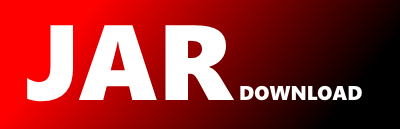
src.main.java.com.eva.properties.Switch Maven / Gradle / Ivy
/*
* $Id: Switch.java 109 2007-03-24 14:55:03Z max $
*
* Copyright (c) 2006-2007 Maximilian Antoni. All rights reserved.
*
* This software is licensed as described in the file LICENSE.txt, which you
* should have received as part of this distribution. The terms are also
* available at http://www.maxantoni.de/projects/eva-properties/license.txt.
*/
package com.eva.properties;
import java.io.File;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.logging.Level;
/**
* wrapps a list of alternatives. If the first alternative evaluates to
* null
, throws an exception or is a file that doesn't exist,
* the next alternative is tried. If no alternative is found, null
* is returned.
*
* @author Max Antoni
* @version $Revision: 109 $
*/
public class Switch implements Replaceable {
private Collection alternatives;
/**
* creates an empty switch.
*/
public Switch() {
super();
alternatives = new ArrayList();
}
/**
* creates a switch with the given alternatives.
*
* @param inAlternatives the alternatives.
*/
public Switch(Collection inAlternatives) {
super();
alternatives = inAlternatives;
}
/**
* adds the given alternative to this switch.
*
* @param inAlternative the alternative.
*/
public void add(Object inAlternative) {
alternatives.add(inAlternative);
}
/*
* @see java.lang.Object#toString()
*/
public String toString() {
Writer writer = new Writer();
write(writer);
return writer.toString();
}
/*
* @see com.eva.properties.Replaceable#write(com.eva.properties.Writer)
*/
public void write(Writer inoutWriter) {
inoutWriter.append("(\n");
inoutWriter.increaseIndentation();
for(Iterator i = alternatives.iterator(); i.hasNext();) {
inoutWriter.write(i.next());
}
inoutWriter.decreaseIndentation();
inoutWriter.appendIndentation();
inoutWriter.append(")\n");
}
/*
* @see com.eva.properties.Replaceable#replace(com.eva.properties.Context)
*/
public Object replace(Context inContext) throws PropertiesException {
for(Iterator i = alternatives.iterator(); i.hasNext();) {
Object value;
try {
value = inContext.replace(i.next());
}
catch(PropertiesException e) {
if(Log.instance().isLoggable(Level.FINE)) {
Log.instance().fine(e.getMessage());
}
continue;
}
if(value == null) {
continue;
}
if(value instanceof File && !((File) value).exists()) {
if(Log.instance().isLoggable(Level.FINE)) {
Log.instance().fine("Properties: "
+ ((File) value).getAbsolutePath()
+ " does not exist.");
}
continue;
}
if(value == Null.INSTANCE) {
continue;
}
if(value == NoProperties.INSTANCE) {
continue;
}
return value;
}
return null;
}
/*
* @see com.eva.properties.Replaceable#copy(com.eva.properties.Properties)
*/
public Replaceable copy(Properties inParent) {
Collection newAlternatives = new ArrayList();
for(Iterator i = alternatives.iterator(); i.hasNext();) {
Object value = i.next();
if(value instanceof Properties) {
newAlternatives.add(((Properties) value).copy(inParent));
}
else if(value instanceof Replaceable) {
newAlternatives.add(((Replaceable) value).copy(inParent));
}
else {
newAlternatives.add(value);
}
}
return new Switch(newAlternatives);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy