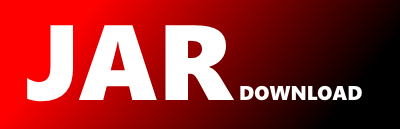
org.adempiere.legacy.apache.ecs.xml.XMLDocument Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tools Show documentation
Show all versions of tools Show documentation
A tool used for all ADempiere project ans first base library.
/******************************************************************************
* Product: Adempiere ERP & CRM Smart Business Solution *
* Copyright (C) 1999-2006 ComPiere, Inc. All Rights Reserved. *
* This program is free software; you can redistribute it and/or modify it *
* under the terms version 2 of the GNU General Public License as published *
* by the Free Software Foundation. This program is distributed in the hope *
* that it will be useful, but WITHOUT ANY WARRANTY; without even the implied *
* warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. *
* See the GNU General Public License for more details. *
* You should have received a copy of the GNU General Public License along *
* with this program; if not, write to the Free Software Foundation, Inc., *
* 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA. *
* For the text or an alternative of this public license, you may reach us *
* ComPiere, Inc., 2620 Augustine Dr. #245, Santa Clara, CA 95054, USA *
* or via [email protected] or http://www.compiere.org/license.html *
*****************************************************************************/
package org.adempiere.legacy.apache.ecs.xml;
import java.io.OutputStream;
import java.io.PrintWriter;
import java.io.Serializable;
import java.util.Vector;
import org.adempiere.legacy.apache.ecs.ConcreteElement;
/**
* XMLDocument This is the container for XML elements that can be used similar
* to org.apache.ecs.Document. However, it correctly handles XML elements and
* doesn't have any notion of a head, body, etc., that is associated with HTML
* documents.
*
* @author Brett McLaughlin
*/
public class XMLDocument
implements Serializable, Cloneable
{
/**
*
*/
private static final long serialVersionUID = 2777290564966686983L;
/** Default Version */
private static final float DEFAULT_XML_VERSION = 1.0f;
/** Version Declaration - FIXME!! */
private String versionDecl;
/** Prolog */
private Vector
type.
*
* @param href =
* String reference to stylesheet
*/
public XMLDocument addStylesheet (String href)
{
return addStylesheet (href, "text/xsl");
}
/**
* This adds the specified element to the prolog of the document
*
* @param element -
* Element to add
*/
public XMLDocument addToProlog (ConcreteElement element)
{
prolog.addElement (element);
return (this);
}
/**
* This adds an element to the XML document. If the document is empty, it
* sets the passed in element as the root element.
*
* @param element -
* XML Element to add
* @return XMLDocument - modified document
*/
public XMLDocument addElement (XML element)
{
if (content == null)
content = element;
else
content.addElement (element);
return (this);
}
/**
* Write the document to the OutputStream
*
* @param out -
* OutputStream to write to
*/
public void output (OutputStream out)
{
/**
* FIXME: The other part of the version hack! Add the version
* declaration to the beginning of the document.
*/
try
{
out.write (versionDecl.getBytes ());
}
catch (Exception e)
{
}
for (int i = 0; i < prolog.size (); i++)
{
ConcreteElement e = (ConcreteElement)prolog.elementAt (i);
e.output (out);
}
if (content != null)
content.output (out);
}
/**
* Write the document to the PrinteWriter
*
* @param out -
* PrintWriter to write to
*/
public void output (PrintWriter out)
{
/**
* FIXME: The other part of the version hack! Add the version
* declaration to the beginning of the document.
*/
out.write (versionDecl);
for (int i = 0; i < prolog.size (); i++)
{
ConcreteElement e = (ConcreteElement)prolog.elementAt (i);
e.output (out);
}
if (content != null)
content.output (out);
}
/**
* Override toString so it does something useful
*
* @return String - representation of the document
*/
public final String toString ()
{
StringBuffer retVal = new StringBuffer ();
if (codeset != null)
{
for (int i = 0; i < prolog.size (); i++)
{
ConcreteElement e = (ConcreteElement)prolog.elementAt (i);
retVal.append (e.toString (getCodeset ()) + "\n");
}
if (content != null)
retVal.append (content.toString (getCodeset ()));
}
else
{
for (int i = 0; i < prolog.size (); i++)
{
ConcreteElement e = (ConcreteElement)prolog.elementAt (i);
retVal.append (e.toString () + "\n");
}
if (content != null)
retVal.append (content.toString ());
}
/**
* FIXME: The other part of the version hack! Add the version
* declaration to the beginning of the document.
*/
return versionDecl + retVal.toString ();
}
/**
* Override toString so it prints something useful
*
* @param codeset -
* String codeset to use
* @return String - representation of the document
*/
public final String toString (String codeset)
{
StringBuffer retVal = new StringBuffer ();
for (int i = 0; i < prolog.size (); i++)
{
ConcreteElement e = (ConcreteElement)prolog.elementAt (i);
retVal.append (e.toString (getCodeset ()) + "\n");
}
if (content != null)
retVal.append (content.toString (getCodeset ()) + "\n");
/**
* FIXME: The other part of the version hack! Add the version
* declaration to the beginning of the document.
*/
return versionDecl + retVal.toString ();
}
/**
* Clone this document
*
* @return Object - cloned XMLDocument
*/
public Object clone ()
{
return content.clone ();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy