supplier getTextAction) {
return invoker().doJActionResult("Get text as lines", () -> getTextAction.get().split("\\n"));
}
public void input(CharSequence text, Consumer inputAction) {
invoker().doJAction("Input text '" + text + "' in text field",
() -> inputAction.accept(text));
}
public void clear(JAction clearAction) {
invoker().doJAction("Clear text field", clearAction);
}
public void focus(JAction focusAction) {
invoker().doJAction("Focus on text field", focusAction);
}
// Selector
public void select(String name, Consumer selectAction) {
invoker().doJAction(format("Select '%s'", name), () -> selectAction.accept(name));
}
public void select(int index, Consumer selectByIndexAction) {
invoker().doJAction(format("Select '%s'", index), () -> selectByIndexAction.accept(index));
}
public void hover(String name, Consumer hoverAction) {
invoker().doJAction(format("Hover '%s'", name), () -> hoverAction.accept(name));
}
public boolean isSelected(String name, Function isSelectedAction) {
return invoker().doJActionResult(format("Wait is '%s' selected", name), () -> isSelectedAction.apply(name));
}
public String getSelected(Supplier isSelectedAction) {
return invoker().doJActionResult("Get Selected element name", isSelectedAction);
}
public int getSelectedIndex(Supplier isSelectedAction) {
return invoker().doJActionResult("Get Selected element index", isSelectedAction);
}
//MultiSelector
public void select(Consumer selectListAction, String... names) {
invoker().doJAction(String.format("Select '%s'", print(names)), () -> selectListAction.accept(names));
}
public void select(Consumer selectListAction, int[] indexes) {
List listIndexes = new ArrayList<>();
for (int i : indexes)
listIndexes.add(Integer.toString(i));
invoker().doJAction(String.format("Select '%s'", print(listIndexes)), () -> selectListAction.accept(indexes));
}
// Expand Action
public void expand(JAction expandAction) {
invoker().doJAction("Expand Element", expandAction);
}
public List areSelected(Supplier> getNames, JFuncTREx waitSelectedAction) {
return invoker().doJActionResult("Are selected", () ->
where(getNames.get(), waitSelectedAction));
}
public void waitSelected(Function waitSelectedAction, String... names) {
boolean result = invoker().doJActionResult(String.format("Are deselected '%s'", print(names)), () -> {
for (String name : names)
if (!waitSelectedAction.apply(name))
return false;
return true;
});
asserter.isTrue(result);
}
public List areDeselected(Supplier> getNames, Function waitSelectedAction) {
return invoker().doJActionResult("Are deselected", () ->
where(getNames.get(), name -> !waitSelectedAction.apply(name)));
}
public void waitDeselected(Function waitSelectedAction, String... names) {
boolean result = invoker().doJActionResult(String.format("Are deselected '%s'",
print(names)), () -> {
for (String name : names)
if (waitSelectedAction.apply(name))
return false;
return true;
});
asserter.isTrue(result);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy