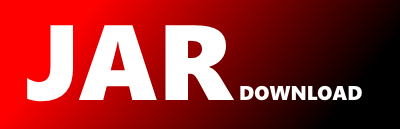
com.epam.jdi.uitests.web.selenium.elements.apiInteract.GetElementModule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jdi-uitest-web Show documentation
Show all versions of jdi-uitest-web Show documentation
Epam UI Automation framework package for Web
package com.epam.jdi.uitests.web.selenium.elements.apiInteract;
/*
* Copyright 2004-2016 EPAM Systems
*
* This file is part of JDI project.
*
* JDI is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* JDI is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY;
* without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the GNU Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with JDI. If not, see .
*/
import com.epam.commons.Timer;
import com.epam.commons.linqinterfaces.JFuncTREx;
import com.epam.jdi.uitests.core.interfaces.base.IAvatar;
import com.epam.jdi.uitests.core.interfaces.base.IBaseElement;
import com.epam.jdi.uitests.web.selenium.elements.base.BaseElement;
import com.epam.jdi.uitests.web.selenium.elements.base.Element;
import org.openqa.selenium.By;
import org.openqa.selenium.SearchContext;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.function.Supplier;
import static com.epam.commons.LinqUtils.any;
import static com.epam.commons.LinqUtils.where;
import static com.epam.commons.ReflectionUtils.isClass;
import static com.epam.jdi.uitests.core.settings.JDISettings.*;
import static com.epam.jdi.uitests.web.selenium.driver.SeleniumDriverFactory.elementSearchCriteria;
import static com.epam.jdi.uitests.web.selenium.driver.SeleniumDriverFactory.onlyOneElementAllowedInSearch;
import static com.epam.jdi.uitests.web.selenium.driver.WebDriverByUtils.*;
import static java.lang.String.format;
/**
* Created by Roman_Iovlev on 7/3/2015.
*/
public class GetElementModule implements IAvatar {
private static final String FAILED_TO_FIND_ELEMENT_MESSAGE
= "Can't find Element '%s' during %s seconds";
private static final String FIND_TO_MUCH_ELEMENTS_MESSAGE
= "Find %s elements instead of one for Element '%s' during %s seconds";
private By byLocator;
public By frameLocator;
public JFuncTREx localElementSearchCriteria = null;
private String driverName = "";
private BaseElement element;
private WebElement webElement;
private List webElements;
public void setLocator(By locator) {
byLocator = locator;
}
public GetElementModule(BaseElement element) {
this.element = element;
if (driverName.equals(""))
driverName = driverFactory.currentDriverName();
}
public GetElementModule(By byLocator, BaseElement element) {
this(element);
this.byLocator = byLocator;
}
public GetElementModule copy() {
return copy(byLocator);
}
public GetElementModule copy(By byLocator) {
GetElementModule clone = new GetElementModule(byLocator, element);
clone.localElementSearchCriteria = localElementSearchCriteria;
clone.frameLocator = frameLocator;
clone.driverName = driverName;
clone.element = element;
clone.webElement = webElement;
clone.webElements = webElements;
return clone;
}
public boolean hasLocator() {
return byLocator != null;
}
public By getLocator() { return byLocator; }
public void setWebElement(WebElement webElement) { this.webElement = webElement; }
public boolean hasWebElement() { if (webElement == null) return false; try { webElement.getTagName(); return true; } catch (Exception ex) {return false; } }
public WebDriver getDriver() {
return (WebDriver) driverFactory.getDriver(driverName);
}
public String getDriverName() {
return driverName;
}
public void setDriverName(String driverName) { this.driverName = driverName; }
public WebElement getElement() {
logger.debug("Get Web Element: " + element);
WebElement element = hasWebElement()
? webElement
: getElementAction();
logger.debug("One Element found");
return element;
}
public List getElements() {
logger.debug("Get Web elements: " + element);
List elements = getElementsAction();
logger.debug("Found %s elements", elements.size());
return elements;
}
public T findImmediately(Supplier func, T ifError) {
element.setWaitTimeout(0);
JFuncTREx temp = localElementSearchCriteria;
localElementSearchCriteria = el -> true;
T result;
try {
result = func.get();
} catch (Exception | Error ex) {
result = ifError;
}
localElementSearchCriteria = temp;
element.restoreWaitTimeout();
return result;
}
public Timer timer(int sec) {
return new Timer(sec * 1000);
}
public Timer timer() {
return timer(timeouts.getCurrentTimeoutSec());
}
private List getElementsByCondition(JFuncTREx condition) {
List elements = timer().getResultByCondition(
this::searchElements,
els -> any(els, getSearchCriteria()));
if (elements == null || elements.size() == 0)
return new ArrayList<>();
return elements.size() < 10 ? where(elements, condition) : elements;
}
private List getElementsAction() {
if (webElements != null)
return webElements;
List result = getElementsByCondition(getSearchCriteria());
timeouts.dropTimeouts();
if (result == null)
throw exception("Can't get Web Elements");
return result;
}
private JFuncTREx getSearchCriteria() {
return localElementSearchCriteria != null ? localElementSearchCriteria : elementSearchCriteria;
}
public GetElementModule searchAll() {
localElementSearchCriteria = Objects::nonNull;
return this;
}
private List getOneOrMoreElements() {
List result = hasWebElement()
? webElements
: searchElements();
if (result.size() == 1)
return result;
return where(result, el -> getSearchCriteria().invoke(el));
}
private WebElement getElementAction() {
int timeout = timeouts.getCurrentTimeoutSec();
List result = getOneOrMoreElements();
switch (result.size()) {
case 0:
throw exception(FAILED_TO_FIND_ELEMENT_MESSAGE, element, timeout);
case 1:
return result.get(0);
default:
if (onlyOneElementAllowedInSearch)
throw exception(FIND_TO_MUCH_ELEMENTS_MESSAGE, result.size(), element, timeout);
return result.get(0);
}
}
private SearchContext getSearchContext(Object element) {
if (element == null || !isClass(element.getClass(), BaseElement.class))
return getDefaultContext();
BaseElement bElement = (BaseElement) element;
if (bElement.useCache && isClass(bElement.getClass(), Element.class)) {
Element el = (Element) bElement;
if (el.avatar.hasWebElement())
return el.avatar.webElement;
}
Object p = bElement.getParent();
By locator = bElement.getLocator();
By frame = bElement.avatar.frameLocator;
SearchContext searchContext = frame != null
? getFrameContext(frame)
: p == null || containsRoot(locator)
? getDefaultContext()
: getSearchContext(p);
return locator != null
? searchContext.findElement(correctLocator(locator))
: searchContext;
}
private SearchContext getDefaultContext() {
return getDriver().switchTo().defaultContent();
}
private SearchContext getFrameContext(By frame) {
return getDriver().switchTo().frame(getDriver().findElement(frame));
}
private List searchElements() {
SearchContext searchContext = getDefaultContext();
if (!containsRoot(getLocator()))
searchContext = getSearchContext(element.getParent());
return searchContext.findElements(correctLocator(getLocator()));
}
private By correctLocator(By locator) {
if (locator == null) return null;
return correctXPaths(containsRoot(locator)
? trimRoot(locator)
: locator);
}
public void clearCookies() {
getDriver().manage().deleteAllCookies();
}
@Override
public String toString() {
if (hasWebElement()) return "Has WebElement";
return shortLogMessagesFormat
? printFullLocator()
: format("Locator: '%s'", getLocator())
+ (element.getParent() != null && isClass(element.getParent().getClass(), IBaseElement.class)
? format(", Context: '%s'", element.printContext())
: "");
}
private String printFullLocator() {
if (!hasLocator())
return "No Locators";
return element.printContext() + "; " + printShortBy(getLocator());
}
private String printShortBy(By by) {
return String.format("%s='%s'", getByName(by), getByLocator(by));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy