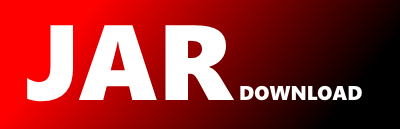
main.java.org.compiere.request.process.RequestInvoice Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of request Show documentation
Show all versions of request Show documentation
Request Management, used to CRM request.
/******************************************************************************
* Product: Adempiere ERP & CRM Smart Business Solution *
* Copyright (C) 1999-2006 ComPiere, Inc. All Rights Reserved. *
* This program is free software; you can redistribute it and/or modify it *
* under the terms version 2 of the GNU General Public License as published *
* by the Free Software Foundation. This program is distributed in the hope *
* that it will be useful, but WITHOUT ANY WARRANTY; without even the implied *
* warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. *
* See the GNU General Public License for more details. *
* You should have received a copy of the GNU General Public License along *
* with this program; if not, write to the Free Software Foundation, Inc., *
* 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA. *
* For the text or an alternative of this public license, you may reach us *
* ComPiere, Inc., 2620 Augustine Dr. #245, Santa Clara, CA 95054, USA *
* or via [email protected] or http://www.compiere.org/license.html *
*****************************************************************************/
package org.compiere.request.process;
import org.compiere.model.MBPartner;
import org.compiere.model.MInvoice;
import org.compiere.model.MInvoiceLine;
import org.compiere.model.MRequest;
import org.compiere.model.MRequestType;
import org.compiere.model.MRequestUpdate;
import org.compiere.model.MStatus;
import org.compiere.model.Query;
import org.compiere.util.AdempiereSystemError;
import org.compiere.util.Msg;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Optional;
import java.util.concurrent.atomic.AtomicInteger;
/**
* Create Invoices for Requests
*
*
* @author Jorg Janke
* @author [email protected] , Victor Perez , e-Evolution Consultant, S.A. www.e-evolution.com
* Add relation between Request Update and Invoice Line #2484
* https://github.com/adempiere/adempiere/issues/2484
*/
public class RequestInvoice extends RequestInvoiceAbstract {
private HashMap invoicesByPartner = new HashMap<>();
/**
* Prepare
*/
protected void prepare() {
super.prepare();
} // prepare
/**
* Process
* @return info
* @throws Exception
*/
protected String doIt() throws Exception {
log.info("R_RequestType_ID=" + getRequestTypeId() + ", R_Group_ID=" + getGroupId()
+ ", R_Category_ID=" + getCategoryId() + ", C_BPartner_ID=" + getBPartnerId()
+ ", p_M_Product_ID=" + getProductId());
validate();
getRequestsToInvoice().stream()
.forEach(request -> {
MInvoice invoice = Optional.ofNullable(invoicesByPartner.get(request.getC_BPartner_ID())).orElseGet(() -> invoiceNew(request));
invoiceLine(request, invoice);
});
invoicesByPartner.entrySet().stream().forEach(entry -> {
MInvoice invoice = entry.getValue();
invoice.processIt(MInvoice.ACTION_Prepare);
invoice.saveEx();
addLog(invoice.getDocumentInfo());
});
return "@Ok@";
} // doIt
private boolean validate() throws AdempiereSystemError {
MRequestType requestType = MRequestType.get(getCtx(), getRequestTypeId());
if (requestType.get_ID() == 0)
throw new AdempiereSystemError("@R_RequestType_ID@ @NotFound@ " + getRequestTypeId());
if (!requestType.isInvoiced())
throw new AdempiereSystemError("@R_RequestType_ID@ <> @IsInvoiced@");
return true;
}
private List getRequestsToInvoice() {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy