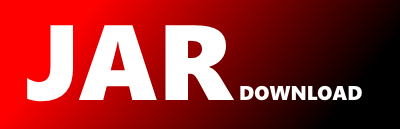
com.espertech.esper.epl.join.exec.composite.CompositeIndexQueryFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of esper Show documentation
Show all versions of esper Show documentation
Complex event processing and event series analysis component
/*
***************************************************************************************
* Copyright (C) 2006 EsperTech, Inc. All rights reserved. *
* http://www.espertech.com/esper *
* http://www.espertech.com *
* ---------------------------------------------------------------------------------- *
* The software in this package is published under the terms of the GPL license *
* a copy of which has been included with this distribution in the license.txt file. *
***************************************************************************************
*/
package com.espertech.esper.epl.join.exec.composite;
import com.espertech.esper.epl.expression.core.ExprNodeUtility;
import com.espertech.esper.epl.join.plan.QueryGraphValueEntryHashKeyed;
import com.espertech.esper.epl.join.plan.QueryGraphValueEntryRange;
import com.espertech.esper.epl.lookup.SubordPropHashKey;
import com.espertech.esper.epl.lookup.SubordPropRangeKey;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
public class CompositeIndexQueryFactory {
public static CompositeIndexQuery makeSubordinate(boolean isNWOnTrigger, int numOuterStreams, Collection keyExpr, Class[] coercionKeyTypes, Collection rangeProps, Class[] rangeCoercionTypes, List expressionTexts) {
// construct chain
List queries = new ArrayList();
if (keyExpr.size() > 0) {
List hashKeys = new ArrayList();
for (SubordPropHashKey keyExp : keyExpr) {
expressionTexts.add(ExprNodeUtility.toExpressionStringMinPrecedenceSafe(keyExp.getHashKey().getKeyExpr()));
hashKeys.add(keyExp.getHashKey());
}
queries.add(new CompositeIndexQueryKeyed(isNWOnTrigger, -1, numOuterStreams, hashKeys, coercionKeyTypes));
}
int count = 0;
for (SubordPropRangeKey rangeProp : rangeProps) {
Class coercionType = rangeCoercionTypes == null ? null : rangeCoercionTypes[count];
queries.add(new CompositeIndexQueryRange(isNWOnTrigger, -1, numOuterStreams, rangeProp, coercionType, expressionTexts));
count++;
}
// Hook up as chain for remove
CompositeIndexQuery last = null;
for (CompositeIndexQuery action : queries) {
if (last != null) {
last.setNext(action);
}
last = action;
}
return queries.get(0);
}
public static CompositeIndexQuery makeJoinSingleLookupStream(boolean isNWOnTrigger, int lookupStream, List hashKeys, Class[] keyCoercionTypes, List rangeProps, Class[] rangeCoercionTypes) {
// construct chain
List queries = new ArrayList();
if (hashKeys.size() > 0) {
queries.add(new CompositeIndexQueryKeyed(false, lookupStream, -1, hashKeys, keyCoercionTypes));
}
int count = 0;
for (QueryGraphValueEntryRange rangeProp : rangeProps) {
Class coercionType = rangeCoercionTypes == null ? null : rangeCoercionTypes[count];
SubordPropRangeKey rkey = new SubordPropRangeKey(rangeProp, coercionType);
queries.add(new CompositeIndexQueryRange(isNWOnTrigger, lookupStream, -1, rkey, coercionType, new ArrayList()));
count++;
}
// Hook up as chain for remove
CompositeIndexQuery last = null;
for (CompositeIndexQuery action : queries) {
if (last != null) {
last.setNext(action);
}
last = action;
}
return queries.get(0);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy