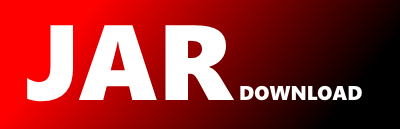
com.evasion.entity.geolocation.AdminDivision Maven / Gradle / Ivy
/*
* To change this template, choose Tools | Templates and open the template in
* the editor.
*/
package com.evasion.entity.geolocation;
import java.util.List;
import javax.persistence.*;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.lang.builder.ToStringBuilder;
/**
*
* @author sebastien.glon
*/
@Entity(name = AdminDivision.ENTITY_NAME)
@Table(name = AdminDivision.ENTITY_NAME)
@NamedQuery(name = "findByAdmCode", query = "SELECT r FROM " + AdminDivision.ENTITY_NAME + " r WHERE r."+AdminDivision.ATTR_CODE+" = ?1")
public class AdminDivision extends Location {
/**
* *
* serialVersionUID.
*/
private static final long serialVersionUID = 1L;
public static final String ENTITY_NAME = "GEO_ADM_DIVISION";
public static final String QUERY_FIND_BY_ADMCODE = "findByAdmCode";
/**
* Taille max d'un code pays.
*/
public static final int CODE_MAX_LENGTH = 2;
public static final String ATTR_CODE = "code";
/**
* Code pays normalise ISO 2.
*/
@Column(unique = true, nullable = false, length = CODE_MAX_LENGTH)
private String code;
@ManyToOne( optional = false)
private Country country;
// @TODO ajouter les accesseurs et modificateurs
@OneToMany(mappedBy="admDivision")
private List cities;
@Embedded
private Geoname geoname;
/**
* Constructeur pour la persistance.
*/
public AdminDivision() {
}
public AdminDivision(String code, Country country, Location location) {
super(location);
this.code = code;
this.country = country;
}
// @TODO implémenter la hierarchie des Division administrative
/*
* @OneToMany @JoinColumn(referencedColumnName="id",
* nullable=true,name="PARENT_ADM") private AdminDivision parent;
*
* @ManyToOne(optional=true) private List children;
*/
public String getCode() {
return code;
}
public void setCode(String code) {
this.code = code;
}
public Country getCountry() {
return country;
}
public void setCountry(Country country) {
this.country = country;
}
public Geoname getGeoname() {
return geoname;
}
public void setGeoname(Geoname geoname) {
this.geoname = geoname;
}
/**
* {@inheritDoc }
*/
@Override
public boolean equals(final Object obj) {
if (obj == null) {
return false;
}
if (this == obj) {
return true;
}
if (!(obj instanceof AdminDivision)) {
return false;
}
AdminDivision rhs = (AdminDivision) obj;
return new EqualsBuilder().append(this.code, rhs.code).
isEquals();
}
/**
* @{@inheritDoc }
*/
@Override
public int hashCode() {
return new HashCodeBuilder(17, 37).append(this.code).toHashCode();
}
/**
* @{@inheritDoc }
*/
@Override
public String toString() {
return new ToStringBuilder(this).
append("code", this.code).
append("name=", this.getName()).
append("Country", this.country).
toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy