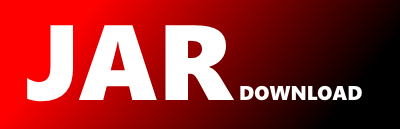
com.evasion.entity.booktravel.Itinerary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of API Show documentation
Show all versions of API Show documentation
API de l'application modulaire evasion-en-ligne
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.evasion.entity.booktravel;
import java.io.Serializable;
import java.util.Calendar;
import java.util.Date;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.Table;
import javax.persistence.Temporal;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
/**
*
* @author sebastien
*/
@Entity
@Table(name = "itinerary")
public class Itinerary implements Serializable {
/***
* serialVersionUID.
*/
private static final long serialVersionUID = 1L;
/**
* Id technique.
*/
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private int distance;
private int denivele;
private int altitudeMax;
private float pourcentagePente;
private String typeTransport;
@Temporal(javax.persistence.TemporalType.TIME)
private Calendar tempsSelle;
private float vitesseMoyenne;
/**
* Getter de l'ID technique.
* @return ID technique.
*/
public Long getId() {
return id;
}
/**
* Setter de l'ID technique.
* @param id ID technique.
*/
public void setId(Long id) {
this.id = id;
}
public int getAltitudeMax() {
return altitudeMax;
}
public void setAltitudeMax(int altitudeMax) {
this.altitudeMax = altitudeMax;
}
public int getDenivele() {
return denivele;
}
public void setDenivele(int denivele) {
this.denivele = denivele;
}
public int getDistance() {
return distance;
}
public void setDistance(int distance) {
this.distance = distance;
}
public float getPourcentagePente() {
return pourcentagePente;
}
public void setPourcentagePente(float pourcentagePente) {
this.pourcentagePente = pourcentagePente;
}
public Date getTempsSelle() {
return tempsSelle.getTime();
}
public void setTempsSelle(Date tempsSelle) {
this.tempsSelle.setTime(tempsSelle);
}
public float getVitesseMoyenne() {
return vitesseMoyenne;
}
public void setVitesseMoyenne(float vitesseMoyenne) {
this.vitesseMoyenne = vitesseMoyenne;
}
public String getTypeTransport() {
return typeTransport;
}
public void setTypeTransport(String typeTransport) {
this.typeTransport = typeTransport;
}
/**
* {@inheritDoc }.
*/
@Override
public boolean equals(final Object obj) {
if (obj == null) {
return false;
}
if (!(obj instanceof Itinerary)) {
return false;
}
final Itinerary other = (Itinerary) obj;
return new EqualsBuilder().append(this.id, other.id).isEquals();
}
/**
* {@inheritDoc }.
*/
@Override
public int hashCode() {
return new HashCodeBuilder().append(id).toHashCode();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy