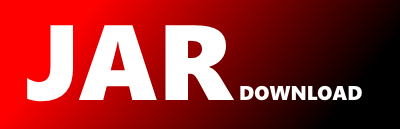
com.evasion.entity.security.User Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of API Show documentation
Show all versions of API Show documentation
API de l'application modulaire evasion-en-ligne
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.evasion.entity.security;
import java.io.Serializable;
import java.util.Calendar;
import java.util.Collection;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.Set;
import javax.persistence.CollectionTable;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.JoinColumn;
import javax.persistence.JoinTable;
import javax.persistence.NamedQuery;
import javax.persistence.PrePersist;
import javax.persistence.Table;
import javax.persistence.Temporal;
import javax.persistence.TemporalType;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
/**
*create table users(
* username varchar_ignorecase(50) not null primary key,
* password varchar_ignorecase(50) not null,
* enabled boolean not null);
*
*create table authorities (
* username varchar_ignorecase(50) not null,
* authority varchar_ignorecase(50) not null,
* constraint fk_authorities_users foreign key(username) references users(username));
* create unique index ix_auth_username on authorities (username,authority);
* @author sebastien.glon
*/
@Entity
@Table(name = "users")
@NamedQuery(name = User.FIND_ALL, query = "SELECT b FROM User b")
public class User implements Serializable {
private static final long serialVersionUID = 1L;
public static final String FIND_ALL = "findAllUsers";
@Id
@Column(nullable = false, length = 50)
private String username;
/**
* Mots de passe de l'utilisateur.
*/
@Column(nullable = false, length = 50)
private String password;
private boolean enabled;
@CollectionTable(name = "authorities")
@Column(name = "authority", nullable = false, length = 50)
private Set authorities;
@JoinTable(name = "group_members",
joinColumns =
@JoinColumn(name = "username"),
inverseJoinColumns =
@JoinColumn(name = "group_id"))
private Set groups;
/**
* Date de dernière connection.
*/
@Temporal(javax.persistence.TemporalType.TIMESTAMP)
@Column(nullable = true)
private Calendar lastLoginInternal;
/**
* Date de creation du compte utilisateur.
*/
@Temporal(TemporalType.TIMESTAMP)
private Calendar creationDateInternal;
/**
* Methode d'inititialisation de la date de creation d'un utilisateur.
* Cette methode est utilise en pre-persistance.
*/
@PrePersist
protected void initCreationDate() {
this.getCreationDateInternal().setTime(new Date());
}
protected Calendar getCreationDateInternal() {
if (creationDateInternal==null) {
creationDateInternal = new GregorianCalendar();
}
return creationDateInternal;
}
protected void setCreationDateInternal(Calendar calendar) {
this.creationDateInternal = calendar;
}
public Date getCreationDate() {
return this.getCreationDateInternal().getTime();
}
protected Calendar getLastLoginInternal() {
if (lastLoginInternal==null) {
lastLoginInternal = new GregorianCalendar();
}
return lastLoginInternal;
}
protected void setLastLoginInternal(Calendar calendar) {
this.lastLoginInternal = calendar;
}
public Date getLastLogin() {
return this.getLastLoginInternal().getTime();
}
public void setLastLogin(Date date) {
lastLoginInternal = new GregorianCalendar();
lastLoginInternal.setTime(date);
}
public Set getAuthorities() {
return authorities;
}
public void setAuthorities(Set authorities) {
this.authorities = authorities;
}
public boolean isEnabled() {
return enabled;
}
public void setEnabled(boolean enabled) {
this.enabled = enabled;
}
public Collection getGroups() {
return groups;
}
public void setGroups(Set groups) {
this.groups = groups;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
/**
* {@inheritDoc }.
*/
@Override
public boolean equals(final Object obj) {
if (obj == null) {
return false;
}
if (this == obj) {
return true;
}
if (!( obj instanceof User )) {
return false;
}
User rhs = (User) obj;
return new EqualsBuilder().append(this.username, rhs.username).isEquals();
}
/**
* {@inheritDoc }.
*/
@Override
public final int hashCode() {
return new HashCodeBuilder(17, 37).append(this.getUsername()).toHashCode();
}
/**
* {@inheritDoc }.
*/
@Override
public final String toString() {
return "com.evasion.plugin.security.User[username=" + username
+ " password=*********"
+ " lastLogin=" + lastLoginInternal
+ " enabled=" + enabled
+ " authorities=" + authorities
+ " groups=" + groups
+ "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy