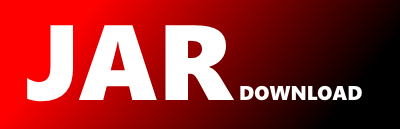
com.evasion.entity.content.Contribution Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
package com.evasion.entity.content;
import com.evasion.EntityJPA;
import com.evasion.entity.security.User;
import java.util.*;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.Lob;
import javax.persistence.ManyToOne;
import javax.persistence.OneToMany;
import javax.persistence.Table;
import javax.persistence.Temporal;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.lang.builder.ToStringBuilder;
/**
* Business Object representant une contribution de type Article.
* @author sebastien
*/
@Entity(name=Contribution.ENTITY_NAME)
@Table(name = Contribution.ENTITY_NAME)
public class Contribution extends EntityJPA {
/**
* *
* serialVersionUID.
*/
private static final long serialVersionUID = 1L;
public static final String ENTITY_NAME = "CMS_CONTRIBUTION";
/**
* Id technique.
*/
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
/**
* Titre de la contribution.
*
*/
private String title;
/**
* Texte de la contribution.
*/
@Lob
@Column(nullable = false)
private String text;
/**
* Liste de commentaire.
*/
@OneToMany(cascade = {CascadeType.PERSIST, CascadeType.REMOVE}, fetch = FetchType.LAZY)
private List commentairesInternal;
/**
* Date d'enregistrement de la contribution.
*/
@Temporal(javax.persistence.TemporalType.DATE)
private Calendar dateEnregistrementInternal;
/**
* Utilisateur rédacteur de la contribution.
*/
private String userName;
/**
* Constructeur par defaut.
*/
protected Contribution() {
}
/**
* Constructeur general de l'objet.
* @param title titre de la contribution.
* @param text corp du texte de la contribution.
* @param userName utilisateur redacteur de la contribution.
*/
public Contribution(String title, String text, String userName) {
this.title = title;
this.text = text;
this.userName = userName;
this.dateEnregistrementInternal = Calendar.getInstance();
}
/**
* Getter de l'ID technique.
* @return ID technique.
*/
public Long getId() {
return id;
}
/**
* Setter de l'ID technique.
* @param id ID technique.
*/
public void setId(Long id) {
this.id = id;
}
/**
* Getter de text.
* @return renvoi de text.
*/
public String getText() {
return text;
}
/**
* Setter de text.
* @param text text a setter.
*/
public void setText(String text) {
this.text = text;
}
/**
* Getter de titre.
* @return renvoi de titre.
*/
public String getTitle() {
return title;
}
/**
* Setter de titre.
* @param title titre a setter.
*/
public void setTitle(String title) {
this.title = title;
}
public Calendar getDateEnregistrementInternal() {
return dateEnregistrementInternal;
}
public void setDateEnregistrementInternal(Calendar dateEnregistrementInternal) {
this.dateEnregistrementInternal = dateEnregistrementInternal;
}
/**
* Getter de la date d'enregistrement de la contribution.
* @return renvoi la date.
*/
public Date getDateEnregistrement() {
if (getDateEnregistrementInternal() == null) {
setDateEnregistrementInternal(Calendar.getInstance());
}
return getDateEnregistrementInternal().getTime();
}
/**
* Setter de la date d'enregistrement.
Cette propriete n'est pas
* directement modifiable par l'utilisateur.
La valeur est initialise
* dans le constructeur de l'objet.
* @param dateEnregistrement
*/
protected void setDateEnregistrement(Date dateEnregistrement) {
Calendar cal = new GregorianCalendar();
cal.setTime(dateEnregistrement);
this.setDateEnregistrementInternal(cal);
}
/**
* Getter de l'utilisateur ayant redige la contribution.
* @return renvoi l'utilisateur.
*/
public String getUserName() {
return userName;
}
/**
* Setter de l'utilisateur ayant redige la contribution.
* @param user valeur a setter.
*/
public void setUser(String userName) {
this.userName = userName;
}
protected List getCommentairesInternal() {
return commentairesInternal;
}
protected void setCommentairesInternal(List commentairesInternal) {
this.commentairesInternal = commentairesInternal;
}
public List getCommentaires() {
return Collections.unmodifiableList(
commentairesInternal);
}
public boolean addCommentaire(Comment commentaire) {
return getCommentairesInternal().add(commentaire);
}
/**
* {@inheritDoc }
*/
@Override
public boolean equals(final Object obj) {
if (obj == null) {
return false;
}
if (this == obj) {
return true;
}
if (!(obj instanceof Contribution)) {
return false;
}
Contribution rhs = (Contribution) obj;
return new EqualsBuilder().append(this.title, rhs.title).
append(this.getDateEnregistrementInternal(), rhs.getDateEnregistrementInternal()).
isEquals();
}
/**
* @{@inheritDoc }
*/
@Override
public int hashCode() {
return new HashCodeBuilder(17, 37).append(this.title).append(this.getDateEnregistrementInternal()).toHashCode();
}
@Override
public String toString() {
return new ToStringBuilder(this).append("id", this.id).
append("title", this.title).
append("dateEnregistrement", this.getDateEnregistrement()).
toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy