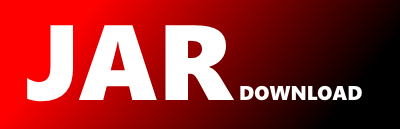
com.equinix.pulumi.metal.Project Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of equinix Show documentation
Show all versions of equinix Show documentation
A Pulumi package for creating and managing equinix cloud resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.equinix.pulumi.metal;
import com.equinix.pulumi.Utilities;
import com.equinix.pulumi.metal.ProjectArgs;
import com.equinix.pulumi.metal.inputs.ProjectState;
import com.equinix.pulumi.metal.outputs.ProjectBgpConfig;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an Equinix Metal project resource to allow you manage devices in your projects.
*
* > **NOTE:** Keep in mind that Equinix Metal invoicing is per project, so creating many `equinix.metal.Project` resources will affect the rendered invoice. If you want to keep your Equinix Metal bill simple and easy to review, please re-use your existing projects.
*
* ## Example Usage
* ### example 1
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.equinix.metal.Project;
* import com.pulumi.equinix.metal.ProjectArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var tfProject1 = new Project("tfProject1", ProjectArgs.builder()
* .name("Terraform Fun")
* .build());
*
* }
* }
* }
*
* ### example 2
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.equinix.metal.Project;
* import com.pulumi.equinix.metal.ProjectArgs;
* import com.pulumi.equinix.metal.inputs.ProjectBgpConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var tfProject1 = new Project("tfProject1", ProjectArgs.builder()
* .name("tftest")
* .bgpConfig(ProjectBgpConfigArgs.builder()
* .deploymentType("local")
* .md5("C179c28c41a85b")
* .asn(65000)
* .build())
* .build());
*
* }
* }
* }
*
* ### example 3
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.equinix.metal.Project;
* import com.pulumi.equinix.metal.ProjectArgs;
* import com.pulumi.equinix.metal.inputs.ProjectBgpConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var existingProject = new Project("existingProject", ProjectArgs.builder()
* .name("The name of the project (if different, will rewrite)")
* .bgpConfig(ProjectBgpConfigArgs.builder()
* .deploymentType("local")
* .md5("C179c28c41a85b")
* .asn(65000)
* .build())
* .build());
*
* }
* }
* }
*
*
* ## Import
*
* This resource can be imported using an existing project ID:
*
* ```sh
* $ pulumi import equinix:metal/project:Project equinix_metal_project {existing_project_id}
* ```
*
*/
@ResourceType(type="equinix:metal/project:Project")
public class Project extends com.pulumi.resources.CustomResource {
/**
* Enable or disable [Backend Transfer](https://metal.equinix.com/developers/docs/networking/backend-transfer/), default is `false`.
*
*/
@Export(name="backendTransfer", refs={Boolean.class}, tree="[0]")
private Output backendTransfer;
/**
* @return Enable or disable [Backend Transfer](https://metal.equinix.com/developers/docs/networking/backend-transfer/), default is `false`.
*
*/
public Output backendTransfer() {
return this.backendTransfer;
}
/**
* Optional BGP settings. Refer to [Equinix Metal guide for BGP](https://metal.equinix.com/developers/docs/networking/local-global-bgp/).
*
* > **NOTE:** Once you set the BGP config in a project, it can't be removed (due to a limitation in the Equinix Metal API). It can be updated.
*
*/
@Export(name="bgpConfig", refs={ProjectBgpConfig.class}, tree="[0]")
private Output* @Nullable */ ProjectBgpConfig> bgpConfig;
/**
* @return Optional BGP settings. Refer to [Equinix Metal guide for BGP](https://metal.equinix.com/developers/docs/networking/local-global-bgp/).
*
* > **NOTE:** Once you set the BGP config in a project, it can't be removed (due to a limitation in the Equinix Metal API). It can be updated.
*
*/
public Output> bgpConfig() {
return Codegen.optional(this.bgpConfig);
}
/**
* The timestamp for when the project was created.
*
*/
@Export(name="created", refs={String.class}, tree="[0]")
private Output created;
/**
* @return The timestamp for when the project was created.
*
*/
public Output created() {
return this.created;
}
/**
* The name of the project. The maximum length is 80 characters
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the project. The maximum length is 80 characters
*
*/
public Output name() {
return this.name;
}
/**
* The UUID of organization under which you want to create the project. If you leave it out, the project will be created under your the default organization of your account.
*
*/
@Export(name="organizationId", refs={String.class}, tree="[0]")
private Output organizationId;
/**
* @return The UUID of organization under which you want to create the project. If you leave it out, the project will be created under your the default organization of your account.
*
*/
public Output organizationId() {
return this.organizationId;
}
/**
* The UUID of payment method for this project. The payment method and the project need to belong to the same organization (passed with `organization_id`, or default).
*
*/
@Export(name="paymentMethodId", refs={String.class}, tree="[0]")
private Output paymentMethodId;
/**
* @return The UUID of payment method for this project. The payment method and the project need to belong to the same organization (passed with `organization_id`, or default).
*
*/
public Output paymentMethodId() {
return this.paymentMethodId;
}
/**
* The timestamp for the last time the project was updated.
*
*/
@Export(name="updated", refs={String.class}, tree="[0]")
private Output updated;
/**
* @return The timestamp for the last time the project was updated.
*
*/
public Output updated() {
return this.updated;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Project(java.lang.String name) {
this(name, ProjectArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Project(java.lang.String name, @Nullable ProjectArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Project(java.lang.String name, @Nullable ProjectArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("equinix:metal/project:Project", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Project(java.lang.String name, Output id, @Nullable ProjectState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("equinix:metal/project:Project", name, state, makeResourceOptions(options, id), false);
}
private static ProjectArgs makeArgs(@Nullable ProjectArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ProjectArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Project get(java.lang.String name, Output id, @Nullable ProjectState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Project(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy