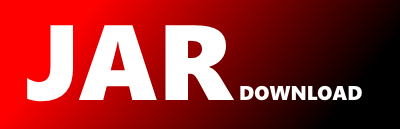
com.evasion.entity.Person Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of API Show documentation
Show all versions of API Show documentation
API de l'application modulaire evasion-en-ligne
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.evasion.entity;
import java.io.Serializable;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.Inheritance;
import javax.persistence.InheritanceType;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
/**
* Definit la structure de base d'une personne physique ou morale.
* @author sebglon
*/
@Entity
@Inheritance(strategy = InheritanceType.JOINED)
public abstract class Person implements Serializable {
/***
* serialVersionUID.
*/
private static final long serialVersionUID = 1L;
/**
* Identifiant d'une personne.
*/
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
/**
* Taille max du nom d'une personne.
*/
public static final int NOM_MAX_LENGTH = 100;
/**
* Nom de la personne.
*/
@Column(nullable = false, length = NOM_MAX_LENGTH)
private String nom;
/**
* Taille max d'une adresse mail.
*/
public static final int EMAIL_MAX_LENGTH = 320;
/**
* Email de contact de la personne.
*/
@Column(nullable = false, length = EMAIL_MAX_LENGTH)
private String email;
/**
* Constructeur par defaut.
*/
public Person() {
}
/**
* Constructeur d'une personne.
* @param name nom de la personne.
* @param mail adresse mail de la personne.
*/
public Person(final String name, final String mail) {
this.nom = name;
this.email = mail;
}
/**
* Getter de l'ID technique.
* @return renvoi d'ID technique.
*/
public Long getId() {
return id;
}
/**
* Setter de l'ID technique.
* @param id valeur de l'ID technique.
*/
public void setId(Long id) {
this.id = id;
}
/**
* Getter de email.
* @return renvoi l'adresse mail de la personne.
*/
public String getEmail() {
return email;
}
/**
* Setter de l'email.
* @param email adresse mail à setter.
*/
public void setEmail(String email) {
this.email = email;
}
/*
* Gettter de nom.
* @return nom de la personne.
*/
public String getNom() {
return nom;
}
/**
* Setter de nom.
* @param nom nom de la personne.
*/
public void setNom(String nom) {
this.nom = nom;
}
/**
* {@inheritDoc }.
*/
@Override
public boolean equals(final Object obj) {
if (obj == null) {
return false;
}
if (this == obj) {
return true;
}
if (!(obj instanceof Person)) {
return false;
}
Person rhs = (Person) obj;
return new EqualsBuilder().append(this.nom, rhs.nom).append(this.email, rhs.email).isEquals();
}
/**
* {@inheritDoc }.
*/
@Override
public int hashCode() {
return new HashCodeBuilder(17, 37).append(this.nom).append(this.email).toHashCode();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy