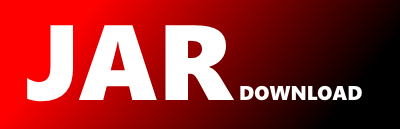
com.espertech.esper.rowregex.RegexPartitionState Maven / Gradle / Ivy
package com.espertech.esper.rowregex;
import com.espertech.esper.client.EventBean;
import com.espertech.esper.collection.MultiKeyUntyped;
import java.util.ArrayList;
import java.util.List;
/**
* All current state holding partial NFA matches.
*/
public class RegexPartitionState
{
private RegexPartitionStateRandomAccessImpl randomAccess;
private List currentStates = new ArrayList();
private MultiKeyUntyped optionalKeys;
private List intervalCallbackItems;
private boolean isCallbackScheduled;
/**
* Ctor.
* @param randomAccess for handling "prev" functions, if any
* @param optionalKeys keys for "partition", if any
* @param hasInterval true if an interval is provided
*/
public RegexPartitionState(RegexPartitionStateRandomAccessImpl randomAccess, MultiKeyUntyped optionalKeys, boolean hasInterval)
{
this.randomAccess = randomAccess;
this.optionalKeys = optionalKeys;
if (hasInterval)
{
intervalCallbackItems = new ArrayList();
}
}
/**
* Ctor.
* @param getter for "prev" access
* @param currentStates existing state
* @param hasInterval true for interval
*/
public RegexPartitionState(RegexPartitionStateRandomAccessGetter getter,
List currentStates,
boolean hasInterval) {
this(getter, currentStates, null, hasInterval);
}
/**
* Ctor.
* @param getter for "prev" access
* @param currentStates existing state
* @param optionalKeys partition keys if any
* @param hasInterval true for interval
*/
public RegexPartitionState(RegexPartitionStateRandomAccessGetter getter,
List currentStates,
MultiKeyUntyped optionalKeys,
boolean hasInterval) {
if (getter != null)
{
randomAccess = new RegexPartitionStateRandomAccessImpl(getter);
}
this.currentStates = currentStates;
this.optionalKeys = optionalKeys;
if (hasInterval)
{
intervalCallbackItems = new ArrayList();
}
}
/**
* Returns the random access for "prev".
* @return access
*/
public RegexPartitionStateRandomAccessImpl getRandomAccess() {
return randomAccess;
}
/**
* Returns partial matches.
* @return state
*/
public List getCurrentStates() {
return currentStates;
}
/**
* Sets partial matches.
* @param currentStates state to set
*/
public void setCurrentStates(List currentStates) {
this.currentStates = currentStates;
}
/**
* Returns partition keys, if any.
* @return keys
*/
public MultiKeyUntyped getOptionalKeys() {
return optionalKeys;
}
/**
* Remove an event from random access for "prev".
* @param oldEvents to remove
*/
public void removeEventFromPrev(EventBean[] oldEvents)
{
if (randomAccess != null)
{
randomAccess.remove(oldEvents);
}
}
/**
* Remove an event from random access for "prev".
* @param oldEvent to remove
*/
public void removeEventFromPrev(EventBean oldEvent)
{
if (randomAccess != null)
{
randomAccess.remove(oldEvent);
}
}
/**
* Remove an event from state.
* @param oldEvent to remove
* @return true for removed, false for not found
*/
public boolean removeEventFromState(EventBean oldEvent)
{
List keepList = new ArrayList();
for (RegexNFAStateEntry entry : currentStates)
{
boolean keep = true;
EventBean[] state = entry.getEventsPerStream();
for (EventBean aState : state)
{
if (aState == oldEvent)
{
keep = false;
break;
}
}
if (keep)
{
MultimatchState[] multimatch = entry.getOptionalMultiMatches();
if (multimatch != null)
{
for (MultimatchState aMultimatch : multimatch)
{
if ((aMultimatch != null) && (aMultimatch.containsEvent(oldEvent)))
{
keep = false;
break;
}
}
}
}
if (keep)
{
keepList.add(entry);
}
}
if (randomAccess != null)
{
randomAccess.remove(oldEvent);
}
currentStates = keepList;
return keepList.isEmpty();
}
/**
* Returns the interval states, if any.
* @return interval states
*/
public List getCallbackItems()
{
return intervalCallbackItems;
}
/**
* Returns indicator if callback is schedule.
* @return scheduled indicator
*/
public boolean isCallbackScheduled()
{
return isCallbackScheduled;
}
/**
* Returns indicator if callback is schedule.
* @param callbackScheduled true if scheduled
*/
public void setCallbackScheduled(boolean callbackScheduled)
{
isCallbackScheduled = callbackScheduled;
}
/**
* Add a callback item for intervals.
* @param endState to add
*/
public void addCallbackItem(RegexNFAStateEntry endState)
{
intervalCallbackItems.add(endState);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy