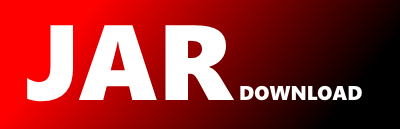
com.erigir.wrench.shiro.OauthPrincipal Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of wrench-shiro-oauth Show documentation
Show all versions of wrench-shiro-oauth Show documentation
An extensible implementation of Apache Shiro that uses OAuth as its source with a provided
Spring MVC configuration
package com.erigir.wrench.shiro;
import org.apache.shiro.SecurityUtils;
import org.apache.shiro.subject.Subject;
import java.util.Collection;
import java.util.Collections;
import java.util.Map;
import java.util.Set;
import java.util.TreeMap;
import java.util.TreeSet;
/**
* Class for shared functionality between various OAuthPrincipal providers.
*
* Note that this class holds a set of roles and permissions - while this
* isn't exactly how Shiro sees the use, I put them in here because:
* A) I have to put them somewhere to allow you to modify them since they don't come from the oauth provider
* B) Shiro doesn't have the ability to enumerate them out of the AuthorizationInfo class, just check existence
*
* B is the main reason - sometimes I need to enumerate, and Shiro doesnt have it (at least in version 1.2.3)
*
* Created by chrweiss on 5/29/15.
*/
public class OauthPrincipal {
private Map otherData = new TreeMap<>();
private String oauthProviderName;
private Set roles = new TreeSet<>();
private Set permissions = new TreeSet<>();
public Map getOtherData() {
return otherData;
}
public void setOtherData(Map otherData) {
this.otherData = otherData;
}
public String getOauthProviderName() {
return oauthProviderName;
}
public void setOauthProviderName(String oauthProviderName) {
this.oauthProviderName = oauthProviderName;
}
public Set getRoles() {
return roles;
}
public void setRoles(Set roles) {
this.roles = roles;
}
public Set getPermissions() {
return permissions;
}
public void setPermissions(Set permissions) {
this.permissions = permissions;
}
/**
* Helper method to extract role list from oauth principals in current session
*
* @return Set of strings containing all roles
*/
public static Set oauthRoles() {
Set rval = new TreeSet<>();
for (OauthPrincipal p : oauthPrincipals()) {
rval.addAll(p.getRoles());
}
return rval;
}
/**
* Helper method to extract role list from oauth principals in current session
*
* @return Set of strings containing all permissions
*/
public static Set oauthPermissions() {
Set rval = new TreeSet<>();
for (OauthPrincipal p : oauthPrincipals()) {
rval.addAll(p.getPermissions());
}
return rval;
}
public static OauthPrincipal firstOauthPrincipal() {
Collection c = oauthPrincipals();
return (c.isEmpty()) ? null : c.iterator().next();
}
public static Collection oauthPrincipals() {
Subject subject = SecurityUtils.getSubject();
return (subject == null) ? Collections.EMPTY_LIST : subject.getPrincipals().byType(OauthPrincipal.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy