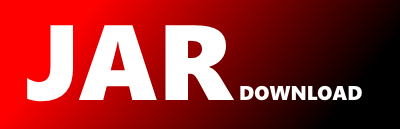
src.test.java.com.eva.properties.FactoryTest Maven / Gradle / Ivy
/*
* $Id: FactoryTest.java 109 2007-03-24 14:55:03Z max $
*
* Copyright (c) 2006-2007 Maximilian Antoni. All rights reserved.
*
* This software is licensed as described in the file LICENSE.txt, which you
* should have received as part of this distribution. The terms are also
* available at http://www.maxantoni.de/projects/eva-properties/license.txt.
*/
package com.eva.properties;
import java.io.IOException;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import junit.framework.TestCase;
/**
* @author Max Antoni
* @version $Revision: 109 $
*/
public class FactoryTest extends TestCase {
public static class InnerTestClass {
String check;
public InnerTestClass() {
check = "empty";
}
public InnerTestClass(List inList) {
assertNotNull(inList);
check = "list";
}
public InnerTestClass(Map inMap) {
assertNotNull(inMap);
check = "map";
}
public InnerTestClass(Map inMap, List inList) {
assertNotNull(inMap);
assertNotNull(inList);
check = "map and list";
}
public InnerTestClass(char inChar) {
check = "char(" + inChar + ")";
}
public InnerTestClass(int inInt) {
check = "int(" + inInt + ")";
}
}
public static class InnerTestClassLong {
String check;
public InnerTestClassLong(long inLong) {
check = "long(" + inLong + ")";
}
}
public static class InnerTestClassFloat {
String check;
public InnerTestClassFloat(float inFloat) {
check = "float(" + inFloat + ")";
}
}
public static class InnerTestClassDouble {
String check;
public InnerTestClassDouble(double inDouble) {
check = "double(" + inDouble + ")";
}
}
private Map properties;
public FactoryTest() throws IOException {
super();
properties = new MapProperties(
"classpath://com/eva/properties/factory.eva");
}
public void testEmptyConstructor() throws PropertiesException {
Factory factory = new Factory(InnerTestClass.class.getName(),
new Object[] {});
Object product = factory.replace(new Context(new MapProperties()));
assertTrue(product instanceof InnerTestClass);
assertEquals("empty", ((InnerTestClass) product).check);
}
public void testListContructor() throws PropertiesException {
Factory factory = new Factory(InnerTestClass.class.getName(),
new Object[] {
Collections.EMPTY_LIST
});
Object product = factory.replace(new Context(new MapProperties()));
assertTrue(product instanceof InnerTestClass);
assertEquals("list", ((InnerTestClass) product).check);
}
public void testMapContructor() throws PropertiesException {
Factory factory = new Factory(InnerTestClass.class.getName(),
new Object[] {
Collections.EMPTY_MAP
});
Object product = factory.replace(new Context(new MapProperties()));
assertTrue(product instanceof InnerTestClass);
assertEquals("map", ((InnerTestClass) product).check);
}
public void testMapAndListContructor() throws PropertiesException {
Factory factory = new Factory(InnerTestClass.class.getName(),
new Object[] {
Collections.EMPTY_MAP, Collections.EMPTY_LIST
});
Object product = factory.replace(new Context(new MapProperties()));
assertTrue(product instanceof InnerTestClass);
assertEquals("map and list", ((InnerTestClass) product).check);
}
public void testEmpty() {
Object product = properties.get("empty");
assertTrue(product instanceof InnerTestClass);
assertEquals("empty", ((InnerTestClass) product).check);
}
public void testList() {
Object product = properties.get("list");
assertTrue(product instanceof InnerTestClass);
assertEquals("list", ((InnerTestClass) product).check);
}
public void testMap() {
Object product = properties.get("map");
assertTrue(product instanceof InnerTestClass);
assertEquals("map", ((InnerTestClass) product).check);
}
public void testMapAndList() {
Object product = properties.get("mapAndList");
assertTrue(product instanceof InnerTestClass);
assertEquals("map and list", ((InnerTestClass) product).check);
}
public void testChar() {
Object product = properties.get("char");
assertTrue(product instanceof InnerTestClass);
assertEquals("char(*)", ((InnerTestClass) product).check);
}
public void testInt() {
Object product = properties.get("int");
assertTrue(product instanceof InnerTestClass);
assertEquals("int(123)", ((InnerTestClass) product).check);
}
public void testLong() {
Object product = properties.get("long");
assertTrue(product instanceof InnerTestClassLong);
assertEquals("long(123)", ((InnerTestClassLong) product).check);
}
public void testFloat() {
Object product = properties.get("float");
assertTrue(product instanceof InnerTestClassFloat);
assertEquals("float(12.3)", ((InnerTestClassFloat) product).check);
}
public void testDouble() {
Object product = properties.get("double");
assertTrue(product instanceof InnerTestClassDouble);
assertEquals("double(12.3)", ((InnerTestClassDouble) product).check);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy