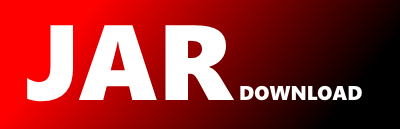
src.main.java.com.eva.properties.Replacer Maven / Gradle / Ivy
/*
* $Id: Replacer.java 109 2007-03-24 14:55:03Z max $
*
* Copyright (c) 2006-2007 Maximilian Antoni. All rights reserved.
*
* This software is licensed as described in the file LICENSE.txt, which you
* should have received as part of this distribution. The terms are also
* available at http://www.maxantoni.de/projects/eva-properties/license.txt.
*/
package com.eva.properties;
import java.io.File;
import java.io.IOException;
import java.net.URL;
import java.util.logging.Level;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/**
* helper object for replacing string values.
*
* @author Max Antoni
* @version $Revision: 109 $
*/
class Replacer {
/**
* ${something.like.this}
*/
private static final Pattern PLACEHOLDER = Pattern.compile(
"\\$\\{[a-zA-Z0-9_\\-\\.]+\\}");
/**
* Never instantiated.
*/
private Replacer() {
// prevent instantiation
}
/**
* replaces the given string value.
*
* @param inValue the string value.
* @param inContext the context.
* @return the object.
* @throws PropertiesException if the string cannot be replaced
* successfully.
*/
static String replace(String inValue, Context inContext)
throws PropertiesException {
Matcher matcher = PLACEHOLDER.matcher(inValue);
if(!matcher.find()) {
return inValue;
}
StringBuffer buffer = new StringBuffer();
int pos = 0;
do {
int s = matcher.start();
if(s > pos) {
buffer.append(inValue.substring(pos, s));
}
String group = matcher.group();
String placeholder = group.substring(2, group.length() - 1);
Object value = inContext.lookup(placeholder);
if(value == null) {
if(Log.instance().isLoggable(Level.FINE)) {
StringBuffer message = new StringBuffer();
message.append(group);
message.append(" is null");
if(inContext.isDebug()) {
message.append(" (");
inContext.writePath(message);
message.append(placeholder);
message.append(")");
}
message.append(".");
Log.instance().log(Level.FINE, message.toString());
}
return null;
}
if(value instanceof File) {
try {
buffer.append(((File) value).getCanonicalPath());
}
catch(IOException e) {
// Use absolute path then:
buffer.append(((File) value).getAbsolutePath());
}
}
else if(value instanceof URL) {
buffer.append(((URL) value).getFile());
}
else {
buffer.append(value);
}
pos = matcher.end();
}
while(matcher.find());
if(pos < inValue.length()) {
buffer.append(inValue.substring(pos));
}
return buffer.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy