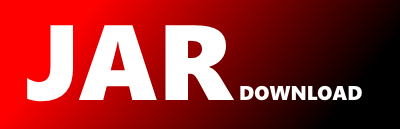
src.main.java.com.eva.log.LogFormatter Maven / Gradle / Ivy
/*
* $Id: LogFormatter.java 109 2007-03-24 14:55:03Z max $
*
* Copyright (c) 2007 Maximilian Antoni. All rights reserved.
*
* This software is licensed as described in the file LICENSE.txt, which you
* should have received as part of this distribution. The terms are also
* available at http://www.maxantoni.de/projects/eva-properties/license.txt.
*/
package com.eva.log;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.text.DateFormat;
import java.util.Date;
import java.util.logging.Formatter;
import java.util.logging.LogRecord;
/**
* default formatter for log messages.
*
* @author Max Antoni
* @version $Revision: 109 $
*/
public final class LogFormatter extends Formatter {
private static DateFormat DATE_FORMAT = DateFormat.getDateTimeInstance(
DateFormat.MEDIUM, DateFormat.MEDIUM);
private static final String COLON = ": ";
private static final String NEWLINE = "\n";
private String prefix;
/**
* creates a log formatter without a prefix.
*/
public LogFormatter() {
super();
}
/**
* creates a log formatter with the given prefix. The prefix will be printed
* in front of every message, after the log level.
*
* @param inPrefix the prefix.
*/
public LogFormatter(String inPrefix) {
super();
prefix = inPrefix;
}
/*
* @see java.util.logging.Formatter#format(java.util.logging.LogRecord)
*/
public String format(LogRecord inLogRecord) {
StringBuffer buffer = new StringBuffer();
buffer.append(DATE_FORMAT.format(new Date(inLogRecord.getMillis())));
buffer.append(' ');
buffer.append(inLogRecord.getLevel().getLocalizedName());
if(inLogRecord.getMessage() == null) {
buffer.append(COLON);
buffer.append(inLogRecord.getSourceClassName());
buffer.append('#');
buffer.append(inLogRecord.getSourceMethodName());
}
else {
if(prefix != null) {
buffer.append(' ');
buffer.append(prefix);
}
buffer.append(COLON);
buffer.append(formatMessage(inLogRecord));
}
buffer.append(NEWLINE);
Throwable throwable = inLogRecord.getThrown();
if(throwable != null) {
StringWriter aStringWriter = new StringWriter();
throwable.printStackTrace(new PrintWriter(aStringWriter));
buffer.append(aStringWriter.getBuffer());
buffer.append(NEWLINE);
}
return buffer.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy