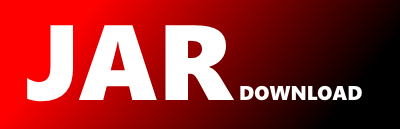
org.adempiere.legacy.apache.ecs.xhtml.pre Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tools Show documentation
Show all versions of tools Show documentation
A tool used for all ADempiere project ans first base library.
/******************************************************************************
* Product: Adempiere ERP & CRM Smart Business Solution *
* Copyright (C) 1999-2006 ComPiere, Inc. All Rights Reserved. *
* This program is free software; you can redistribute it and/or modify it *
* under the terms version 2 of the GNU General Public License as published *
* by the Free Software Foundation. This program is distributed in the hope *
* that it will be useful, but WITHOUT ANY WARRANTY; without even the implied *
* warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. *
* See the GNU General Public License for more details. *
* You should have received a copy of the GNU General Public License along *
* with this program; if not, write to the Free Software Foundation, Inc., *
* 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA. *
* For the text or an alternative of this public license, you may reach us *
* ComPiere, Inc., 2620 Augustine Dr. #245, Santa Clara, CA 95054, USA *
* or via [email protected] or http://www.compiere.org/license.html *
*****************************************************************************/
package org.adempiere.legacy.apache.ecs.xhtml;
import org.adempiere.legacy.apache.ecs.Element;
import org.adempiere.legacy.apache.ecs.KeyEvents;
import org.adempiere.legacy.apache.ecs.MouseEvents;
import org.adempiere.legacy.apache.ecs.MultiPartElement;
/**
This class creates a <pre> tag.
@version $Id: pre.java,v 1.2 2006/07/30 00:54:02 jjanke Exp $
@author Stephan Nagy
@author Jon S. Stevens
@author Bojan Smojver
*/
public class pre extends MultiPartElement implements MouseEvents, KeyEvents
{
/**
*
*/
private static final long serialVersionUID = 2940107215887001778L;
/**
Private initialization routine.
*/
{
setElementType("pre");
setCase(LOWERCASE);
setAttributeQuote(true);
setPrettyPrint(false);
}
/**
Basic constructor. You need to set the attributes using the
set* methods.
*/
public pre()
{
}
/**
Use the set* methods to set the values
of the attributes.
@param element set the value of <pre>value</pre>
*/
public pre(Element element)
{
addElement(element);
}
/**
Use the set* methods to set the values
of the attributes.
@param value set the value of <pre>value</pre>
@param width set the value of width=""
*/
public pre(String value, int width)
{
setWidth(width);
addElement(value);
}
/**
Use the set* methods to set the values
of the attributes.
@param element set the value of <pre>value</pre>
@param width set the value of width=""
*/
public pre(Element element, int width)
{
setWidth(width);
addElement(element);
}
/**
Use the set* methods to set the values
of the attributes.
@param element set the value of <pre>value</pre>
@param width set the value of width=""
*/
public pre(Element element, String width)
{
setWidth(width);
addElement(element);
}
/**
Use the set* methods to set the values
of the attributes.
@param value set the value of <pre>value</pre>
*/
public pre(String value)
{
addElement(value);
}
/**
Sets the width="" attribute
@param width the width="" attribute
*/
public pre setWidth(int width)
{
addAttribute("width",Integer.toString(width));
return this;
}
/**
Sets the width="" attribute
@param width the width="" attribute
*/
public pre setWidth(String width)
{
addAttribute("width",width);
return this;
}
/**
Sets the lang="" and xml:lang="" attributes
@param lang the lang="" and xml:lang="" attributes
*/
public Element setLang(String lang)
{
addAttribute("lang",lang);
addAttribute("xml:lang",lang);
return this;
}
/**
Adds an Element to the element.
@param hashcode name of element for hash table
@param element Adds an Element to the element.
*/
public pre addElement(String hashcode,Element element)
{
addElementToRegistry(hashcode,element);
return(this);
}
/**
Adds an Element to the element.
@param hashcode name of element for hash table
@param element Adds an Element to the element.
*/
public pre addElement(String hashcode,String element)
{
addElementToRegistry(hashcode,element);
return(this);
}
/**
Adds an Element to the element.
@param element Adds an Element to the element.
*/
public pre addElement(Element element)
{
addElementToRegistry(element);
return(this);
}
/**
Adds an Element to the element.
@param element Adds an Element to the element.
*/
public pre addElement(String element)
{
addElementToRegistry(element);
return(this);
}
/**
Removes an Element from the element.
@param hashcode the name of the element to be removed.
*/
public pre removeElement(String hashcode)
{
removeElementFromRegistry(hashcode);
return(this);
}
/**
The onclick event occurs when the pointing device button is clicked
over an element. This attribute may be used with most elements.
@param The script
*/
public void setOnClick(String script)
{
addAttribute ( "onclick", script );
}
/**
The ondblclick event occurs when the pointing device button is double
clicked over an element. This attribute may be used with most elements.
@param The script
*/
public void setOnDblClick(String script)
{
addAttribute ( "ondblclick", script );
}
/**
The onmousedown event occurs when the pointing device button is pressed
over an element. This attribute may be used with most elements.
@param The script
*/
public void setOnMouseDown(String script)
{
addAttribute ( "onmousedown", script );
}
/**
The onmouseup event occurs when the pointing device button is released
over an element. This attribute may be used with most elements.
@param The script
*/
public void setOnMouseUp(String script)
{
addAttribute ( "onmouseup", script );
}
/**
The onmouseover event occurs when the pointing device is moved onto an
element. This attribute may be used with most elements.
@param The script
*/
public void setOnMouseOver(String script)
{
addAttribute ( "onmouseover", script );
}
/**
The onmousemove event occurs when the pointing device is moved while it
is over an element. This attribute may be used with most elements.
@param The script
*/
public void setOnMouseMove(String script)
{
addAttribute ( "onmousemove", script );
}
/**
The onmouseout event occurs when the pointing device is moved away from
an element. This attribute may be used with most elements.
@param The script
*/
public void setOnMouseOut(String script)
{
addAttribute ( "onmouseout", script );
}
/**
The onkeypress event occurs when a key is pressed and released over an
element. This attribute may be used with most elements.
@param The script
*/
public void setOnKeyPress(String script)
{
addAttribute ( "onkeypress", script );
}
/**
The onkeydown event occurs when a key is pressed down over an element.
This attribute may be used with most elements.
@param The script
*/
public void setOnKeyDown(String script)
{
addAttribute ( "onkeydown", script );
}
/**
The onkeyup event occurs when a key is released over an element. This
attribute may be used with most elements.
@param The script
*/
public void setOnKeyUp(String script)
{
addAttribute ( "onkeyup", script );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy