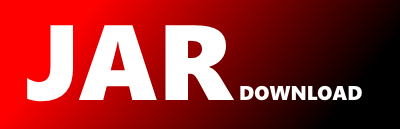
com.etsy.statsd.profiler.server.RequestHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of statsd-jvm-profiler Show documentation
Show all versions of statsd-jvm-profiler Show documentation
Simple JVM profiler using StatsD
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy